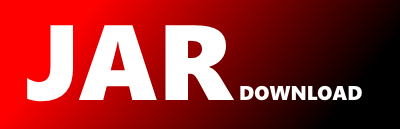
com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettings Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.sagemaker.outputs;
import com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettingsCanvasAppSettings;
import com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettingsCodeEditorAppSettings;
import com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettingsCustomFileSystemConfig;
import com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettingsCustomPosixUserConfig;
import com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettingsJupyterLabAppSettings;
import com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettingsJupyterServerAppSettings;
import com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettingsKernelGatewayAppSettings;
import com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettingsRSessionAppSettings;
import com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettingsRStudioServerProAppSettings;
import com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettingsSharingSettings;
import com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettingsSpaceStorageSettings;
import com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettingsStudioWebPortalSettings;
import com.pulumi.aws.sagemaker.outputs.DomainDefaultUserSettingsTensorBoardAppSettings;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DomainDefaultUserSettings {
/**
* @return The Canvas app settings. See `canvas_app_settings` Block below.
*
*/
private @Nullable DomainDefaultUserSettingsCanvasAppSettings canvasAppSettings;
/**
* @return The Code Editor application settings. See `code_editor_app_settings` Block below.
*
*/
private @Nullable DomainDefaultUserSettingsCodeEditorAppSettings codeEditorAppSettings;
/**
* @return The settings for assigning a custom file system to a user profile. Permitted users can access this file system in Amazon SageMaker Studio. See `custom_file_system_config` Block below.
*
*/
private @Nullable List customFileSystemConfigs;
/**
* @return Details about the POSIX identity that is used for file system operations. See `custom_posix_user_config` Block below.
*
*/
private @Nullable DomainDefaultUserSettingsCustomPosixUserConfig customPosixUserConfig;
/**
* @return The default experience that the user is directed to when accessing the domain. The supported values are: `studio::`: Indicates that Studio is the default experience. This value can only be passed if StudioWebPortal is set to ENABLED. `app:JupyterServer:`: Indicates that Studio Classic is the default experience.
*
*/
private @Nullable String defaultLandingUri;
/**
* @return The execution role ARN for the user.
*
*/
private String executionRole;
/**
* @return The settings for the JupyterLab application. See `jupyter_lab_app_settings` Block below.
*
*/
private @Nullable DomainDefaultUserSettingsJupyterLabAppSettings jupyterLabAppSettings;
/**
* @return The Jupyter server's app settings. See `jupyter_server_app_settings` Block below.
*
*/
private @Nullable DomainDefaultUserSettingsJupyterServerAppSettings jupyterServerAppSettings;
/**
* @return The kernel gateway app settings. See `kernel_gateway_app_settings` Block below.
*
*/
private @Nullable DomainDefaultUserSettingsKernelGatewayAppSettings kernelGatewayAppSettings;
/**
* @return The RSession app settings. See `r_session_app_settings` Block below.
*
*/
private @Nullable DomainDefaultUserSettingsRSessionAppSettings rSessionAppSettings;
/**
* @return A collection of settings that configure user interaction with the RStudioServerPro app. See `r_studio_server_pro_app_settings` Block below.
*
*/
private @Nullable DomainDefaultUserSettingsRStudioServerProAppSettings rStudioServerProAppSettings;
/**
* @return A list of security group IDs that will be attached to the user.
*
*/
private @Nullable List securityGroups;
/**
* @return The sharing settings. See `sharing_settings` Block below.
*
*/
private @Nullable DomainDefaultUserSettingsSharingSettings sharingSettings;
/**
* @return The storage settings for a private space. See `space_storage_settings` Block below.
*
*/
private @Nullable DomainDefaultUserSettingsSpaceStorageSettings spaceStorageSettings;
/**
* @return Whether the user can access Studio. If this value is set to `DISABLED`, the user cannot access Studio, even if that is the default experience for the domain. Valid values are `ENABLED` and `DISABLED`.
*
*/
private @Nullable String studioWebPortal;
/**
* @return The Studio Web Portal settings. See `studio_web_portal_settings` Block below.
*
*/
private @Nullable DomainDefaultUserSettingsStudioWebPortalSettings studioWebPortalSettings;
/**
* @return The TensorBoard app settings. See `tensor_board_app_settings` Block below.
*
*/
private @Nullable DomainDefaultUserSettingsTensorBoardAppSettings tensorBoardAppSettings;
private DomainDefaultUserSettings() {}
/**
* @return The Canvas app settings. See `canvas_app_settings` Block below.
*
*/
public Optional canvasAppSettings() {
return Optional.ofNullable(this.canvasAppSettings);
}
/**
* @return The Code Editor application settings. See `code_editor_app_settings` Block below.
*
*/
public Optional codeEditorAppSettings() {
return Optional.ofNullable(this.codeEditorAppSettings);
}
/**
* @return The settings for assigning a custom file system to a user profile. Permitted users can access this file system in Amazon SageMaker Studio. See `custom_file_system_config` Block below.
*
*/
public List customFileSystemConfigs() {
return this.customFileSystemConfigs == null ? List.of() : this.customFileSystemConfigs;
}
/**
* @return Details about the POSIX identity that is used for file system operations. See `custom_posix_user_config` Block below.
*
*/
public Optional customPosixUserConfig() {
return Optional.ofNullable(this.customPosixUserConfig);
}
/**
* @return The default experience that the user is directed to when accessing the domain. The supported values are: `studio::`: Indicates that Studio is the default experience. This value can only be passed if StudioWebPortal is set to ENABLED. `app:JupyterServer:`: Indicates that Studio Classic is the default experience.
*
*/
public Optional defaultLandingUri() {
return Optional.ofNullable(this.defaultLandingUri);
}
/**
* @return The execution role ARN for the user.
*
*/
public String executionRole() {
return this.executionRole;
}
/**
* @return The settings for the JupyterLab application. See `jupyter_lab_app_settings` Block below.
*
*/
public Optional jupyterLabAppSettings() {
return Optional.ofNullable(this.jupyterLabAppSettings);
}
/**
* @return The Jupyter server's app settings. See `jupyter_server_app_settings` Block below.
*
*/
public Optional jupyterServerAppSettings() {
return Optional.ofNullable(this.jupyterServerAppSettings);
}
/**
* @return The kernel gateway app settings. See `kernel_gateway_app_settings` Block below.
*
*/
public Optional kernelGatewayAppSettings() {
return Optional.ofNullable(this.kernelGatewayAppSettings);
}
/**
* @return The RSession app settings. See `r_session_app_settings` Block below.
*
*/
public Optional rSessionAppSettings() {
return Optional.ofNullable(this.rSessionAppSettings);
}
/**
* @return A collection of settings that configure user interaction with the RStudioServerPro app. See `r_studio_server_pro_app_settings` Block below.
*
*/
public Optional rStudioServerProAppSettings() {
return Optional.ofNullable(this.rStudioServerProAppSettings);
}
/**
* @return A list of security group IDs that will be attached to the user.
*
*/
public List securityGroups() {
return this.securityGroups == null ? List.of() : this.securityGroups;
}
/**
* @return The sharing settings. See `sharing_settings` Block below.
*
*/
public Optional sharingSettings() {
return Optional.ofNullable(this.sharingSettings);
}
/**
* @return The storage settings for a private space. See `space_storage_settings` Block below.
*
*/
public Optional spaceStorageSettings() {
return Optional.ofNullable(this.spaceStorageSettings);
}
/**
* @return Whether the user can access Studio. If this value is set to `DISABLED`, the user cannot access Studio, even if that is the default experience for the domain. Valid values are `ENABLED` and `DISABLED`.
*
*/
public Optional studioWebPortal() {
return Optional.ofNullable(this.studioWebPortal);
}
/**
* @return The Studio Web Portal settings. See `studio_web_portal_settings` Block below.
*
*/
public Optional studioWebPortalSettings() {
return Optional.ofNullable(this.studioWebPortalSettings);
}
/**
* @return The TensorBoard app settings. See `tensor_board_app_settings` Block below.
*
*/
public Optional tensorBoardAppSettings() {
return Optional.ofNullable(this.tensorBoardAppSettings);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DomainDefaultUserSettings defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable DomainDefaultUserSettingsCanvasAppSettings canvasAppSettings;
private @Nullable DomainDefaultUserSettingsCodeEditorAppSettings codeEditorAppSettings;
private @Nullable List customFileSystemConfigs;
private @Nullable DomainDefaultUserSettingsCustomPosixUserConfig customPosixUserConfig;
private @Nullable String defaultLandingUri;
private String executionRole;
private @Nullable DomainDefaultUserSettingsJupyterLabAppSettings jupyterLabAppSettings;
private @Nullable DomainDefaultUserSettingsJupyterServerAppSettings jupyterServerAppSettings;
private @Nullable DomainDefaultUserSettingsKernelGatewayAppSettings kernelGatewayAppSettings;
private @Nullable DomainDefaultUserSettingsRSessionAppSettings rSessionAppSettings;
private @Nullable DomainDefaultUserSettingsRStudioServerProAppSettings rStudioServerProAppSettings;
private @Nullable List securityGroups;
private @Nullable DomainDefaultUserSettingsSharingSettings sharingSettings;
private @Nullable DomainDefaultUserSettingsSpaceStorageSettings spaceStorageSettings;
private @Nullable String studioWebPortal;
private @Nullable DomainDefaultUserSettingsStudioWebPortalSettings studioWebPortalSettings;
private @Nullable DomainDefaultUserSettingsTensorBoardAppSettings tensorBoardAppSettings;
public Builder() {}
public Builder(DomainDefaultUserSettings defaults) {
Objects.requireNonNull(defaults);
this.canvasAppSettings = defaults.canvasAppSettings;
this.codeEditorAppSettings = defaults.codeEditorAppSettings;
this.customFileSystemConfigs = defaults.customFileSystemConfigs;
this.customPosixUserConfig = defaults.customPosixUserConfig;
this.defaultLandingUri = defaults.defaultLandingUri;
this.executionRole = defaults.executionRole;
this.jupyterLabAppSettings = defaults.jupyterLabAppSettings;
this.jupyterServerAppSettings = defaults.jupyterServerAppSettings;
this.kernelGatewayAppSettings = defaults.kernelGatewayAppSettings;
this.rSessionAppSettings = defaults.rSessionAppSettings;
this.rStudioServerProAppSettings = defaults.rStudioServerProAppSettings;
this.securityGroups = defaults.securityGroups;
this.sharingSettings = defaults.sharingSettings;
this.spaceStorageSettings = defaults.spaceStorageSettings;
this.studioWebPortal = defaults.studioWebPortal;
this.studioWebPortalSettings = defaults.studioWebPortalSettings;
this.tensorBoardAppSettings = defaults.tensorBoardAppSettings;
}
@CustomType.Setter
public Builder canvasAppSettings(@Nullable DomainDefaultUserSettingsCanvasAppSettings canvasAppSettings) {
this.canvasAppSettings = canvasAppSettings;
return this;
}
@CustomType.Setter
public Builder codeEditorAppSettings(@Nullable DomainDefaultUserSettingsCodeEditorAppSettings codeEditorAppSettings) {
this.codeEditorAppSettings = codeEditorAppSettings;
return this;
}
@CustomType.Setter
public Builder customFileSystemConfigs(@Nullable List customFileSystemConfigs) {
this.customFileSystemConfigs = customFileSystemConfigs;
return this;
}
public Builder customFileSystemConfigs(DomainDefaultUserSettingsCustomFileSystemConfig... customFileSystemConfigs) {
return customFileSystemConfigs(List.of(customFileSystemConfigs));
}
@CustomType.Setter
public Builder customPosixUserConfig(@Nullable DomainDefaultUserSettingsCustomPosixUserConfig customPosixUserConfig) {
this.customPosixUserConfig = customPosixUserConfig;
return this;
}
@CustomType.Setter
public Builder defaultLandingUri(@Nullable String defaultLandingUri) {
this.defaultLandingUri = defaultLandingUri;
return this;
}
@CustomType.Setter
public Builder executionRole(String executionRole) {
if (executionRole == null) {
throw new MissingRequiredPropertyException("DomainDefaultUserSettings", "executionRole");
}
this.executionRole = executionRole;
return this;
}
@CustomType.Setter
public Builder jupyterLabAppSettings(@Nullable DomainDefaultUserSettingsJupyterLabAppSettings jupyterLabAppSettings) {
this.jupyterLabAppSettings = jupyterLabAppSettings;
return this;
}
@CustomType.Setter
public Builder jupyterServerAppSettings(@Nullable DomainDefaultUserSettingsJupyterServerAppSettings jupyterServerAppSettings) {
this.jupyterServerAppSettings = jupyterServerAppSettings;
return this;
}
@CustomType.Setter
public Builder kernelGatewayAppSettings(@Nullable DomainDefaultUserSettingsKernelGatewayAppSettings kernelGatewayAppSettings) {
this.kernelGatewayAppSettings = kernelGatewayAppSettings;
return this;
}
@CustomType.Setter
public Builder rSessionAppSettings(@Nullable DomainDefaultUserSettingsRSessionAppSettings rSessionAppSettings) {
this.rSessionAppSettings = rSessionAppSettings;
return this;
}
@CustomType.Setter
public Builder rStudioServerProAppSettings(@Nullable DomainDefaultUserSettingsRStudioServerProAppSettings rStudioServerProAppSettings) {
this.rStudioServerProAppSettings = rStudioServerProAppSettings;
return this;
}
@CustomType.Setter
public Builder securityGroups(@Nullable List securityGroups) {
this.securityGroups = securityGroups;
return this;
}
public Builder securityGroups(String... securityGroups) {
return securityGroups(List.of(securityGroups));
}
@CustomType.Setter
public Builder sharingSettings(@Nullable DomainDefaultUserSettingsSharingSettings sharingSettings) {
this.sharingSettings = sharingSettings;
return this;
}
@CustomType.Setter
public Builder spaceStorageSettings(@Nullable DomainDefaultUserSettingsSpaceStorageSettings spaceStorageSettings) {
this.spaceStorageSettings = spaceStorageSettings;
return this;
}
@CustomType.Setter
public Builder studioWebPortal(@Nullable String studioWebPortal) {
this.studioWebPortal = studioWebPortal;
return this;
}
@CustomType.Setter
public Builder studioWebPortalSettings(@Nullable DomainDefaultUserSettingsStudioWebPortalSettings studioWebPortalSettings) {
this.studioWebPortalSettings = studioWebPortalSettings;
return this;
}
@CustomType.Setter
public Builder tensorBoardAppSettings(@Nullable DomainDefaultUserSettingsTensorBoardAppSettings tensorBoardAppSettings) {
this.tensorBoardAppSettings = tensorBoardAppSettings;
return this;
}
public DomainDefaultUserSettings build() {
final var _resultValue = new DomainDefaultUserSettings();
_resultValue.canvasAppSettings = canvasAppSettings;
_resultValue.codeEditorAppSettings = codeEditorAppSettings;
_resultValue.customFileSystemConfigs = customFileSystemConfigs;
_resultValue.customPosixUserConfig = customPosixUserConfig;
_resultValue.defaultLandingUri = defaultLandingUri;
_resultValue.executionRole = executionRole;
_resultValue.jupyterLabAppSettings = jupyterLabAppSettings;
_resultValue.jupyterServerAppSettings = jupyterServerAppSettings;
_resultValue.kernelGatewayAppSettings = kernelGatewayAppSettings;
_resultValue.rSessionAppSettings = rSessionAppSettings;
_resultValue.rStudioServerProAppSettings = rStudioServerProAppSettings;
_resultValue.securityGroups = securityGroups;
_resultValue.sharingSettings = sharingSettings;
_resultValue.spaceStorageSettings = spaceStorageSettings;
_resultValue.studioWebPortal = studioWebPortal;
_resultValue.studioWebPortalSettings = studioWebPortalSettings;
_resultValue.tensorBoardAppSettings = tensorBoardAppSettings;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy