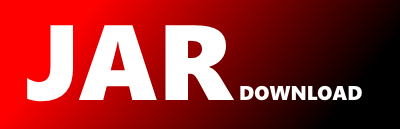
com.pulumi.aws.cloudtrail.TrailArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudtrail;
import com.pulumi.aws.cloudtrail.inputs.TrailAdvancedEventSelectorArgs;
import com.pulumi.aws.cloudtrail.inputs.TrailEventSelectorArgs;
import com.pulumi.aws.cloudtrail.inputs.TrailInsightSelectorArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TrailArgs extends com.pulumi.resources.ResourceArgs {
public static final TrailArgs Empty = new TrailArgs();
/**
* Specifies an advanced event selector for enabling data event logging. Fields documented below. Conflicts with `event_selector`.
*
*/
@Import(name="advancedEventSelectors")
private @Nullable Output> advancedEventSelectors;
/**
* @return Specifies an advanced event selector for enabling data event logging. Fields documented below. Conflicts with `event_selector`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy