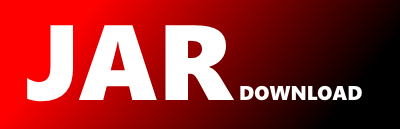
com.pulumi.aws.codebuild.outputs.ProjectSource Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.codebuild.outputs;
import com.pulumi.aws.codebuild.outputs.ProjectSourceBuildStatusConfig;
import com.pulumi.aws.codebuild.outputs.ProjectSourceGitSubmodulesConfig;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ProjectSource {
/**
* @return Configuration block that contains information that defines how the build project reports the build status to the source provider. This option is only used when the source provider is GitHub, GitHub Enterprise, GitLab, GitLab Self Managed, or Bitbucket. `build_status_config` blocks are documented below.
*
*/
private @Nullable ProjectSourceBuildStatusConfig buildStatusConfig;
/**
* @return Build specification to use for this build project's related builds. This must be set when `type` is `NO_SOURCE`. Also, if a non-default buildspec file name or file path aside from the root is used, it must be specified.
*
*/
private @Nullable String buildspec;
/**
* @return Truncate git history to this many commits. Use `0` for a `Full` checkout which you need to run commands like `git branch --show-current`. See [AWS CodePipeline User Guide: Tutorial: Use full clone with a GitHub pipeline source](https://docs.aws.amazon.com/codepipeline/latest/userguide/tutorials-github-gitclone.html) for details.
*
*/
private @Nullable Integer gitCloneDepth;
/**
* @return Configuration block. Detailed below.
*
*/
private @Nullable ProjectSourceGitSubmodulesConfig gitSubmodulesConfig;
/**
* @return Ignore SSL warnings when connecting to source control.
*
*/
private @Nullable Boolean insecureSsl;
/**
* @return Location of the source code from git or s3.
*
*/
private @Nullable String location;
/**
* @return Whether to report the status of a build's start and finish to your source provider. This option is valid only when your source provider is GitHub, GitHub Enterprise, GitLab, GitLab Self Managed, or Bitbucket.
*
*/
private @Nullable Boolean reportBuildStatus;
/**
* @return Type of repository that contains the source code to be built. Valid values: `BITBUCKET`, `CODECOMMIT`, `CODEPIPELINE`, `GITHUB`, `GITHUB_ENTERPRISE`, `GITLAB`, `GITLAB_SELF_MANAGED`, `NO_SOURCE`, `S3`.
*
*/
private String type;
private ProjectSource() {}
/**
* @return Configuration block that contains information that defines how the build project reports the build status to the source provider. This option is only used when the source provider is GitHub, GitHub Enterprise, GitLab, GitLab Self Managed, or Bitbucket. `build_status_config` blocks are documented below.
*
*/
public Optional buildStatusConfig() {
return Optional.ofNullable(this.buildStatusConfig);
}
/**
* @return Build specification to use for this build project's related builds. This must be set when `type` is `NO_SOURCE`. Also, if a non-default buildspec file name or file path aside from the root is used, it must be specified.
*
*/
public Optional buildspec() {
return Optional.ofNullable(this.buildspec);
}
/**
* @return Truncate git history to this many commits. Use `0` for a `Full` checkout which you need to run commands like `git branch --show-current`. See [AWS CodePipeline User Guide: Tutorial: Use full clone with a GitHub pipeline source](https://docs.aws.amazon.com/codepipeline/latest/userguide/tutorials-github-gitclone.html) for details.
*
*/
public Optional gitCloneDepth() {
return Optional.ofNullable(this.gitCloneDepth);
}
/**
* @return Configuration block. Detailed below.
*
*/
public Optional gitSubmodulesConfig() {
return Optional.ofNullable(this.gitSubmodulesConfig);
}
/**
* @return Ignore SSL warnings when connecting to source control.
*
*/
public Optional insecureSsl() {
return Optional.ofNullable(this.insecureSsl);
}
/**
* @return Location of the source code from git or s3.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return Whether to report the status of a build's start and finish to your source provider. This option is valid only when your source provider is GitHub, GitHub Enterprise, GitLab, GitLab Self Managed, or Bitbucket.
*
*/
public Optional reportBuildStatus() {
return Optional.ofNullable(this.reportBuildStatus);
}
/**
* @return Type of repository that contains the source code to be built. Valid values: `BITBUCKET`, `CODECOMMIT`, `CODEPIPELINE`, `GITHUB`, `GITHUB_ENTERPRISE`, `GITLAB`, `GITLAB_SELF_MANAGED`, `NO_SOURCE`, `S3`.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ProjectSource defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable ProjectSourceBuildStatusConfig buildStatusConfig;
private @Nullable String buildspec;
private @Nullable Integer gitCloneDepth;
private @Nullable ProjectSourceGitSubmodulesConfig gitSubmodulesConfig;
private @Nullable Boolean insecureSsl;
private @Nullable String location;
private @Nullable Boolean reportBuildStatus;
private String type;
public Builder() {}
public Builder(ProjectSource defaults) {
Objects.requireNonNull(defaults);
this.buildStatusConfig = defaults.buildStatusConfig;
this.buildspec = defaults.buildspec;
this.gitCloneDepth = defaults.gitCloneDepth;
this.gitSubmodulesConfig = defaults.gitSubmodulesConfig;
this.insecureSsl = defaults.insecureSsl;
this.location = defaults.location;
this.reportBuildStatus = defaults.reportBuildStatus;
this.type = defaults.type;
}
@CustomType.Setter
public Builder buildStatusConfig(@Nullable ProjectSourceBuildStatusConfig buildStatusConfig) {
this.buildStatusConfig = buildStatusConfig;
return this;
}
@CustomType.Setter
public Builder buildspec(@Nullable String buildspec) {
this.buildspec = buildspec;
return this;
}
@CustomType.Setter
public Builder gitCloneDepth(@Nullable Integer gitCloneDepth) {
this.gitCloneDepth = gitCloneDepth;
return this;
}
@CustomType.Setter
public Builder gitSubmodulesConfig(@Nullable ProjectSourceGitSubmodulesConfig gitSubmodulesConfig) {
this.gitSubmodulesConfig = gitSubmodulesConfig;
return this;
}
@CustomType.Setter
public Builder insecureSsl(@Nullable Boolean insecureSsl) {
this.insecureSsl = insecureSsl;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder reportBuildStatus(@Nullable Boolean reportBuildStatus) {
this.reportBuildStatus = reportBuildStatus;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("ProjectSource", "type");
}
this.type = type;
return this;
}
public ProjectSource build() {
final var _resultValue = new ProjectSource();
_resultValue.buildStatusConfig = buildStatusConfig;
_resultValue.buildspec = buildspec;
_resultValue.gitCloneDepth = gitCloneDepth;
_resultValue.gitSubmodulesConfig = gitSubmodulesConfig;
_resultValue.insecureSsl = insecureSsl;
_resultValue.location = location;
_resultValue.reportBuildStatus = reportBuildStatus;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy