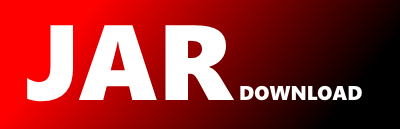
com.pulumi.aws.appconfig.inputs.EnvironmentState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.appconfig.inputs;
import com.pulumi.aws.appconfig.inputs.EnvironmentMonitorArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EnvironmentState extends com.pulumi.resources.ResourceArgs {
public static final EnvironmentState Empty = new EnvironmentState();
/**
* AppConfig application ID. Must be between 4 and 7 characters in length.
*
*/
@Import(name="applicationId")
private @Nullable Output applicationId;
/**
* @return AppConfig application ID. Must be between 4 and 7 characters in length.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy