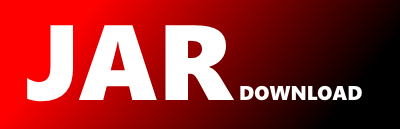
com.pulumi.aws.appstream.Stack Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.appstream;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.appstream.StackArgs;
import com.pulumi.aws.appstream.inputs.StackState;
import com.pulumi.aws.appstream.outputs.StackAccessEndpoint;
import com.pulumi.aws.appstream.outputs.StackApplicationSettings;
import com.pulumi.aws.appstream.outputs.StackStorageConnector;
import com.pulumi.aws.appstream.outputs.StackStreamingExperienceSettings;
import com.pulumi.aws.appstream.outputs.StackUserSetting;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an AppStream stack.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.appstream.Stack;
* import com.pulumi.aws.appstream.StackArgs;
* import com.pulumi.aws.appstream.inputs.StackStorageConnectorArgs;
* import com.pulumi.aws.appstream.inputs.StackUserSettingArgs;
* import com.pulumi.aws.appstream.inputs.StackApplicationSettingsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Stack("example", StackArgs.builder()
* .name("stack name")
* .description("stack description")
* .displayName("stack display name")
* .feedbackUrl("http://your-domain/feedback")
* .redirectUrl("http://your-domain/redirect")
* .storageConnectors(StackStorageConnectorArgs.builder()
* .connectorType("HOMEFOLDERS")
* .build())
* .userSettings(
* StackUserSettingArgs.builder()
* .action("CLIPBOARD_COPY_FROM_LOCAL_DEVICE")
* .permission("ENABLED")
* .build(),
* StackUserSettingArgs.builder()
* .action("CLIPBOARD_COPY_TO_LOCAL_DEVICE")
* .permission("ENABLED")
* .build(),
* StackUserSettingArgs.builder()
* .action("DOMAIN_PASSWORD_SIGNIN")
* .permission("ENABLED")
* .build(),
* StackUserSettingArgs.builder()
* .action("DOMAIN_SMART_CARD_SIGNIN")
* .permission("DISABLED")
* .build(),
* StackUserSettingArgs.builder()
* .action("FILE_DOWNLOAD")
* .permission("ENABLED")
* .build(),
* StackUserSettingArgs.builder()
* .action("FILE_UPLOAD")
* .permission("ENABLED")
* .build(),
* StackUserSettingArgs.builder()
* .action("PRINTING_TO_LOCAL_DEVICE")
* .permission("ENABLED")
* .build())
* .applicationSettings(StackApplicationSettingsArgs.builder()
* .enabled(true)
* .settingsGroup("SettingsGroup")
* .build())
* .tags(Map.of("TagName", "TagValue"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import `aws_appstream_stack` using the id. For example:
*
* ```sh
* $ pulumi import aws:appstream/stack:Stack example stackID
* ```
*
*/
@ResourceType(type="aws:appstream/stack:Stack")
public class Stack extends com.pulumi.resources.CustomResource {
/**
* Set of configuration blocks defining the interface VPC endpoints. Users of the stack can connect to AppStream 2.0 only through the specified endpoints.
* See `access_endpoints` below.
*
*/
@Export(name="accessEndpoints", refs={List.class,StackAccessEndpoint.class}, tree="[0,1]")
private Output> accessEndpoints;
/**
* @return Set of configuration blocks defining the interface VPC endpoints. Users of the stack can connect to AppStream 2.0 only through the specified endpoints.
* See `access_endpoints` below.
*
*/
public Output> accessEndpoints() {
return this.accessEndpoints;
}
/**
* Settings for application settings persistence.
* See `application_settings` below.
*
*/
@Export(name="applicationSettings", refs={StackApplicationSettings.class}, tree="[0]")
private Output applicationSettings;
/**
* @return Settings for application settings persistence.
* See `application_settings` below.
*
*/
public Output applicationSettings() {
return this.applicationSettings;
}
/**
* ARN of the appstream stack.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the appstream stack.
*
*/
public Output arn() {
return this.arn;
}
/**
* Date and time, in UTC and extended RFC 3339 format, when the stack was created.
*
*/
@Export(name="createdTime", refs={String.class}, tree="[0]")
private Output createdTime;
/**
* @return Date and time, in UTC and extended RFC 3339 format, when the stack was created.
*
*/
public Output createdTime() {
return this.createdTime;
}
/**
* Description for the AppStream stack.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description for the AppStream stack.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* Stack name to display.
*
*/
@Export(name="displayName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> displayName;
/**
* @return Stack name to display.
*
*/
public Output> displayName() {
return Codegen.optional(this.displayName);
}
/**
* Domains where AppStream 2.0 streaming sessions can be embedded in an iframe. You must approve the domains that you want to host embedded AppStream 2.0 streaming sessions.
*
*/
@Export(name="embedHostDomains", refs={List.class,String.class}, tree="[0,1]")
private Output> embedHostDomains;
/**
* @return Domains where AppStream 2.0 streaming sessions can be embedded in an iframe. You must approve the domains that you want to host embedded AppStream 2.0 streaming sessions.
*
*/
public Output> embedHostDomains() {
return this.embedHostDomains;
}
/**
* URL that users are redirected to after they click the Send Feedback link. If no URL is specified, no Send Feedback link is displayed. .
*
*/
@Export(name="feedbackUrl", refs={String.class}, tree="[0]")
private Output feedbackUrl;
/**
* @return URL that users are redirected to after they click the Send Feedback link. If no URL is specified, no Send Feedback link is displayed. .
*
*/
public Output feedbackUrl() {
return this.feedbackUrl;
}
/**
* Unique name for the AppStream stack.
*
* The following arguments are optional:
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Unique name for the AppStream stack.
*
* The following arguments are optional:
*
*/
public Output name() {
return this.name;
}
/**
* URL that users are redirected to after their streaming session ends.
*
*/
@Export(name="redirectUrl", refs={String.class}, tree="[0]")
private Output redirectUrl;
/**
* @return URL that users are redirected to after their streaming session ends.
*
*/
public Output redirectUrl() {
return this.redirectUrl;
}
/**
* Configuration block for the storage connectors to enable.
* See `storage_connectors` below.
*
*/
@Export(name="storageConnectors", refs={List.class,StackStorageConnector.class}, tree="[0,1]")
private Output> storageConnectors;
/**
* @return Configuration block for the storage connectors to enable.
* See `storage_connectors` below.
*
*/
public Output> storageConnectors() {
return this.storageConnectors;
}
/**
* The streaming protocol you want your stack to prefer. This can be UDP or TCP. Currently, UDP is only supported in the Windows native client.
* See `streaming_experience_settings` below.
*
*/
@Export(name="streamingExperienceSettings", refs={StackStreamingExperienceSettings.class}, tree="[0]")
private Output streamingExperienceSettings;
/**
* @return The streaming protocol you want your stack to prefer. This can be UDP or TCP. Currently, UDP is only supported in the Windows native client.
* See `streaming_experience_settings` below.
*
*/
public Output streamingExperienceSettings() {
return this.streamingExperienceSettings;
}
/**
* Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy