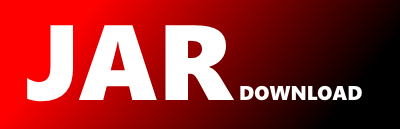
com.pulumi.aws.cognito.inputs.UserPoolLambdaConfigArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cognito.inputs;
import com.pulumi.aws.cognito.inputs.UserPoolLambdaConfigCustomEmailSenderArgs;
import com.pulumi.aws.cognito.inputs.UserPoolLambdaConfigCustomSmsSenderArgs;
import com.pulumi.aws.cognito.inputs.UserPoolLambdaConfigPreTokenGenerationConfigArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class UserPoolLambdaConfigArgs extends com.pulumi.resources.ResourceArgs {
public static final UserPoolLambdaConfigArgs Empty = new UserPoolLambdaConfigArgs();
/**
* ARN of the lambda creating an authentication challenge.
*
*/
@Import(name="createAuthChallenge")
private @Nullable Output createAuthChallenge;
/**
* @return ARN of the lambda creating an authentication challenge.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy