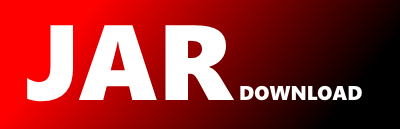
com.pulumi.aws.directconnect.inputs.TransitVirtualInterfaceState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.directconnect.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TransitVirtualInterfaceState extends com.pulumi.resources.ResourceArgs {
public static final TransitVirtualInterfaceState Empty = new TransitVirtualInterfaceState();
/**
* The address family for the BGP peer. ` ipv4 ` or `ipv6`.
*
*/
@Import(name="addressFamily")
private @Nullable Output addressFamily;
/**
* @return The address family for the BGP peer. ` ipv4 ` or `ipv6`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy