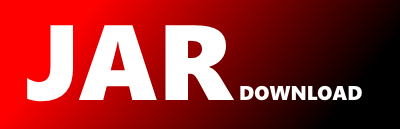
com.pulumi.aws.servicequotas.inputs.TemplateState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.servicequotas.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TemplateState extends com.pulumi.resources.ResourceArgs {
public static final TemplateState Empty = new TemplateState();
/**
* Indicates whether the quota is global.
*
*/
@Import(name="globalQuota")
private @Nullable Output globalQuota;
/**
* @return Indicates whether the quota is global.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy