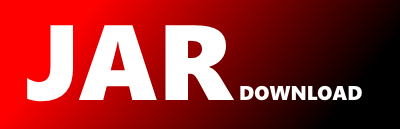
com.pulumi.aws.lambda.LayerVersion Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lambda;
import com.pulumi.asset.Archive;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.lambda.LayerVersionArgs;
import com.pulumi.aws.lambda.inputs.LayerVersionState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a Lambda Layer Version resource. Lambda Layers allow you to reuse shared bits of code across multiple lambda functions.
*
* For information about Lambda Layers and how to use them, see [AWS Lambda Layers](https://docs.aws.amazon.com/lambda/latest/dg/configuration-layers.html).
*
* > **NOTE:** Setting `skip_destroy` to `true` means that the AWS Provider will _not_ destroy any layer version, even when running destroy. Layer versions are thus intentional dangling resources that are _not_ managed by the provider and may incur extra expense in your AWS account.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.lambda.LayerVersion;
* import com.pulumi.aws.lambda.LayerVersionArgs;
* import com.pulumi.asset.FileArchive;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var lambdaLayer = new LayerVersion("lambdaLayer", LayerVersionArgs.builder()
* .code(new FileArchive("lambda_layer_payload.zip"))
* .layerName("lambda_layer_name")
* .compatibleRuntimes("nodejs20.x")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Specifying the Deployment Package
*
* AWS Lambda Layers expect source code to be provided as a deployment package whose structure varies depending on which `compatible_runtimes` this layer specifies.
* See [Runtimes](https://docs.aws.amazon.com/lambda/latest/dg/API_PublishLayerVersion.html#SSS-PublishLayerVersion-request-CompatibleRuntimes) for the valid values of `compatible_runtimes`.
*
* Once you have created your deployment package you can specify it either directly as a local file (using the `filename` argument) or
* indirectly via Amazon S3 (using the `s3_bucket`, `s3_key` and `s3_object_version` arguments). When providing the deployment
* package via S3 it may be useful to use the `aws.s3.BucketObjectv2` resource to upload it.
*
* For larger deployment packages it is recommended by Amazon to upload via S3, since the S3 API has better support for uploading large files efficiently.
*
* ## Import
*
* Using `pulumi import`, import Lambda Layers using `arn`. For example:
*
* ```sh
* $ pulumi import aws:lambda/layerVersion:LayerVersion test_layer arn:aws:lambda:_REGION_:_ACCOUNT_ID_:layer:_LAYER_NAME_:_LAYER_VERSION_
* ```
*
*/
@ResourceType(type="aws:lambda/layerVersion:LayerVersion")
public class LayerVersion extends com.pulumi.resources.CustomResource {
/**
* ARN of the Lambda Layer with version.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the Lambda Layer with version.
*
*/
public Output arn() {
return this.arn;
}
/**
* Path to the function's deployment package within the local filesystem. If defined, The `s3_`-prefixed options cannot be used.
*
*/
@Export(name="code", refs={Archive.class}, tree="[0]")
private Output* @Nullable */ Archive> code;
/**
* @return Path to the function's deployment package within the local filesystem. If defined, The `s3_`-prefixed options cannot be used.
*
*/
public Output> code() {
return Codegen.optional(this.code);
}
/**
* Base64-encoded representation of raw SHA-256 sum of the zip file.
*
*/
@Export(name="codeSha256", refs={String.class}, tree="[0]")
private Output codeSha256;
/**
* @return Base64-encoded representation of raw SHA-256 sum of the zip file.
*
*/
public Output codeSha256() {
return this.codeSha256;
}
/**
* List of [Architectures](https://docs.aws.amazon.com/lambda/latest/dg/API_PublishLayerVersion.html#SSS-PublishLayerVersion-request-CompatibleArchitectures) this layer is compatible with. Currently `x86_64` and `arm64` can be specified.
*
*/
@Export(name="compatibleArchitectures", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> compatibleArchitectures;
/**
* @return List of [Architectures](https://docs.aws.amazon.com/lambda/latest/dg/API_PublishLayerVersion.html#SSS-PublishLayerVersion-request-CompatibleArchitectures) this layer is compatible with. Currently `x86_64` and `arm64` can be specified.
*
*/
public Output>> compatibleArchitectures() {
return Codegen.optional(this.compatibleArchitectures);
}
/**
* List of [Runtimes](https://docs.aws.amazon.com/lambda/latest/dg/API_PublishLayerVersion.html#SSS-PublishLayerVersion-request-CompatibleRuntimes) this layer is compatible with. Up to 15 runtimes can be specified.
*
*/
@Export(name="compatibleRuntimes", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> compatibleRuntimes;
/**
* @return List of [Runtimes](https://docs.aws.amazon.com/lambda/latest/dg/API_PublishLayerVersion.html#SSS-PublishLayerVersion-request-CompatibleRuntimes) this layer is compatible with. Up to 15 runtimes can be specified.
*
*/
public Output>> compatibleRuntimes() {
return Codegen.optional(this.compatibleRuntimes);
}
/**
* Date this resource was created.
*
*/
@Export(name="createdDate", refs={String.class}, tree="[0]")
private Output createdDate;
/**
* @return Date this resource was created.
*
*/
public Output createdDate() {
return this.createdDate;
}
/**
* Description of what your Lambda Layer does.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description of what your Lambda Layer does.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* ARN of the Lambda Layer without version.
*
*/
@Export(name="layerArn", refs={String.class}, tree="[0]")
private Output layerArn;
/**
* @return ARN of the Lambda Layer without version.
*
*/
public Output layerArn() {
return this.layerArn;
}
/**
* Unique name for your Lambda Layer
*
* The following arguments are optional:
*
*/
@Export(name="layerName", refs={String.class}, tree="[0]")
private Output layerName;
/**
* @return Unique name for your Lambda Layer
*
* The following arguments are optional:
*
*/
public Output layerName() {
return this.layerName;
}
/**
* License info for your Lambda Layer. See [License Info](https://docs.aws.amazon.com/lambda/latest/dg/API_PublishLayerVersion.html#SSS-PublishLayerVersion-request-LicenseInfo).
*
*/
@Export(name="licenseInfo", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> licenseInfo;
/**
* @return License info for your Lambda Layer. See [License Info](https://docs.aws.amazon.com/lambda/latest/dg/API_PublishLayerVersion.html#SSS-PublishLayerVersion-request-LicenseInfo).
*
*/
public Output> licenseInfo() {
return Codegen.optional(this.licenseInfo);
}
/**
* S3 bucket location containing the function's deployment package. Conflicts with `filename`. This bucket must reside in the same AWS region where you are creating the Lambda function.
*
*/
@Export(name="s3Bucket", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> s3Bucket;
/**
* @return S3 bucket location containing the function's deployment package. Conflicts with `filename`. This bucket must reside in the same AWS region where you are creating the Lambda function.
*
*/
public Output> s3Bucket() {
return Codegen.optional(this.s3Bucket);
}
/**
* S3 key of an object containing the function's deployment package. Conflicts with `filename`.
*
*/
@Export(name="s3Key", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> s3Key;
/**
* @return S3 key of an object containing the function's deployment package. Conflicts with `filename`.
*
*/
public Output> s3Key() {
return Codegen.optional(this.s3Key);
}
/**
* Object version containing the function's deployment package. Conflicts with `filename`.
*
*/
@Export(name="s3ObjectVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> s3ObjectVersion;
/**
* @return Object version containing the function's deployment package. Conflicts with `filename`.
*
*/
public Output> s3ObjectVersion() {
return Codegen.optional(this.s3ObjectVersion);
}
/**
* ARN of a signing job.
*
*/
@Export(name="signingJobArn", refs={String.class}, tree="[0]")
private Output signingJobArn;
/**
* @return ARN of a signing job.
*
*/
public Output signingJobArn() {
return this.signingJobArn;
}
/**
* ARN for a signing profile version.
*
*/
@Export(name="signingProfileVersionArn", refs={String.class}, tree="[0]")
private Output signingProfileVersionArn;
/**
* @return ARN for a signing profile version.
*
*/
public Output signingProfileVersionArn() {
return this.signingProfileVersionArn;
}
/**
* Whether to retain the old version of a previously deployed Lambda Layer. Default is `false`. When this is not set to `true`, changing any of `compatible_architectures`, `compatible_runtimes`, `description`, `filename`, `layer_name`, `license_info`, `s3_bucket`, `s3_key`, `s3_object_version`, or `source_code_hash` forces deletion of the existing layer version and creation of a new layer version.
*
*/
@Export(name="skipDestroy", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> skipDestroy;
/**
* @return Whether to retain the old version of a previously deployed Lambda Layer. Default is `false`. When this is not set to `true`, changing any of `compatible_architectures`, `compatible_runtimes`, `description`, `filename`, `layer_name`, `license_info`, `s3_bucket`, `s3_key`, `s3_object_version`, or `source_code_hash` forces deletion of the existing layer version and creation of a new layer version.
*
*/
public Output> skipDestroy() {
return Codegen.optional(this.skipDestroy);
}
/**
* Virtual attribute used to trigger replacement when source code changes. Must be set to a base64-encoded SHA256 hash of the package file specified with either `filename` or `s3_key`.
*
*/
@Export(name="sourceCodeHash", refs={String.class}, tree="[0]")
private Output sourceCodeHash;
/**
* @return Virtual attribute used to trigger replacement when source code changes. Must be set to a base64-encoded SHA256 hash of the package file specified with either `filename` or `s3_key`.
*
*/
public Output sourceCodeHash() {
return this.sourceCodeHash;
}
/**
* Size in bytes of the function .zip file.
*
*/
@Export(name="sourceCodeSize", refs={Integer.class}, tree="[0]")
private Output sourceCodeSize;
/**
* @return Size in bytes of the function .zip file.
*
*/
public Output sourceCodeSize() {
return this.sourceCodeSize;
}
/**
* Lambda Layer version.
*
*/
@Export(name="version", refs={String.class}, tree="[0]")
private Output version;
/**
* @return Lambda Layer version.
*
*/
public Output version() {
return this.version;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public LayerVersion(java.lang.String name) {
this(name, LayerVersionArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public LayerVersion(java.lang.String name, LayerVersionArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public LayerVersion(java.lang.String name, LayerVersionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:lambda/layerVersion:LayerVersion", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private LayerVersion(java.lang.String name, Output id, @Nullable LayerVersionState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:lambda/layerVersion:LayerVersion", name, state, makeResourceOptions(options, id), false);
}
private static LayerVersionArgs makeArgs(LayerVersionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? LayerVersionArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static LayerVersion get(java.lang.String name, Output id, @Nullable LayerVersionState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new LayerVersion(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy