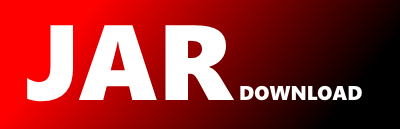
com.pulumi.aws.servicecatalog.outputs.GetPortfolioConstraintsDetail Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.servicecatalog.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetPortfolioConstraintsDetail {
/**
* @return Identifier of the constraint.
*
*/
private String constraintId;
/**
* @return Description of the constraint.
*
*/
private String description;
private String owner;
/**
* @return Portfolio identifier.
*
* The following arguments are optional:
*
*/
private String portfolioId;
/**
* @return Product identifier.
*
*/
private String productId;
/**
* @return Type of constraint. Valid values are `LAUNCH`, `NOTIFICATION`, `STACKSET`, and `TEMPLATE`.
*
*/
private String type;
private GetPortfolioConstraintsDetail() {}
/**
* @return Identifier of the constraint.
*
*/
public String constraintId() {
return this.constraintId;
}
/**
* @return Description of the constraint.
*
*/
public String description() {
return this.description;
}
public String owner() {
return this.owner;
}
/**
* @return Portfolio identifier.
*
* The following arguments are optional:
*
*/
public String portfolioId() {
return this.portfolioId;
}
/**
* @return Product identifier.
*
*/
public String productId() {
return this.productId;
}
/**
* @return Type of constraint. Valid values are `LAUNCH`, `NOTIFICATION`, `STACKSET`, and `TEMPLATE`.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPortfolioConstraintsDetail defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String constraintId;
private String description;
private String owner;
private String portfolioId;
private String productId;
private String type;
public Builder() {}
public Builder(GetPortfolioConstraintsDetail defaults) {
Objects.requireNonNull(defaults);
this.constraintId = defaults.constraintId;
this.description = defaults.description;
this.owner = defaults.owner;
this.portfolioId = defaults.portfolioId;
this.productId = defaults.productId;
this.type = defaults.type;
}
@CustomType.Setter
public Builder constraintId(String constraintId) {
if (constraintId == null) {
throw new MissingRequiredPropertyException("GetPortfolioConstraintsDetail", "constraintId");
}
this.constraintId = constraintId;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetPortfolioConstraintsDetail", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder owner(String owner) {
if (owner == null) {
throw new MissingRequiredPropertyException("GetPortfolioConstraintsDetail", "owner");
}
this.owner = owner;
return this;
}
@CustomType.Setter
public Builder portfolioId(String portfolioId) {
if (portfolioId == null) {
throw new MissingRequiredPropertyException("GetPortfolioConstraintsDetail", "portfolioId");
}
this.portfolioId = portfolioId;
return this;
}
@CustomType.Setter
public Builder productId(String productId) {
if (productId == null) {
throw new MissingRequiredPropertyException("GetPortfolioConstraintsDetail", "productId");
}
this.productId = productId;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetPortfolioConstraintsDetail", "type");
}
this.type = type;
return this;
}
public GetPortfolioConstraintsDetail build() {
final var _resultValue = new GetPortfolioConstraintsDetail();
_resultValue.constraintId = constraintId;
_resultValue.description = description;
_resultValue.owner = owner;
_resultValue.portfolioId = portfolioId;
_resultValue.productId = productId;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy