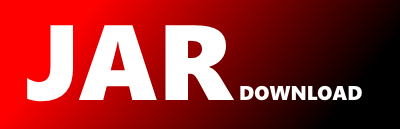
com.pulumi.aws.batch.outputs.ComputeEnvironmentComputeResources Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.batch.outputs;
import com.pulumi.aws.batch.outputs.ComputeEnvironmentComputeResourcesEc2Configuration;
import com.pulumi.aws.batch.outputs.ComputeEnvironmentComputeResourcesLaunchTemplate;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ComputeEnvironmentComputeResources {
/**
* @return The allocation strategy to use for the compute resource in case not enough instances of the best fitting instance type can be allocated. For valid values, refer to the [AWS documentation](https://docs.aws.amazon.com/batch/latest/APIReference/API_ComputeResource.html#Batch-Type-ComputeResource-allocationStrategy). Defaults to `BEST_FIT`. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
private @Nullable String allocationStrategy;
/**
* @return Integer of maximum percentage that a Spot Instance price can be when compared with the On-Demand price for that instance type before instances are launched. For example, if your bid percentage is 20% (`20`), then the Spot price must be below 20% of the current On-Demand price for that EC2 instance. If you leave this field empty, the default value is 100% of the On-Demand price. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
private @Nullable Integer bidPercentage;
/**
* @return The desired number of EC2 vCPUS in the compute environment. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
private @Nullable Integer desiredVcpus;
/**
* @return Provides information used to select Amazon Machine Images (AMIs) for EC2 instances in the compute environment. If Ec2Configuration isn't specified, the default is ECS_AL2. This parameter isn't applicable to jobs that are running on Fargate resources, and shouldn't be specified.
*
*/
private @Nullable List ec2Configurations;
/**
* @return The EC2 key pair that is used for instances launched in the compute environment. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
private @Nullable String ec2KeyPair;
/**
* @return The Amazon Machine Image (AMI) ID used for instances launched in the compute environment. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified. (Deprecated, use `ec2_configuration` `image_id_override` instead)
*
*/
private @Nullable String imageId;
/**
* @return The Amazon ECS instance role applied to Amazon EC2 instances in a compute environment. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
private @Nullable String instanceRole;
/**
* @return A list of instance types that may be launched. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
private @Nullable List instanceTypes;
/**
* @return The launch template to use for your compute resources. See details below. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
private @Nullable ComputeEnvironmentComputeResourcesLaunchTemplate launchTemplate;
/**
* @return The maximum number of EC2 vCPUs that an environment can reach.
*
*/
private Integer maxVcpus;
/**
* @return The minimum number of EC2 vCPUs that an environment should maintain. For `EC2` or `SPOT` compute environments, if the parameter is not explicitly defined, a `0` default value will be set. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
private @Nullable Integer minVcpus;
/**
* @return The Amazon EC2 placement group to associate with your compute resources.
*
*/
private @Nullable String placementGroup;
/**
* @return A list of EC2 security group that are associated with instances launched in the compute environment. This parameter is required for Fargate compute environments.
*
*/
private @Nullable List securityGroupIds;
/**
* @return The Amazon Resource Name (ARN) of the Amazon EC2 Spot Fleet IAM role applied to a SPOT compute environment. This parameter is required for SPOT compute environments. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
private @Nullable String spotIamFleetRole;
/**
* @return A list of VPC subnets into which the compute resources are launched.
*
*/
private List subnets;
/**
* @return Key-value pair tags to be applied to resources that are launched in the compute environment. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
private @Nullable Map tags;
/**
* @return The type of compute environment. Valid items are `EC2`, `SPOT`, `FARGATE` or `FARGATE_SPOT`.
*
*/
private String type;
private ComputeEnvironmentComputeResources() {}
/**
* @return The allocation strategy to use for the compute resource in case not enough instances of the best fitting instance type can be allocated. For valid values, refer to the [AWS documentation](https://docs.aws.amazon.com/batch/latest/APIReference/API_ComputeResource.html#Batch-Type-ComputeResource-allocationStrategy). Defaults to `BEST_FIT`. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
public Optional allocationStrategy() {
return Optional.ofNullable(this.allocationStrategy);
}
/**
* @return Integer of maximum percentage that a Spot Instance price can be when compared with the On-Demand price for that instance type before instances are launched. For example, if your bid percentage is 20% (`20`), then the Spot price must be below 20% of the current On-Demand price for that EC2 instance. If you leave this field empty, the default value is 100% of the On-Demand price. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
public Optional bidPercentage() {
return Optional.ofNullable(this.bidPercentage);
}
/**
* @return The desired number of EC2 vCPUS in the compute environment. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
public Optional desiredVcpus() {
return Optional.ofNullable(this.desiredVcpus);
}
/**
* @return Provides information used to select Amazon Machine Images (AMIs) for EC2 instances in the compute environment. If Ec2Configuration isn't specified, the default is ECS_AL2. This parameter isn't applicable to jobs that are running on Fargate resources, and shouldn't be specified.
*
*/
public List ec2Configurations() {
return this.ec2Configurations == null ? List.of() : this.ec2Configurations;
}
/**
* @return The EC2 key pair that is used for instances launched in the compute environment. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
public Optional ec2KeyPair() {
return Optional.ofNullable(this.ec2KeyPair);
}
/**
* @return The Amazon Machine Image (AMI) ID used for instances launched in the compute environment. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified. (Deprecated, use `ec2_configuration` `image_id_override` instead)
*
*/
public Optional imageId() {
return Optional.ofNullable(this.imageId);
}
/**
* @return The Amazon ECS instance role applied to Amazon EC2 instances in a compute environment. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
public Optional instanceRole() {
return Optional.ofNullable(this.instanceRole);
}
/**
* @return A list of instance types that may be launched. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
public List instanceTypes() {
return this.instanceTypes == null ? List.of() : this.instanceTypes;
}
/**
* @return The launch template to use for your compute resources. See details below. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
public Optional launchTemplate() {
return Optional.ofNullable(this.launchTemplate);
}
/**
* @return The maximum number of EC2 vCPUs that an environment can reach.
*
*/
public Integer maxVcpus() {
return this.maxVcpus;
}
/**
* @return The minimum number of EC2 vCPUs that an environment should maintain. For `EC2` or `SPOT` compute environments, if the parameter is not explicitly defined, a `0` default value will be set. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
public Optional minVcpus() {
return Optional.ofNullable(this.minVcpus);
}
/**
* @return The Amazon EC2 placement group to associate with your compute resources.
*
*/
public Optional placementGroup() {
return Optional.ofNullable(this.placementGroup);
}
/**
* @return A list of EC2 security group that are associated with instances launched in the compute environment. This parameter is required for Fargate compute environments.
*
*/
public List securityGroupIds() {
return this.securityGroupIds == null ? List.of() : this.securityGroupIds;
}
/**
* @return The Amazon Resource Name (ARN) of the Amazon EC2 Spot Fleet IAM role applied to a SPOT compute environment. This parameter is required for SPOT compute environments. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
public Optional spotIamFleetRole() {
return Optional.ofNullable(this.spotIamFleetRole);
}
/**
* @return A list of VPC subnets into which the compute resources are launched.
*
*/
public List subnets() {
return this.subnets;
}
/**
* @return Key-value pair tags to be applied to resources that are launched in the compute environment. This parameter isn't applicable to jobs running on Fargate resources, and shouldn't be specified.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of compute environment. Valid items are `EC2`, `SPOT`, `FARGATE` or `FARGATE_SPOT`.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ComputeEnvironmentComputeResources defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String allocationStrategy;
private @Nullable Integer bidPercentage;
private @Nullable Integer desiredVcpus;
private @Nullable List ec2Configurations;
private @Nullable String ec2KeyPair;
private @Nullable String imageId;
private @Nullable String instanceRole;
private @Nullable List instanceTypes;
private @Nullable ComputeEnvironmentComputeResourcesLaunchTemplate launchTemplate;
private Integer maxVcpus;
private @Nullable Integer minVcpus;
private @Nullable String placementGroup;
private @Nullable List securityGroupIds;
private @Nullable String spotIamFleetRole;
private List subnets;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(ComputeEnvironmentComputeResources defaults) {
Objects.requireNonNull(defaults);
this.allocationStrategy = defaults.allocationStrategy;
this.bidPercentage = defaults.bidPercentage;
this.desiredVcpus = defaults.desiredVcpus;
this.ec2Configurations = defaults.ec2Configurations;
this.ec2KeyPair = defaults.ec2KeyPair;
this.imageId = defaults.imageId;
this.instanceRole = defaults.instanceRole;
this.instanceTypes = defaults.instanceTypes;
this.launchTemplate = defaults.launchTemplate;
this.maxVcpus = defaults.maxVcpus;
this.minVcpus = defaults.minVcpus;
this.placementGroup = defaults.placementGroup;
this.securityGroupIds = defaults.securityGroupIds;
this.spotIamFleetRole = defaults.spotIamFleetRole;
this.subnets = defaults.subnets;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder allocationStrategy(@Nullable String allocationStrategy) {
this.allocationStrategy = allocationStrategy;
return this;
}
@CustomType.Setter
public Builder bidPercentage(@Nullable Integer bidPercentage) {
this.bidPercentage = bidPercentage;
return this;
}
@CustomType.Setter
public Builder desiredVcpus(@Nullable Integer desiredVcpus) {
this.desiredVcpus = desiredVcpus;
return this;
}
@CustomType.Setter
public Builder ec2Configurations(@Nullable List ec2Configurations) {
this.ec2Configurations = ec2Configurations;
return this;
}
public Builder ec2Configurations(ComputeEnvironmentComputeResourcesEc2Configuration... ec2Configurations) {
return ec2Configurations(List.of(ec2Configurations));
}
@CustomType.Setter
public Builder ec2KeyPair(@Nullable String ec2KeyPair) {
this.ec2KeyPair = ec2KeyPair;
return this;
}
@CustomType.Setter
public Builder imageId(@Nullable String imageId) {
this.imageId = imageId;
return this;
}
@CustomType.Setter
public Builder instanceRole(@Nullable String instanceRole) {
this.instanceRole = instanceRole;
return this;
}
@CustomType.Setter
public Builder instanceTypes(@Nullable List instanceTypes) {
this.instanceTypes = instanceTypes;
return this;
}
public Builder instanceTypes(String... instanceTypes) {
return instanceTypes(List.of(instanceTypes));
}
@CustomType.Setter
public Builder launchTemplate(@Nullable ComputeEnvironmentComputeResourcesLaunchTemplate launchTemplate) {
this.launchTemplate = launchTemplate;
return this;
}
@CustomType.Setter
public Builder maxVcpus(Integer maxVcpus) {
if (maxVcpus == null) {
throw new MissingRequiredPropertyException("ComputeEnvironmentComputeResources", "maxVcpus");
}
this.maxVcpus = maxVcpus;
return this;
}
@CustomType.Setter
public Builder minVcpus(@Nullable Integer minVcpus) {
this.minVcpus = minVcpus;
return this;
}
@CustomType.Setter
public Builder placementGroup(@Nullable String placementGroup) {
this.placementGroup = placementGroup;
return this;
}
@CustomType.Setter
public Builder securityGroupIds(@Nullable List securityGroupIds) {
this.securityGroupIds = securityGroupIds;
return this;
}
public Builder securityGroupIds(String... securityGroupIds) {
return securityGroupIds(List.of(securityGroupIds));
}
@CustomType.Setter
public Builder spotIamFleetRole(@Nullable String spotIamFleetRole) {
this.spotIamFleetRole = spotIamFleetRole;
return this;
}
@CustomType.Setter
public Builder subnets(List subnets) {
if (subnets == null) {
throw new MissingRequiredPropertyException("ComputeEnvironmentComputeResources", "subnets");
}
this.subnets = subnets;
return this;
}
public Builder subnets(String... subnets) {
return subnets(List.of(subnets));
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("ComputeEnvironmentComputeResources", "type");
}
this.type = type;
return this;
}
public ComputeEnvironmentComputeResources build() {
final var _resultValue = new ComputeEnvironmentComputeResources();
_resultValue.allocationStrategy = allocationStrategy;
_resultValue.bidPercentage = bidPercentage;
_resultValue.desiredVcpus = desiredVcpus;
_resultValue.ec2Configurations = ec2Configurations;
_resultValue.ec2KeyPair = ec2KeyPair;
_resultValue.imageId = imageId;
_resultValue.instanceRole = instanceRole;
_resultValue.instanceTypes = instanceTypes;
_resultValue.launchTemplate = launchTemplate;
_resultValue.maxVcpus = maxVcpus;
_resultValue.minVcpus = minVcpus;
_resultValue.placementGroup = placementGroup;
_resultValue.securityGroupIds = securityGroupIds;
_resultValue.spotIamFleetRole = spotIamFleetRole;
_resultValue.subnets = subnets;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy