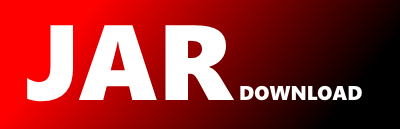
com.pulumi.aws.appfabric.AppAuthorization Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.appfabric;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.appfabric.AppAuthorizationArgs;
import com.pulumi.aws.appfabric.inputs.AppAuthorizationState;
import com.pulumi.aws.appfabric.outputs.AppAuthorizationCredential;
import com.pulumi.aws.appfabric.outputs.AppAuthorizationTenant;
import com.pulumi.aws.appfabric.outputs.AppAuthorizationTimeouts;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Resource for managing an AWS AppFabric App Authorization.
*
* ## Example Usage
*
* ### Basic Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.appfabric.AppAuthorization;
* import com.pulumi.aws.appfabric.AppAuthorizationArgs;
* import com.pulumi.aws.appfabric.inputs.AppAuthorizationCredentialArgs;
* import com.pulumi.aws.appfabric.inputs.AppAuthorizationTenantArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new AppAuthorization("example", AppAuthorizationArgs.builder()
* .app("TERRAFORMCLOUD")
* .appBundleArn(arn)
* .authType("apiKey")
* .credential(AppAuthorizationCredentialArgs.builder()
* .apiKeyCredentials(AppAuthorizationCredentialApiKeyCredentialArgs.builder()
* .apiKey("exampleapikeytoken")
* .build())
* .build())
* .tenants(AppAuthorizationTenantArgs.builder()
* .tenantDisplayName("example")
* .tenantIdentifier("example")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
*/
@ResourceType(type="aws:appfabric/appAuthorization:AppAuthorization")
public class AppAuthorization extends com.pulumi.resources.CustomResource {
/**
* The name of the application for valid values see https://docs.aws.amazon.com/appfabric/latest/api/API_CreateAppAuthorization.html.
*
*/
@Export(name="app", refs={String.class}, tree="[0]")
private Output app;
/**
* @return The name of the application for valid values see https://docs.aws.amazon.com/appfabric/latest/api/API_CreateAppAuthorization.html.
*
*/
public Output app() {
return this.app;
}
/**
* The Amazon Resource Name (ARN) of the app bundle to use for the request.
*
*/
@Export(name="appBundleArn", refs={String.class}, tree="[0]")
private Output appBundleArn;
/**
* @return The Amazon Resource Name (ARN) of the app bundle to use for the request.
*
*/
public Output appBundleArn() {
return this.appBundleArn;
}
/**
* ARN of the App Authorization. Do not begin the description with "An", "The", "Defines", "Indicates", or "Specifies," as these are verbose. In other words, "Indicates the amount of storage," can be rewritten as "Amount of storage," without losing any information.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the App Authorization. Do not begin the description with "An", "The", "Defines", "Indicates", or "Specifies," as these are verbose. In other words, "Indicates the amount of storage," can be rewritten as "Amount of storage," without losing any information.
*
*/
public Output arn() {
return this.arn;
}
/**
* The authorization type for the app authorization valid values are oauth2 and apiKey.
*
*/
@Export(name="authType", refs={String.class}, tree="[0]")
private Output authType;
/**
* @return The authorization type for the app authorization valid values are oauth2 and apiKey.
*
*/
public Output authType() {
return this.authType;
}
/**
* The application URL for the OAuth flow.
*
*/
@Export(name="authUrl", refs={String.class}, tree="[0]")
private Output authUrl;
/**
* @return The application URL for the OAuth flow.
*
*/
public Output authUrl() {
return this.authUrl;
}
@Export(name="createdAt", refs={String.class}, tree="[0]")
private Output createdAt;
public Output createdAt() {
return this.createdAt;
}
/**
* Contains credentials for the application, such as an API key or OAuth2 client ID and secret.
* Specify credentials that match the authorization type for your request. For example, if the authorization type for your request is OAuth2 (oauth2), then you should provide only the OAuth2 credentials.
*
*/
@Export(name="credential", refs={AppAuthorizationCredential.class}, tree="[0]")
private Output* @Nullable */ AppAuthorizationCredential> credential;
/**
* @return Contains credentials for the application, such as an API key or OAuth2 client ID and secret.
* Specify credentials that match the authorization type for your request. For example, if the authorization type for your request is OAuth2 (oauth2), then you should provide only the OAuth2 credentials.
*
*/
public Output> credential() {
return Codegen.optional(this.credential);
}
/**
* The user persona of the app authorization.
*
*/
@Export(name="persona", refs={String.class}, tree="[0]")
private Output persona;
/**
* @return The user persona of the app authorization.
*
*/
public Output persona() {
return this.persona;
}
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy