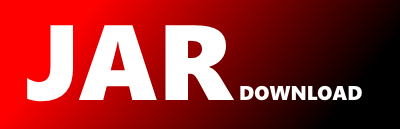
com.pulumi.aws.emr.outputs.ClusterEc2Attributes Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.emr.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ClusterEc2Attributes {
/**
* @return String containing a comma separated list of additional Amazon EC2 security group IDs for the master node.
*
*/
private @Nullable String additionalMasterSecurityGroups;
/**
* @return String containing a comma separated list of additional Amazon EC2 security group IDs for the slave nodes as a comma separated string.
*
*/
private @Nullable String additionalSlaveSecurityGroups;
/**
* @return Identifier of the Amazon EC2 EMR-Managed security group for the master node.
*
*/
private @Nullable String emrManagedMasterSecurityGroup;
/**
* @return Identifier of the Amazon EC2 EMR-Managed security group for the slave nodes.
*
*/
private @Nullable String emrManagedSlaveSecurityGroup;
/**
* @return Instance Profile for EC2 instances of the cluster assume this role.
*
*/
private String instanceProfile;
/**
* @return Amazon EC2 key pair that can be used to ssh to the master node as the user called `hadoop`.
*
*/
private @Nullable String keyName;
/**
* @return Identifier of the Amazon EC2 service-access security group - required when the cluster runs on a private subnet.
*
*/
private @Nullable String serviceAccessSecurityGroup;
/**
* @return VPC subnet id where you want the job flow to launch. Cannot specify the `cc1.4xlarge` instance type for nodes of a job flow launched in an Amazon VPC.
*
*/
private @Nullable String subnetId;
/**
* @return List of VPC subnet id-s where you want the job flow to launch. Amazon EMR identifies the best Availability Zone to launch instances according to your fleet specifications.
*
* > **NOTE on EMR-Managed security groups:** These security groups will have any missing inbound or outbound access rules added and maintained by AWS, to ensure proper communication between instances in a cluster. The EMR service will maintain these rules for groups provided in `emr_managed_master_security_group` and `emr_managed_slave_security_group`; attempts to remove the required rules may succeed, only for the EMR service to re-add them in a matter of minutes. This may cause this provider to fail to destroy an environment that contains an EMR cluster, because the EMR service does not revoke rules added on deletion, leaving a cyclic dependency between the security groups that prevents their deletion. To avoid this, use the `revoke_rules_on_delete` optional attribute for any Security Group used in `emr_managed_master_security_group` and `emr_managed_slave_security_group`. See [Amazon EMR-Managed Security Groups](http://docs.aws.amazon.com/emr/latest/ManagementGuide/emr-man-sec-groups.html) for more information about the EMR-managed security group rules.
*
*/
private @Nullable List subnetIds;
private ClusterEc2Attributes() {}
/**
* @return String containing a comma separated list of additional Amazon EC2 security group IDs for the master node.
*
*/
public Optional additionalMasterSecurityGroups() {
return Optional.ofNullable(this.additionalMasterSecurityGroups);
}
/**
* @return String containing a comma separated list of additional Amazon EC2 security group IDs for the slave nodes as a comma separated string.
*
*/
public Optional additionalSlaveSecurityGroups() {
return Optional.ofNullable(this.additionalSlaveSecurityGroups);
}
/**
* @return Identifier of the Amazon EC2 EMR-Managed security group for the master node.
*
*/
public Optional emrManagedMasterSecurityGroup() {
return Optional.ofNullable(this.emrManagedMasterSecurityGroup);
}
/**
* @return Identifier of the Amazon EC2 EMR-Managed security group for the slave nodes.
*
*/
public Optional emrManagedSlaveSecurityGroup() {
return Optional.ofNullable(this.emrManagedSlaveSecurityGroup);
}
/**
* @return Instance Profile for EC2 instances of the cluster assume this role.
*
*/
public String instanceProfile() {
return this.instanceProfile;
}
/**
* @return Amazon EC2 key pair that can be used to ssh to the master node as the user called `hadoop`.
*
*/
public Optional keyName() {
return Optional.ofNullable(this.keyName);
}
/**
* @return Identifier of the Amazon EC2 service-access security group - required when the cluster runs on a private subnet.
*
*/
public Optional serviceAccessSecurityGroup() {
return Optional.ofNullable(this.serviceAccessSecurityGroup);
}
/**
* @return VPC subnet id where you want the job flow to launch. Cannot specify the `cc1.4xlarge` instance type for nodes of a job flow launched in an Amazon VPC.
*
*/
public Optional subnetId() {
return Optional.ofNullable(this.subnetId);
}
/**
* @return List of VPC subnet id-s where you want the job flow to launch. Amazon EMR identifies the best Availability Zone to launch instances according to your fleet specifications.
*
* > **NOTE on EMR-Managed security groups:** These security groups will have any missing inbound or outbound access rules added and maintained by AWS, to ensure proper communication between instances in a cluster. The EMR service will maintain these rules for groups provided in `emr_managed_master_security_group` and `emr_managed_slave_security_group`; attempts to remove the required rules may succeed, only for the EMR service to re-add them in a matter of minutes. This may cause this provider to fail to destroy an environment that contains an EMR cluster, because the EMR service does not revoke rules added on deletion, leaving a cyclic dependency between the security groups that prevents their deletion. To avoid this, use the `revoke_rules_on_delete` optional attribute for any Security Group used in `emr_managed_master_security_group` and `emr_managed_slave_security_group`. See [Amazon EMR-Managed Security Groups](http://docs.aws.amazon.com/emr/latest/ManagementGuide/emr-man-sec-groups.html) for more information about the EMR-managed security group rules.
*
*/
public List subnetIds() {
return this.subnetIds == null ? List.of() : this.subnetIds;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ClusterEc2Attributes defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String additionalMasterSecurityGroups;
private @Nullable String additionalSlaveSecurityGroups;
private @Nullable String emrManagedMasterSecurityGroup;
private @Nullable String emrManagedSlaveSecurityGroup;
private String instanceProfile;
private @Nullable String keyName;
private @Nullable String serviceAccessSecurityGroup;
private @Nullable String subnetId;
private @Nullable List subnetIds;
public Builder() {}
public Builder(ClusterEc2Attributes defaults) {
Objects.requireNonNull(defaults);
this.additionalMasterSecurityGroups = defaults.additionalMasterSecurityGroups;
this.additionalSlaveSecurityGroups = defaults.additionalSlaveSecurityGroups;
this.emrManagedMasterSecurityGroup = defaults.emrManagedMasterSecurityGroup;
this.emrManagedSlaveSecurityGroup = defaults.emrManagedSlaveSecurityGroup;
this.instanceProfile = defaults.instanceProfile;
this.keyName = defaults.keyName;
this.serviceAccessSecurityGroup = defaults.serviceAccessSecurityGroup;
this.subnetId = defaults.subnetId;
this.subnetIds = defaults.subnetIds;
}
@CustomType.Setter
public Builder additionalMasterSecurityGroups(@Nullable String additionalMasterSecurityGroups) {
this.additionalMasterSecurityGroups = additionalMasterSecurityGroups;
return this;
}
@CustomType.Setter
public Builder additionalSlaveSecurityGroups(@Nullable String additionalSlaveSecurityGroups) {
this.additionalSlaveSecurityGroups = additionalSlaveSecurityGroups;
return this;
}
@CustomType.Setter
public Builder emrManagedMasterSecurityGroup(@Nullable String emrManagedMasterSecurityGroup) {
this.emrManagedMasterSecurityGroup = emrManagedMasterSecurityGroup;
return this;
}
@CustomType.Setter
public Builder emrManagedSlaveSecurityGroup(@Nullable String emrManagedSlaveSecurityGroup) {
this.emrManagedSlaveSecurityGroup = emrManagedSlaveSecurityGroup;
return this;
}
@CustomType.Setter
public Builder instanceProfile(String instanceProfile) {
if (instanceProfile == null) {
throw new MissingRequiredPropertyException("ClusterEc2Attributes", "instanceProfile");
}
this.instanceProfile = instanceProfile;
return this;
}
@CustomType.Setter
public Builder keyName(@Nullable String keyName) {
this.keyName = keyName;
return this;
}
@CustomType.Setter
public Builder serviceAccessSecurityGroup(@Nullable String serviceAccessSecurityGroup) {
this.serviceAccessSecurityGroup = serviceAccessSecurityGroup;
return this;
}
@CustomType.Setter
public Builder subnetId(@Nullable String subnetId) {
this.subnetId = subnetId;
return this;
}
@CustomType.Setter
public Builder subnetIds(@Nullable List subnetIds) {
this.subnetIds = subnetIds;
return this;
}
public Builder subnetIds(String... subnetIds) {
return subnetIds(List.of(subnetIds));
}
public ClusterEc2Attributes build() {
final var _resultValue = new ClusterEc2Attributes();
_resultValue.additionalMasterSecurityGroups = additionalMasterSecurityGroups;
_resultValue.additionalSlaveSecurityGroups = additionalSlaveSecurityGroups;
_resultValue.emrManagedMasterSecurityGroup = emrManagedMasterSecurityGroup;
_resultValue.emrManagedSlaveSecurityGroup = emrManagedSlaveSecurityGroup;
_resultValue.instanceProfile = instanceProfile;
_resultValue.keyName = keyName;
_resultValue.serviceAccessSecurityGroup = serviceAccessSecurityGroup;
_resultValue.subnetId = subnetId;
_resultValue.subnetIds = subnetIds;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy