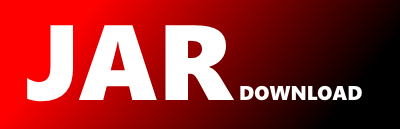
com.pulumi.aws.ec2.inputs.FleetOnDemandOptionsArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.inputs;
import com.pulumi.aws.ec2.inputs.FleetOnDemandOptionsCapacityReservationOptionsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class FleetOnDemandOptionsArgs extends com.pulumi.resources.ResourceArgs {
public static final FleetOnDemandOptionsArgs Empty = new FleetOnDemandOptionsArgs();
/**
* The order of the launch template overrides to use in fulfilling On-Demand capacity. Valid values: `lowestPrice`, `prioritized`. Default: `lowestPrice`.
*
*/
@Import(name="allocationStrategy")
private @Nullable Output allocationStrategy;
/**
* @return The order of the launch template overrides to use in fulfilling On-Demand capacity. Valid values: `lowestPrice`, `prioritized`. Default: `lowestPrice`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy