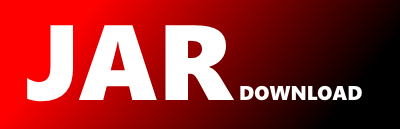
com.pulumi.aws.ec2.inputs.VpcEndpointConnectionNotificationState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class VpcEndpointConnectionNotificationState extends com.pulumi.resources.ResourceArgs {
public static final VpcEndpointConnectionNotificationState Empty = new VpcEndpointConnectionNotificationState();
/**
* One or more endpoint [events](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateVpcEndpointConnectionNotification.html#API_CreateVpcEndpointConnectionNotification_RequestParameters) for which to receive notifications.
*
* > **NOTE:** One of `vpc_endpoint_service_id` or `vpc_endpoint_id` must be specified.
*
*/
@Import(name="connectionEvents")
private @Nullable Output> connectionEvents;
/**
* @return One or more endpoint [events](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_CreateVpcEndpointConnectionNotification.html#API_CreateVpcEndpointConnectionNotification_RequestParameters) for which to receive notifications.
*
* > **NOTE:** One of `vpc_endpoint_service_id` or `vpc_endpoint_id` must be specified.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy