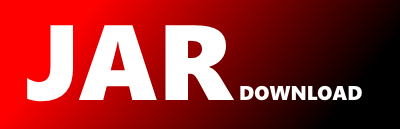
com.pulumi.aws.bedrock.CustomModelArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.bedrock;
import com.pulumi.aws.bedrock.inputs.CustomModelOutputDataConfigArgs;
import com.pulumi.aws.bedrock.inputs.CustomModelTimeoutsArgs;
import com.pulumi.aws.bedrock.inputs.CustomModelTrainingDataConfigArgs;
import com.pulumi.aws.bedrock.inputs.CustomModelValidationDataConfigArgs;
import com.pulumi.aws.bedrock.inputs.CustomModelVpcConfigArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CustomModelArgs extends com.pulumi.resources.ResourceArgs {
public static final CustomModelArgs Empty = new CustomModelArgs();
/**
* The Amazon Resource Name (ARN) of the base model.
*
*/
@Import(name="baseModelIdentifier", required=true)
private Output baseModelIdentifier;
/**
* @return The Amazon Resource Name (ARN) of the base model.
*
*/
public Output baseModelIdentifier() {
return this.baseModelIdentifier;
}
/**
* The custom model is encrypted at rest using this key. Specify the key ARN.
*
*/
@Import(name="customModelKmsKeyId")
private @Nullable Output customModelKmsKeyId;
/**
* @return The custom model is encrypted at rest using this key. Specify the key ARN.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy