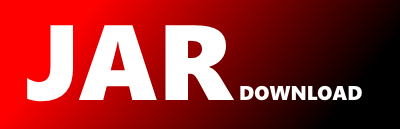
com.pulumi.aws.servicecatalog.PortfolioShareArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.servicecatalog;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PortfolioShareArgs extends com.pulumi.resources.ResourceArgs {
public static final PortfolioShareArgs Empty = new PortfolioShareArgs();
/**
* Language code. Valid values: `en` (English), `jp` (Japanese), `zh` (Chinese). Default value is `en`.
*
*/
@Import(name="acceptLanguage")
private @Nullable Output acceptLanguage;
/**
* @return Language code. Valid values: `en` (English), `jp` (Japanese), `zh` (Chinese). Default value is `en`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy