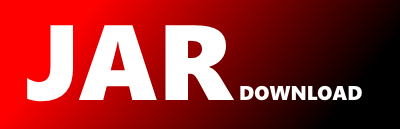
com.pulumi.aws.apigateway.BasePathMappingArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.apigateway;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BasePathMappingArgs extends com.pulumi.resources.ResourceArgs {
public static final BasePathMappingArgs Empty = new BasePathMappingArgs();
/**
* Path segment that must be prepended to the path when accessing the API via this mapping. If omitted, the API is exposed at the root of the given domain.
*
*/
@Import(name="basePath")
private @Nullable Output basePath;
/**
* @return Path segment that must be prepended to the path when accessing the API via this mapping. If omitted, the API is exposed at the root of the given domain.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy