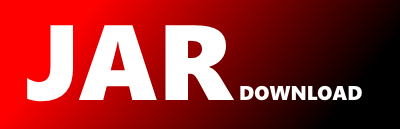
com.pulumi.aws.cloudwatch.EventTarget Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudwatch;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.cloudwatch.EventTargetArgs;
import com.pulumi.aws.cloudwatch.inputs.EventTargetState;
import com.pulumi.aws.cloudwatch.outputs.EventTargetBatchTarget;
import com.pulumi.aws.cloudwatch.outputs.EventTargetDeadLetterConfig;
import com.pulumi.aws.cloudwatch.outputs.EventTargetEcsTarget;
import com.pulumi.aws.cloudwatch.outputs.EventTargetHttpTarget;
import com.pulumi.aws.cloudwatch.outputs.EventTargetInputTransformer;
import com.pulumi.aws.cloudwatch.outputs.EventTargetKinesisTarget;
import com.pulumi.aws.cloudwatch.outputs.EventTargetRedshiftTarget;
import com.pulumi.aws.cloudwatch.outputs.EventTargetRetryPolicy;
import com.pulumi.aws.cloudwatch.outputs.EventTargetRunCommandTarget;
import com.pulumi.aws.cloudwatch.outputs.EventTargetSagemakerPipelineTarget;
import com.pulumi.aws.cloudwatch.outputs.EventTargetSqsTarget;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an EventBridge Target resource.
*
* > **Note:** EventBridge was formerly known as CloudWatch Events. The functionality is identical.
*
* ## Example Usage
*
* ### Kinesis Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.EventRule;
* import com.pulumi.aws.cloudwatch.EventRuleArgs;
* import com.pulumi.aws.kinesis.Stream;
* import com.pulumi.aws.kinesis.StreamArgs;
* import com.pulumi.aws.cloudwatch.EventTarget;
* import com.pulumi.aws.cloudwatch.EventTargetArgs;
* import com.pulumi.aws.cloudwatch.inputs.EventTargetRunCommandTargetArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var console = new EventRule("console", EventRuleArgs.builder()
* .name("capture-ec2-scaling-events")
* .description("Capture all EC2 scaling events")
* .eventPattern(serializeJson(
* jsonObject(
* jsonProperty("source", jsonArray("aws.autoscaling")),
* jsonProperty("detail-type", jsonArray(
* "EC2 Instance Launch Successful",
* "EC2 Instance Terminate Successful",
* "EC2 Instance Launch Unsuccessful",
* "EC2 Instance Terminate Unsuccessful"
* ))
* )))
* .build());
*
* var testStream = new Stream("testStream", StreamArgs.builder()
* .name("kinesis-test")
* .shardCount(1)
* .build());
*
* var yada = new EventTarget("yada", EventTargetArgs.builder()
* .targetId("Yada")
* .rule(console.name())
* .arn(testStream.arn())
* .runCommandTargets(
* EventTargetRunCommandTargetArgs.builder()
* .key("tag:Name")
* .values("FooBar")
* .build(),
* EventTargetRunCommandTargetArgs.builder()
* .key("InstanceIds")
* .values("i-162058cd308bffec2")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### SSM Document Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.ssm.Document;
* import com.pulumi.aws.ssm.DocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.iam.Policy;
* import com.pulumi.aws.iam.PolicyArgs;
* import com.pulumi.aws.iam.RolePolicyAttachment;
* import com.pulumi.aws.iam.RolePolicyAttachmentArgs;
* import com.pulumi.aws.cloudwatch.EventRule;
* import com.pulumi.aws.cloudwatch.EventRuleArgs;
* import com.pulumi.aws.cloudwatch.EventTarget;
* import com.pulumi.aws.cloudwatch.EventTargetArgs;
* import com.pulumi.aws.cloudwatch.inputs.EventTargetRunCommandTargetArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var ssmLifecycleTrust = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .actions("sts:AssumeRole")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("events.amazonaws.com")
* .build())
* .build())
* .build());
*
* var stopInstance = new Document("stopInstance", DocumentArgs.builder()
* .name("stop_instance")
* .documentType("Command")
* .content(serializeJson(
* jsonObject(
* jsonProperty("schemaVersion", "1.2"),
* jsonProperty("description", "Stop an instance"),
* jsonProperty("parameters", jsonObject(
*
* )),
* jsonProperty("runtimeConfig", jsonObject(
* jsonProperty("aws:runShellScript", jsonObject(
* jsonProperty("properties", jsonArray(jsonObject(
* jsonProperty("id", "0.aws:runShellScript"),
* jsonProperty("runCommand", jsonArray("halt"))
* )))
* ))
* ))
* )))
* .build());
*
* final var ssmLifecycle = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(
* GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions("ssm:SendCommand")
* .resources("arn:aws:ec2:eu-west-1:1234567890:instance/*")
* .conditions(GetPolicyDocumentStatementConditionArgs.builder()
* .test("StringEquals")
* .variable("ec2:ResourceTag/Terminate")
* .values("*")
* .build())
* .build(),
* GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions("ssm:SendCommand")
* .resources(stopInstance.arn())
* .build())
* .build());
*
* var ssmLifecycleRole = new Role("ssmLifecycleRole", RoleArgs.builder()
* .name("SSMLifecycle")
* .assumeRolePolicy(ssmLifecycleTrust.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* var ssmLifecyclePolicy = new Policy("ssmLifecyclePolicy", PolicyArgs.builder()
* .name("SSMLifecycle")
* .policy(ssmLifecycle.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(ssmLifecycle -> ssmLifecycle.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .build());
*
* var ssmLifecycleRolePolicyAttachment = new RolePolicyAttachment("ssmLifecycleRolePolicyAttachment", RolePolicyAttachmentArgs.builder()
* .policyArn(ssmLifecyclePolicy.arn())
* .role(ssmLifecycleRole.name())
* .build());
*
* var stopInstances = new EventRule("stopInstances", EventRuleArgs.builder()
* .name("StopInstance")
* .description("Stop instances nightly")
* .scheduleExpression("cron(0 0 * * ? *)")
* .build());
*
* var stopInstancesEventTarget = new EventTarget("stopInstancesEventTarget", EventTargetArgs.builder()
* .targetId("StopInstance")
* .arn(stopInstance.arn())
* .rule(stopInstances.name())
* .roleArn(ssmLifecycleRole.arn())
* .runCommandTargets(EventTargetRunCommandTargetArgs.builder()
* .key("tag:Terminate")
* .values("midnight")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### RunCommand Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.EventRule;
* import com.pulumi.aws.cloudwatch.EventRuleArgs;
* import com.pulumi.aws.cloudwatch.EventTarget;
* import com.pulumi.aws.cloudwatch.EventTargetArgs;
* import com.pulumi.aws.cloudwatch.inputs.EventTargetRunCommandTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var stopInstances = new EventRule("stopInstances", EventRuleArgs.builder()
* .name("StopInstance")
* .description("Stop instances nightly")
* .scheduleExpression("cron(0 0 * * ? *)")
* .build());
*
* var stopInstancesEventTarget = new EventTarget("stopInstancesEventTarget", EventTargetArgs.builder()
* .targetId("StopInstance")
* .arn(String.format("arn:aws:ssm:%s::document/AWS-RunShellScript", awsRegion))
* .input("{\"commands\":[\"halt\"]}")
* .rule(stopInstances.name())
* .roleArn(ssmLifecycle.arn())
* .runCommandTargets(EventTargetRunCommandTargetArgs.builder()
* .key("tag:Terminate")
* .values("midnight")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### ECS Run Task with Role and Task Override Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.iam.RolePolicy;
* import com.pulumi.aws.iam.RolePolicyArgs;
* import com.pulumi.aws.cloudwatch.EventTarget;
* import com.pulumi.aws.cloudwatch.EventTargetArgs;
* import com.pulumi.aws.cloudwatch.inputs.EventTargetEcsTargetArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var assumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("events.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
*
* var ecsEvents = new Role("ecsEvents", RoleArgs.builder()
* .name("ecs_events")
* .assumeRolePolicy(assumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* final var ecsEventsRunTaskWithAnyRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(
* GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions("iam:PassRole")
* .resources("*")
* .build(),
* GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions("ecs:RunTask")
* .resources(StdFunctions.replace(ReplaceArgs.builder()
* .text(taskName.arn())
* .search("/:\\d+$/")
* .replace(":*")
* .build()).result())
* .build())
* .build());
*
* var ecsEventsRunTaskWithAnyRoleRolePolicy = new RolePolicy("ecsEventsRunTaskWithAnyRoleRolePolicy", RolePolicyArgs.builder()
* .name("ecs_events_run_task_with_any_role")
* .role(ecsEvents.id())
* .policy(ecsEventsRunTaskWithAnyRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* var ecsScheduledTask = new EventTarget("ecsScheduledTask", EventTargetArgs.builder()
* .targetId("run-scheduled-task-every-hour")
* .arn(clusterName.arn())
* .rule(everyHour.name())
* .roleArn(ecsEvents.arn())
* .ecsTarget(EventTargetEcsTargetArgs.builder()
* .taskCount(1)
* .taskDefinitionArn(taskName.arn())
* .build())
* .input(serializeJson(
* jsonObject(
* jsonProperty("containerOverrides", jsonArray(jsonObject(
* jsonProperty("name", "name-of-container-to-override"),
* jsonProperty("command", jsonArray(
* "bin/console",
* "scheduled-task"
* ))
* )))
* )))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### API Gateway target
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.EventRule;
* import com.pulumi.aws.apigateway.Deployment;
* import com.pulumi.aws.apigateway.DeploymentArgs;
* import com.pulumi.aws.apigateway.Stage;
* import com.pulumi.aws.apigateway.StageArgs;
* import com.pulumi.aws.cloudwatch.EventTarget;
* import com.pulumi.aws.cloudwatch.EventTargetArgs;
* import com.pulumi.aws.cloudwatch.inputs.EventTargetHttpTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var exampleEventRule = new EventRule("exampleEventRule");
*
* var exampleDeployment = new Deployment("exampleDeployment", DeploymentArgs.builder()
* .restApi(exampleAwsApiGatewayRestApi.id())
* .build());
*
* var exampleStage = new Stage("exampleStage", StageArgs.builder()
* .restApi(exampleAwsApiGatewayRestApi.id())
* .deployment(exampleDeployment.id())
* .build());
*
* var example = new EventTarget("example", EventTargetArgs.builder()
* .arn(exampleStage.executionArn().applyValue(executionArn -> String.format("%s/GET", executionArn)))
* .rule(exampleEventRule.id())
* .httpTarget(EventTargetHttpTargetArgs.builder()
* .queryStringParameters(Map.of("Body", "$.detail.body"))
* .headerParameters(Map.of("Env", "Test"))
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Cross-Account Event Bus target
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.iam.Policy;
* import com.pulumi.aws.iam.PolicyArgs;
* import com.pulumi.aws.iam.RolePolicyAttachment;
* import com.pulumi.aws.iam.RolePolicyAttachmentArgs;
* import com.pulumi.aws.cloudwatch.EventRule;
* import com.pulumi.aws.cloudwatch.EventRuleArgs;
* import com.pulumi.aws.cloudwatch.EventTarget;
* import com.pulumi.aws.cloudwatch.EventTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var assumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("events.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
*
* var eventBusInvokeRemoteEventBusRole = new Role("eventBusInvokeRemoteEventBusRole", RoleArgs.builder()
* .name("event-bus-invoke-remote-event-bus")
* .assumeRolePolicy(assumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* final var eventBusInvokeRemoteEventBus = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions("events:PutEvents")
* .resources("arn:aws:events:eu-west-1:1234567890:event-bus/My-Event-Bus")
* .build())
* .build());
*
* var eventBusInvokeRemoteEventBusPolicy = new Policy("eventBusInvokeRemoteEventBusPolicy", PolicyArgs.builder()
* .name("event_bus_invoke_remote_event_bus")
* .policy(eventBusInvokeRemoteEventBus.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* var eventBusInvokeRemoteEventBusRolePolicyAttachment = new RolePolicyAttachment("eventBusInvokeRemoteEventBusRolePolicyAttachment", RolePolicyAttachmentArgs.builder()
* .role(eventBusInvokeRemoteEventBusRole.name())
* .policyArn(eventBusInvokeRemoteEventBusPolicy.arn())
* .build());
*
* var stopInstances = new EventRule("stopInstances", EventRuleArgs.builder()
* .name("StopInstance")
* .description("Stop instances nightly")
* .scheduleExpression("cron(0 0 * * ? *)")
* .build());
*
* var stopInstancesEventTarget = new EventTarget("stopInstancesEventTarget", EventTargetArgs.builder()
* .targetId("StopInstance")
* .arn("arn:aws:events:eu-west-1:1234567890:event-bus/My-Event-Bus")
* .rule(stopInstances.name())
* .roleArn(eventBusInvokeRemoteEventBusRole.arn())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Input Transformer Usage - JSON Object
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.EventRule;
* import com.pulumi.aws.cloudwatch.EventTarget;
* import com.pulumi.aws.cloudwatch.EventTargetArgs;
* import com.pulumi.aws.cloudwatch.inputs.EventTargetInputTransformerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var exampleEventRule = new EventRule("exampleEventRule");
*
* var example = new EventTarget("example", EventTargetArgs.builder()
* .arn(exampleAwsLambdaFunction.arn())
* .rule(exampleEventRule.id())
* .inputTransformer(EventTargetInputTransformerArgs.builder()
* .inputPaths(Map.ofEntries(
* Map.entry("instance", "$.detail.instance"),
* Map.entry("status", "$.detail.status")
* ))
* .inputTemplate("""
* {
* "instance_id": ,
* "instance_status":
* }
* """)
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Input Transformer Usage - Simple String
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.EventRule;
* import com.pulumi.aws.cloudwatch.EventTarget;
* import com.pulumi.aws.cloudwatch.EventTargetArgs;
* import com.pulumi.aws.cloudwatch.inputs.EventTargetInputTransformerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var exampleEventRule = new EventRule("exampleEventRule");
*
* var example = new EventTarget("example", EventTargetArgs.builder()
* .arn(exampleAwsLambdaFunction.arn())
* .rule(exampleEventRule.id())
* .inputTransformer(EventTargetInputTransformerArgs.builder()
* .inputPaths(Map.ofEntries(
* Map.entry("instance", "$.detail.instance"),
* Map.entry("status", "$.detail.status")
* ))
* .inputTemplate("\" is in state \"")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Cloudwatch Log Group Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.LogGroup;
* import com.pulumi.aws.cloudwatch.LogGroupArgs;
* import com.pulumi.aws.cloudwatch.EventRule;
* import com.pulumi.aws.cloudwatch.EventRuleArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.cloudwatch.LogResourcePolicy;
* import com.pulumi.aws.cloudwatch.LogResourcePolicyArgs;
* import com.pulumi.aws.cloudwatch.EventTarget;
* import com.pulumi.aws.cloudwatch.EventTargetArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new LogGroup("example", LogGroupArgs.builder()
* .name("/aws/events/guardduty/logs")
* .retentionInDays(1)
* .build());
*
* var exampleEventRule = new EventRule("exampleEventRule", EventRuleArgs.builder()
* .name("guard-duty_event_rule")
* .description("GuardDuty Findings")
* .eventPattern(serializeJson(
* jsonObject(
* jsonProperty("source", jsonArray("aws.guardduty"))
* )))
* .tags(Map.of("Environment", "example"))
* .build());
*
* final var exampleLogPolicy = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(
* GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions("logs:CreateLogStream")
* .resources(example.arn().applyValue(arn -> String.format("%s:*", arn)))
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers(
* "events.amazonaws.com",
* "delivery.logs.amazonaws.com")
* .build())
* .build(),
* GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions("logs:PutLogEvents")
* .resources(example.arn().applyValue(arn -> String.format("%s:*:*", arn)))
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers(
* "events.amazonaws.com",
* "delivery.logs.amazonaws.com")
* .build())
* .conditions(GetPolicyDocumentStatementConditionArgs.builder()
* .test("ArnEquals")
* .values(exampleEventRule.arn())
* .variable("aws:SourceArn")
* .build())
* .build())
* .build());
*
* var exampleLogResourcePolicy = new LogResourcePolicy("exampleLogResourcePolicy", LogResourcePolicyArgs.builder()
* .policyDocument(exampleLogPolicy.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(exampleLogPolicy -> exampleLogPolicy.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .policyName("guardduty-log-publishing-policy")
* .build());
*
* var exampleEventTarget = new EventTarget("exampleEventTarget", EventTargetArgs.builder()
* .rule(exampleEventRule.name())
* .arn(example.arn())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import EventBridge Targets using `event_bus_name/rule-name/target-id` (if you omit `event_bus_name`, the `default` event bus will be used). For example:
*
* ```sh
* $ pulumi import aws:cloudwatch/eventTarget:EventTarget test-event-target rule-name/target-id
* ```
*
*/
@ResourceType(type="aws:cloudwatch/eventTarget:EventTarget")
public class EventTarget extends com.pulumi.resources.CustomResource {
/**
* The Amazon Resource Name (ARN) of the target.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The Amazon Resource Name (ARN) of the target.
*
*/
public Output arn() {
return this.arn;
}
/**
* Parameters used when you are using the rule to invoke an Amazon Batch Job. Documented below. A maximum of 1 are allowed.
*
*/
@Export(name="batchTarget", refs={EventTargetBatchTarget.class}, tree="[0]")
private Output* @Nullable */ EventTargetBatchTarget> batchTarget;
/**
* @return Parameters used when you are using the rule to invoke an Amazon Batch Job. Documented below. A maximum of 1 are allowed.
*
*/
public Output> batchTarget() {
return Codegen.optional(this.batchTarget);
}
/**
* Parameters used when you are providing a dead letter config. Documented below. A maximum of 1 are allowed.
*
*/
@Export(name="deadLetterConfig", refs={EventTargetDeadLetterConfig.class}, tree="[0]")
private Output* @Nullable */ EventTargetDeadLetterConfig> deadLetterConfig;
/**
* @return Parameters used when you are providing a dead letter config. Documented below. A maximum of 1 are allowed.
*
*/
public Output> deadLetterConfig() {
return Codegen.optional(this.deadLetterConfig);
}
/**
* Parameters used when you are using the rule to invoke Amazon ECS Task. Documented below. A maximum of 1 are allowed.
*
*/
@Export(name="ecsTarget", refs={EventTargetEcsTarget.class}, tree="[0]")
private Output* @Nullable */ EventTargetEcsTarget> ecsTarget;
/**
* @return Parameters used when you are using the rule to invoke Amazon ECS Task. Documented below. A maximum of 1 are allowed.
*
*/
public Output> ecsTarget() {
return Codegen.optional(this.ecsTarget);
}
/**
* The name or ARN of the event bus to associate with the rule.
* If you omit this, the `default` event bus is used.
*
*/
@Export(name="eventBusName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> eventBusName;
/**
* @return The name or ARN of the event bus to associate with the rule.
* If you omit this, the `default` event bus is used.
*
*/
public Output> eventBusName() {
return Codegen.optional(this.eventBusName);
}
/**
* Used to delete managed rules created by AWS. Defaults to `false`.
*
*/
@Export(name="forceDestroy", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> forceDestroy;
/**
* @return Used to delete managed rules created by AWS. Defaults to `false`.
*
*/
public Output> forceDestroy() {
return Codegen.optional(this.forceDestroy);
}
/**
* Parameters used when you are using the rule to invoke an API Gateway REST endpoint. Documented below. A maximum of 1 is allowed.
*
*/
@Export(name="httpTarget", refs={EventTargetHttpTarget.class}, tree="[0]")
private Output* @Nullable */ EventTargetHttpTarget> httpTarget;
/**
* @return Parameters used when you are using the rule to invoke an API Gateway REST endpoint. Documented below. A maximum of 1 is allowed.
*
*/
public Output> httpTarget() {
return Codegen.optional(this.httpTarget);
}
/**
* Valid JSON text passed to the target. Conflicts with `input_path` and `input_transformer`.
*
*/
@Export(name="input", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> input;
/**
* @return Valid JSON text passed to the target. Conflicts with `input_path` and `input_transformer`.
*
*/
public Output> input() {
return Codegen.optional(this.input);
}
/**
* The value of the [JSONPath](http://goessner.net/articles/JsonPath/) that is used for extracting part of the matched event when passing it to the target. Conflicts with `input` and `input_transformer`.
*
*/
@Export(name="inputPath", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> inputPath;
/**
* @return The value of the [JSONPath](http://goessner.net/articles/JsonPath/) that is used for extracting part of the matched event when passing it to the target. Conflicts with `input` and `input_transformer`.
*
*/
public Output> inputPath() {
return Codegen.optional(this.inputPath);
}
/**
* Parameters used when you are providing a custom input to a target based on certain event data. Conflicts with `input` and `input_path`.
*
*/
@Export(name="inputTransformer", refs={EventTargetInputTransformer.class}, tree="[0]")
private Output* @Nullable */ EventTargetInputTransformer> inputTransformer;
/**
* @return Parameters used when you are providing a custom input to a target based on certain event data. Conflicts with `input` and `input_path`.
*
*/
public Output> inputTransformer() {
return Codegen.optional(this.inputTransformer);
}
/**
* Parameters used when you are using the rule to invoke an Amazon Kinesis Stream. Documented below. A maximum of 1 are allowed.
*
*/
@Export(name="kinesisTarget", refs={EventTargetKinesisTarget.class}, tree="[0]")
private Output* @Nullable */ EventTargetKinesisTarget> kinesisTarget;
/**
* @return Parameters used when you are using the rule to invoke an Amazon Kinesis Stream. Documented below. A maximum of 1 are allowed.
*
*/
public Output> kinesisTarget() {
return Codegen.optional(this.kinesisTarget);
}
/**
* Parameters used when you are using the rule to invoke an Amazon Redshift Statement. Documented below. A maximum of 1 are allowed.
*
*/
@Export(name="redshiftTarget", refs={EventTargetRedshiftTarget.class}, tree="[0]")
private Output* @Nullable */ EventTargetRedshiftTarget> redshiftTarget;
/**
* @return Parameters used when you are using the rule to invoke an Amazon Redshift Statement. Documented below. A maximum of 1 are allowed.
*
*/
public Output> redshiftTarget() {
return Codegen.optional(this.redshiftTarget);
}
/**
* Parameters used when you are providing retry policies. Documented below. A maximum of 1 are allowed.
*
*/
@Export(name="retryPolicy", refs={EventTargetRetryPolicy.class}, tree="[0]")
private Output* @Nullable */ EventTargetRetryPolicy> retryPolicy;
/**
* @return Parameters used when you are providing retry policies. Documented below. A maximum of 1 are allowed.
*
*/
public Output> retryPolicy() {
return Codegen.optional(this.retryPolicy);
}
/**
* The Amazon Resource Name (ARN) of the IAM role to be used for this target when the rule is triggered. Required if `ecs_target` is used or target in `arn` is EC2 instance, Kinesis data stream, Step Functions state machine, or Event Bus in different account or region.
*
*/
@Export(name="roleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> roleArn;
/**
* @return The Amazon Resource Name (ARN) of the IAM role to be used for this target when the rule is triggered. Required if `ecs_target` is used or target in `arn` is EC2 instance, Kinesis data stream, Step Functions state machine, or Event Bus in different account or region.
*
*/
public Output> roleArn() {
return Codegen.optional(this.roleArn);
}
/**
* The name of the rule you want to add targets to.
*
* The following arguments are optional:
*
*/
@Export(name="rule", refs={String.class}, tree="[0]")
private Output rule;
/**
* @return The name of the rule you want to add targets to.
*
* The following arguments are optional:
*
*/
public Output rule() {
return this.rule;
}
/**
* Parameters used when you are using the rule to invoke Amazon EC2 Run Command. Documented below. A maximum of 5 are allowed.
*
*/
@Export(name="runCommandTargets", refs={List.class,EventTargetRunCommandTarget.class}, tree="[0,1]")
private Output* @Nullable */ List> runCommandTargets;
/**
* @return Parameters used when you are using the rule to invoke Amazon EC2 Run Command. Documented below. A maximum of 5 are allowed.
*
*/
public Output>> runCommandTargets() {
return Codegen.optional(this.runCommandTargets);
}
/**
* Parameters used when you are using the rule to invoke an Amazon SageMaker Pipeline. Documented below. A maximum of 1 are allowed.
*
*/
@Export(name="sagemakerPipelineTarget", refs={EventTargetSagemakerPipelineTarget.class}, tree="[0]")
private Output* @Nullable */ EventTargetSagemakerPipelineTarget> sagemakerPipelineTarget;
/**
* @return Parameters used when you are using the rule to invoke an Amazon SageMaker Pipeline. Documented below. A maximum of 1 are allowed.
*
*/
public Output> sagemakerPipelineTarget() {
return Codegen.optional(this.sagemakerPipelineTarget);
}
/**
* Parameters used when you are using the rule to invoke an Amazon SQS Queue. Documented below. A maximum of 1 are allowed.
*
*/
@Export(name="sqsTarget", refs={EventTargetSqsTarget.class}, tree="[0]")
private Output* @Nullable */ EventTargetSqsTarget> sqsTarget;
/**
* @return Parameters used when you are using the rule to invoke an Amazon SQS Queue. Documented below. A maximum of 1 are allowed.
*
*/
public Output> sqsTarget() {
return Codegen.optional(this.sqsTarget);
}
/**
* The unique target assignment ID. If missing, will generate a random, unique id.
*
*/
@Export(name="targetId", refs={String.class}, tree="[0]")
private Output targetId;
/**
* @return The unique target assignment ID. If missing, will generate a random, unique id.
*
*/
public Output targetId() {
return this.targetId;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public EventTarget(java.lang.String name) {
this(name, EventTargetArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public EventTarget(java.lang.String name, EventTargetArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public EventTarget(java.lang.String name, EventTargetArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:cloudwatch/eventTarget:EventTarget", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private EventTarget(java.lang.String name, Output id, @Nullable EventTargetState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:cloudwatch/eventTarget:EventTarget", name, state, makeResourceOptions(options, id), false);
}
private static EventTargetArgs makeArgs(EventTargetArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? EventTargetArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static EventTarget get(java.lang.String name, Output id, @Nullable EventTargetState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new EventTarget(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy