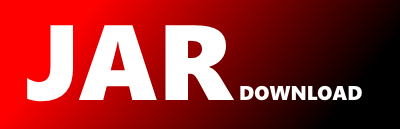
com.pulumi.aws.ec2.outputs.LaunchTemplateInstanceRequirements Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.aws.ec2.outputs.LaunchTemplateInstanceRequirementsAcceleratorCount;
import com.pulumi.aws.ec2.outputs.LaunchTemplateInstanceRequirementsAcceleratorTotalMemoryMib;
import com.pulumi.aws.ec2.outputs.LaunchTemplateInstanceRequirementsBaselineEbsBandwidthMbps;
import com.pulumi.aws.ec2.outputs.LaunchTemplateInstanceRequirementsMemoryGibPerVcpu;
import com.pulumi.aws.ec2.outputs.LaunchTemplateInstanceRequirementsMemoryMib;
import com.pulumi.aws.ec2.outputs.LaunchTemplateInstanceRequirementsNetworkBandwidthGbps;
import com.pulumi.aws.ec2.outputs.LaunchTemplateInstanceRequirementsNetworkInterfaceCount;
import com.pulumi.aws.ec2.outputs.LaunchTemplateInstanceRequirementsTotalLocalStorageGb;
import com.pulumi.aws.ec2.outputs.LaunchTemplateInstanceRequirementsVcpuCount;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class LaunchTemplateInstanceRequirements {
/**
* @return Block describing the minimum and maximum number of accelerators (GPUs, FPGAs, or AWS Inferentia chips). Default is no minimum or maximum.
*
*/
private @Nullable LaunchTemplateInstanceRequirementsAcceleratorCount acceleratorCount;
/**
* @return List of accelerator manufacturer names. Default is any manufacturer.
*
*/
private @Nullable List acceleratorManufacturers;
/**
* @return List of accelerator names. Default is any acclerator.
*
*/
private @Nullable List acceleratorNames;
/**
* @return Block describing the minimum and maximum total memory of the accelerators. Default is no minimum or maximum.
*
*/
private @Nullable LaunchTemplateInstanceRequirementsAcceleratorTotalMemoryMib acceleratorTotalMemoryMib;
/**
* @return List of accelerator types. Default is any accelerator type.
*
*/
private @Nullable List acceleratorTypes;
/**
* @return List of instance types to apply your specified attributes against. All other instance types are ignored, even if they match your specified attributes. You can use strings with one or more wild cards, represented by an asterisk (\*), to allow an instance type, size, or generation. The following are examples: `m5.8xlarge`, `c5*.*`, `m5a.*`, `r*`, `*3*`. For example, if you specify `c5*`, you are allowing the entire C5 instance family, which includes all C5a and C5n instance types. If you specify `m5a.*`, you are allowing all the M5a instance types, but not the M5n instance types. Maximum of 400 entries in the list; each entry is limited to 30 characters. Default is all instance types.
*
* > **NOTE:** If you specify `allowed_instance_types`, you can't specify `excluded_instance_types`.
*
*/
private @Nullable List allowedInstanceTypes;
/**
* @return Indicate whether bare metal instace types should be `included`, `excluded`, or `required`. Default is `excluded`.
*
*/
private @Nullable String bareMetal;
/**
* @return Block describing the minimum and maximum baseline EBS bandwidth, in Mbps. Default is no minimum or maximum.
*
*/
private @Nullable LaunchTemplateInstanceRequirementsBaselineEbsBandwidthMbps baselineEbsBandwidthMbps;
/**
* @return Indicate whether burstable performance instance types should be `included`, `excluded`, or `required`. Default is `excluded`.
*
*/
private @Nullable String burstablePerformance;
/**
* @return List of CPU manufacturer names. Default is any manufacturer.
*
* > **NOTE:** Don't confuse the CPU hardware manufacturer with the CPU hardware architecture. Instances will be launched with a compatible CPU architecture based on the Amazon Machine Image (AMI) that you specify in your launch template.
*
*/
private @Nullable List cpuManufacturers;
/**
* @return List of instance types to exclude. You can use strings with one or more wild cards, represented by an asterisk (\*), to exclude an instance type, size, or generation. The following are examples: `m5.8xlarge`, `c5*.*`, `m5a.*`, `r*`, `*3*`. For example, if you specify `c5*`, you are excluding the entire C5 instance family, which includes all C5a and C5n instance types. If you specify `m5a.*`, you are excluding all the M5a instance types, but not the M5n instance types. Maximum of 400 entries in the list; each entry is limited to 30 characters. Default is no excluded instance types.
*
* > **NOTE:** If you specify `excluded_instance_types`, you can't specify `allowed_instance_types`.
*
*/
private @Nullable List excludedInstanceTypes;
/**
* @return List of instance generation names. Default is any generation.
*
*/
private @Nullable List instanceGenerations;
/**
* @return Indicate whether instance types with local storage volumes are `included`, `excluded`, or `required`. Default is `included`.
*
*/
private @Nullable String localStorage;
/**
* @return List of local storage type names. Default any storage type.
*
*/
private @Nullable List localStorageTypes;
/**
* @return The price protection threshold for Spot Instances. This is the maximum you’ll pay for a Spot Instance, expressed as a percentage higher than the cheapest M, C, or R instance type with your specified attributes. When Amazon EC2 Auto Scaling selects instance types with your attributes, we will exclude instance types whose price is higher than your threshold. The parameter accepts an integer, which Amazon EC2 Auto Scaling interprets as a percentage. To turn off price protection, specify a high value, such as 999999. Conflicts with `spot_max_price_percentage_over_lowest_price`
*
*/
private @Nullable Integer maxSpotPriceAsPercentageOfOptimalOnDemandPrice;
/**
* @return Block describing the minimum and maximum amount of memory (GiB) per vCPU. Default is no minimum or maximum.
*
*/
private @Nullable LaunchTemplateInstanceRequirementsMemoryGibPerVcpu memoryGibPerVcpu;
/**
* @return Block describing the minimum and maximum amount of memory (MiB). Default is no maximum.
*
*/
private LaunchTemplateInstanceRequirementsMemoryMib memoryMib;
/**
* @return Block describing the minimum and maximum amount of network bandwidth, in gigabits per second (Gbps). Default is no minimum or maximum.
*
*/
private @Nullable LaunchTemplateInstanceRequirementsNetworkBandwidthGbps networkBandwidthGbps;
/**
* @return Block describing the minimum and maximum number of network interfaces. Default is no minimum or maximum.
*
*/
private @Nullable LaunchTemplateInstanceRequirementsNetworkInterfaceCount networkInterfaceCount;
/**
* @return The price protection threshold for On-Demand Instances. This is the maximum you’ll pay for an On-Demand Instance, expressed as a percentage higher than the cheapest M, C, or R instance type with your specified attributes. When Amazon EC2 Auto Scaling selects instance types with your attributes, we will exclude instance types whose price is higher than your threshold. The parameter accepts an integer, which Amazon EC2 Auto Scaling interprets as a percentage. To turn off price protection, specify a high value, such as 999999. Default is 20.
*
* If you set DesiredCapacityType to vcpu or memory-mib, the price protection threshold is applied based on the per vCPU or per memory price instead of the per instance price.
*
*/
private @Nullable Integer onDemandMaxPricePercentageOverLowestPrice;
/**
* @return Indicate whether instance types must support On-Demand Instance Hibernation, either `true` or `false`. Default is `false`.
*
*/
private @Nullable Boolean requireHibernateSupport;
/**
* @return The price protection threshold for Spot Instances. This is the maximum you’ll pay for a Spot Instance, expressed as a percentage higher than the cheapest M, C, or R instance type with your specified attributes. When Amazon EC2 Auto Scaling selects instance types with your attributes, we will exclude instance types whose price is higher than your threshold. The parameter accepts an integer, which Amazon EC2 Auto Scaling interprets as a percentage. To turn off price protection, specify a high value, such as 999999. Default is 100. Conflicts with `max_spot_price_as_percentage_of_optimal_on_demand_price`
*
* If you set DesiredCapacityType to vcpu or memory-mib, the price protection threshold is applied based on the per vCPU or per memory price instead of the per instance price.
*
*/
private @Nullable Integer spotMaxPricePercentageOverLowestPrice;
/**
* @return Block describing the minimum and maximum total local storage (GB). Default is no minimum or maximum.
*
*/
private @Nullable LaunchTemplateInstanceRequirementsTotalLocalStorageGb totalLocalStorageGb;
/**
* @return Block describing the minimum and maximum number of vCPUs. Default is no maximum.
*
*/
private LaunchTemplateInstanceRequirementsVcpuCount vcpuCount;
private LaunchTemplateInstanceRequirements() {}
/**
* @return Block describing the minimum and maximum number of accelerators (GPUs, FPGAs, or AWS Inferentia chips). Default is no minimum or maximum.
*
*/
public Optional acceleratorCount() {
return Optional.ofNullable(this.acceleratorCount);
}
/**
* @return List of accelerator manufacturer names. Default is any manufacturer.
*
*/
public List acceleratorManufacturers() {
return this.acceleratorManufacturers == null ? List.of() : this.acceleratorManufacturers;
}
/**
* @return List of accelerator names. Default is any acclerator.
*
*/
public List acceleratorNames() {
return this.acceleratorNames == null ? List.of() : this.acceleratorNames;
}
/**
* @return Block describing the minimum and maximum total memory of the accelerators. Default is no minimum or maximum.
*
*/
public Optional acceleratorTotalMemoryMib() {
return Optional.ofNullable(this.acceleratorTotalMemoryMib);
}
/**
* @return List of accelerator types. Default is any accelerator type.
*
*/
public List acceleratorTypes() {
return this.acceleratorTypes == null ? List.of() : this.acceleratorTypes;
}
/**
* @return List of instance types to apply your specified attributes against. All other instance types are ignored, even if they match your specified attributes. You can use strings with one or more wild cards, represented by an asterisk (\*), to allow an instance type, size, or generation. The following are examples: `m5.8xlarge`, `c5*.*`, `m5a.*`, `r*`, `*3*`. For example, if you specify `c5*`, you are allowing the entire C5 instance family, which includes all C5a and C5n instance types. If you specify `m5a.*`, you are allowing all the M5a instance types, but not the M5n instance types. Maximum of 400 entries in the list; each entry is limited to 30 characters. Default is all instance types.
*
* > **NOTE:** If you specify `allowed_instance_types`, you can't specify `excluded_instance_types`.
*
*/
public List allowedInstanceTypes() {
return this.allowedInstanceTypes == null ? List.of() : this.allowedInstanceTypes;
}
/**
* @return Indicate whether bare metal instace types should be `included`, `excluded`, or `required`. Default is `excluded`.
*
*/
public Optional bareMetal() {
return Optional.ofNullable(this.bareMetal);
}
/**
* @return Block describing the minimum and maximum baseline EBS bandwidth, in Mbps. Default is no minimum or maximum.
*
*/
public Optional baselineEbsBandwidthMbps() {
return Optional.ofNullable(this.baselineEbsBandwidthMbps);
}
/**
* @return Indicate whether burstable performance instance types should be `included`, `excluded`, or `required`. Default is `excluded`.
*
*/
public Optional burstablePerformance() {
return Optional.ofNullable(this.burstablePerformance);
}
/**
* @return List of CPU manufacturer names. Default is any manufacturer.
*
* > **NOTE:** Don't confuse the CPU hardware manufacturer with the CPU hardware architecture. Instances will be launched with a compatible CPU architecture based on the Amazon Machine Image (AMI) that you specify in your launch template.
*
*/
public List cpuManufacturers() {
return this.cpuManufacturers == null ? List.of() : this.cpuManufacturers;
}
/**
* @return List of instance types to exclude. You can use strings with one or more wild cards, represented by an asterisk (\*), to exclude an instance type, size, or generation. The following are examples: `m5.8xlarge`, `c5*.*`, `m5a.*`, `r*`, `*3*`. For example, if you specify `c5*`, you are excluding the entire C5 instance family, which includes all C5a and C5n instance types. If you specify `m5a.*`, you are excluding all the M5a instance types, but not the M5n instance types. Maximum of 400 entries in the list; each entry is limited to 30 characters. Default is no excluded instance types.
*
* > **NOTE:** If you specify `excluded_instance_types`, you can't specify `allowed_instance_types`.
*
*/
public List excludedInstanceTypes() {
return this.excludedInstanceTypes == null ? List.of() : this.excludedInstanceTypes;
}
/**
* @return List of instance generation names. Default is any generation.
*
*/
public List instanceGenerations() {
return this.instanceGenerations == null ? List.of() : this.instanceGenerations;
}
/**
* @return Indicate whether instance types with local storage volumes are `included`, `excluded`, or `required`. Default is `included`.
*
*/
public Optional localStorage() {
return Optional.ofNullable(this.localStorage);
}
/**
* @return List of local storage type names. Default any storage type.
*
*/
public List localStorageTypes() {
return this.localStorageTypes == null ? List.of() : this.localStorageTypes;
}
/**
* @return The price protection threshold for Spot Instances. This is the maximum you’ll pay for a Spot Instance, expressed as a percentage higher than the cheapest M, C, or R instance type with your specified attributes. When Amazon EC2 Auto Scaling selects instance types with your attributes, we will exclude instance types whose price is higher than your threshold. The parameter accepts an integer, which Amazon EC2 Auto Scaling interprets as a percentage. To turn off price protection, specify a high value, such as 999999. Conflicts with `spot_max_price_percentage_over_lowest_price`
*
*/
public Optional maxSpotPriceAsPercentageOfOptimalOnDemandPrice() {
return Optional.ofNullable(this.maxSpotPriceAsPercentageOfOptimalOnDemandPrice);
}
/**
* @return Block describing the minimum and maximum amount of memory (GiB) per vCPU. Default is no minimum or maximum.
*
*/
public Optional memoryGibPerVcpu() {
return Optional.ofNullable(this.memoryGibPerVcpu);
}
/**
* @return Block describing the minimum and maximum amount of memory (MiB). Default is no maximum.
*
*/
public LaunchTemplateInstanceRequirementsMemoryMib memoryMib() {
return this.memoryMib;
}
/**
* @return Block describing the minimum and maximum amount of network bandwidth, in gigabits per second (Gbps). Default is no minimum or maximum.
*
*/
public Optional networkBandwidthGbps() {
return Optional.ofNullable(this.networkBandwidthGbps);
}
/**
* @return Block describing the minimum and maximum number of network interfaces. Default is no minimum or maximum.
*
*/
public Optional networkInterfaceCount() {
return Optional.ofNullable(this.networkInterfaceCount);
}
/**
* @return The price protection threshold for On-Demand Instances. This is the maximum you’ll pay for an On-Demand Instance, expressed as a percentage higher than the cheapest M, C, or R instance type with your specified attributes. When Amazon EC2 Auto Scaling selects instance types with your attributes, we will exclude instance types whose price is higher than your threshold. The parameter accepts an integer, which Amazon EC2 Auto Scaling interprets as a percentage. To turn off price protection, specify a high value, such as 999999. Default is 20.
*
* If you set DesiredCapacityType to vcpu or memory-mib, the price protection threshold is applied based on the per vCPU or per memory price instead of the per instance price.
*
*/
public Optional onDemandMaxPricePercentageOverLowestPrice() {
return Optional.ofNullable(this.onDemandMaxPricePercentageOverLowestPrice);
}
/**
* @return Indicate whether instance types must support On-Demand Instance Hibernation, either `true` or `false`. Default is `false`.
*
*/
public Optional requireHibernateSupport() {
return Optional.ofNullable(this.requireHibernateSupport);
}
/**
* @return The price protection threshold for Spot Instances. This is the maximum you’ll pay for a Spot Instance, expressed as a percentage higher than the cheapest M, C, or R instance type with your specified attributes. When Amazon EC2 Auto Scaling selects instance types with your attributes, we will exclude instance types whose price is higher than your threshold. The parameter accepts an integer, which Amazon EC2 Auto Scaling interprets as a percentage. To turn off price protection, specify a high value, such as 999999. Default is 100. Conflicts with `max_spot_price_as_percentage_of_optimal_on_demand_price`
*
* If you set DesiredCapacityType to vcpu or memory-mib, the price protection threshold is applied based on the per vCPU or per memory price instead of the per instance price.
*
*/
public Optional spotMaxPricePercentageOverLowestPrice() {
return Optional.ofNullable(this.spotMaxPricePercentageOverLowestPrice);
}
/**
* @return Block describing the minimum and maximum total local storage (GB). Default is no minimum or maximum.
*
*/
public Optional totalLocalStorageGb() {
return Optional.ofNullable(this.totalLocalStorageGb);
}
/**
* @return Block describing the minimum and maximum number of vCPUs. Default is no maximum.
*
*/
public LaunchTemplateInstanceRequirementsVcpuCount vcpuCount() {
return this.vcpuCount;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(LaunchTemplateInstanceRequirements defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable LaunchTemplateInstanceRequirementsAcceleratorCount acceleratorCount;
private @Nullable List acceleratorManufacturers;
private @Nullable List acceleratorNames;
private @Nullable LaunchTemplateInstanceRequirementsAcceleratorTotalMemoryMib acceleratorTotalMemoryMib;
private @Nullable List acceleratorTypes;
private @Nullable List allowedInstanceTypes;
private @Nullable String bareMetal;
private @Nullable LaunchTemplateInstanceRequirementsBaselineEbsBandwidthMbps baselineEbsBandwidthMbps;
private @Nullable String burstablePerformance;
private @Nullable List cpuManufacturers;
private @Nullable List excludedInstanceTypes;
private @Nullable List instanceGenerations;
private @Nullable String localStorage;
private @Nullable List localStorageTypes;
private @Nullable Integer maxSpotPriceAsPercentageOfOptimalOnDemandPrice;
private @Nullable LaunchTemplateInstanceRequirementsMemoryGibPerVcpu memoryGibPerVcpu;
private LaunchTemplateInstanceRequirementsMemoryMib memoryMib;
private @Nullable LaunchTemplateInstanceRequirementsNetworkBandwidthGbps networkBandwidthGbps;
private @Nullable LaunchTemplateInstanceRequirementsNetworkInterfaceCount networkInterfaceCount;
private @Nullable Integer onDemandMaxPricePercentageOverLowestPrice;
private @Nullable Boolean requireHibernateSupport;
private @Nullable Integer spotMaxPricePercentageOverLowestPrice;
private @Nullable LaunchTemplateInstanceRequirementsTotalLocalStorageGb totalLocalStorageGb;
private LaunchTemplateInstanceRequirementsVcpuCount vcpuCount;
public Builder() {}
public Builder(LaunchTemplateInstanceRequirements defaults) {
Objects.requireNonNull(defaults);
this.acceleratorCount = defaults.acceleratorCount;
this.acceleratorManufacturers = defaults.acceleratorManufacturers;
this.acceleratorNames = defaults.acceleratorNames;
this.acceleratorTotalMemoryMib = defaults.acceleratorTotalMemoryMib;
this.acceleratorTypes = defaults.acceleratorTypes;
this.allowedInstanceTypes = defaults.allowedInstanceTypes;
this.bareMetal = defaults.bareMetal;
this.baselineEbsBandwidthMbps = defaults.baselineEbsBandwidthMbps;
this.burstablePerformance = defaults.burstablePerformance;
this.cpuManufacturers = defaults.cpuManufacturers;
this.excludedInstanceTypes = defaults.excludedInstanceTypes;
this.instanceGenerations = defaults.instanceGenerations;
this.localStorage = defaults.localStorage;
this.localStorageTypes = defaults.localStorageTypes;
this.maxSpotPriceAsPercentageOfOptimalOnDemandPrice = defaults.maxSpotPriceAsPercentageOfOptimalOnDemandPrice;
this.memoryGibPerVcpu = defaults.memoryGibPerVcpu;
this.memoryMib = defaults.memoryMib;
this.networkBandwidthGbps = defaults.networkBandwidthGbps;
this.networkInterfaceCount = defaults.networkInterfaceCount;
this.onDemandMaxPricePercentageOverLowestPrice = defaults.onDemandMaxPricePercentageOverLowestPrice;
this.requireHibernateSupport = defaults.requireHibernateSupport;
this.spotMaxPricePercentageOverLowestPrice = defaults.spotMaxPricePercentageOverLowestPrice;
this.totalLocalStorageGb = defaults.totalLocalStorageGb;
this.vcpuCount = defaults.vcpuCount;
}
@CustomType.Setter
public Builder acceleratorCount(@Nullable LaunchTemplateInstanceRequirementsAcceleratorCount acceleratorCount) {
this.acceleratorCount = acceleratorCount;
return this;
}
@CustomType.Setter
public Builder acceleratorManufacturers(@Nullable List acceleratorManufacturers) {
this.acceleratorManufacturers = acceleratorManufacturers;
return this;
}
public Builder acceleratorManufacturers(String... acceleratorManufacturers) {
return acceleratorManufacturers(List.of(acceleratorManufacturers));
}
@CustomType.Setter
public Builder acceleratorNames(@Nullable List acceleratorNames) {
this.acceleratorNames = acceleratorNames;
return this;
}
public Builder acceleratorNames(String... acceleratorNames) {
return acceleratorNames(List.of(acceleratorNames));
}
@CustomType.Setter
public Builder acceleratorTotalMemoryMib(@Nullable LaunchTemplateInstanceRequirementsAcceleratorTotalMemoryMib acceleratorTotalMemoryMib) {
this.acceleratorTotalMemoryMib = acceleratorTotalMemoryMib;
return this;
}
@CustomType.Setter
public Builder acceleratorTypes(@Nullable List acceleratorTypes) {
this.acceleratorTypes = acceleratorTypes;
return this;
}
public Builder acceleratorTypes(String... acceleratorTypes) {
return acceleratorTypes(List.of(acceleratorTypes));
}
@CustomType.Setter
public Builder allowedInstanceTypes(@Nullable List allowedInstanceTypes) {
this.allowedInstanceTypes = allowedInstanceTypes;
return this;
}
public Builder allowedInstanceTypes(String... allowedInstanceTypes) {
return allowedInstanceTypes(List.of(allowedInstanceTypes));
}
@CustomType.Setter
public Builder bareMetal(@Nullable String bareMetal) {
this.bareMetal = bareMetal;
return this;
}
@CustomType.Setter
public Builder baselineEbsBandwidthMbps(@Nullable LaunchTemplateInstanceRequirementsBaselineEbsBandwidthMbps baselineEbsBandwidthMbps) {
this.baselineEbsBandwidthMbps = baselineEbsBandwidthMbps;
return this;
}
@CustomType.Setter
public Builder burstablePerformance(@Nullable String burstablePerformance) {
this.burstablePerformance = burstablePerformance;
return this;
}
@CustomType.Setter
public Builder cpuManufacturers(@Nullable List cpuManufacturers) {
this.cpuManufacturers = cpuManufacturers;
return this;
}
public Builder cpuManufacturers(String... cpuManufacturers) {
return cpuManufacturers(List.of(cpuManufacturers));
}
@CustomType.Setter
public Builder excludedInstanceTypes(@Nullable List excludedInstanceTypes) {
this.excludedInstanceTypes = excludedInstanceTypes;
return this;
}
public Builder excludedInstanceTypes(String... excludedInstanceTypes) {
return excludedInstanceTypes(List.of(excludedInstanceTypes));
}
@CustomType.Setter
public Builder instanceGenerations(@Nullable List instanceGenerations) {
this.instanceGenerations = instanceGenerations;
return this;
}
public Builder instanceGenerations(String... instanceGenerations) {
return instanceGenerations(List.of(instanceGenerations));
}
@CustomType.Setter
public Builder localStorage(@Nullable String localStorage) {
this.localStorage = localStorage;
return this;
}
@CustomType.Setter
public Builder localStorageTypes(@Nullable List localStorageTypes) {
this.localStorageTypes = localStorageTypes;
return this;
}
public Builder localStorageTypes(String... localStorageTypes) {
return localStorageTypes(List.of(localStorageTypes));
}
@CustomType.Setter
public Builder maxSpotPriceAsPercentageOfOptimalOnDemandPrice(@Nullable Integer maxSpotPriceAsPercentageOfOptimalOnDemandPrice) {
this.maxSpotPriceAsPercentageOfOptimalOnDemandPrice = maxSpotPriceAsPercentageOfOptimalOnDemandPrice;
return this;
}
@CustomType.Setter
public Builder memoryGibPerVcpu(@Nullable LaunchTemplateInstanceRequirementsMemoryGibPerVcpu memoryGibPerVcpu) {
this.memoryGibPerVcpu = memoryGibPerVcpu;
return this;
}
@CustomType.Setter
public Builder memoryMib(LaunchTemplateInstanceRequirementsMemoryMib memoryMib) {
if (memoryMib == null) {
throw new MissingRequiredPropertyException("LaunchTemplateInstanceRequirements", "memoryMib");
}
this.memoryMib = memoryMib;
return this;
}
@CustomType.Setter
public Builder networkBandwidthGbps(@Nullable LaunchTemplateInstanceRequirementsNetworkBandwidthGbps networkBandwidthGbps) {
this.networkBandwidthGbps = networkBandwidthGbps;
return this;
}
@CustomType.Setter
public Builder networkInterfaceCount(@Nullable LaunchTemplateInstanceRequirementsNetworkInterfaceCount networkInterfaceCount) {
this.networkInterfaceCount = networkInterfaceCount;
return this;
}
@CustomType.Setter
public Builder onDemandMaxPricePercentageOverLowestPrice(@Nullable Integer onDemandMaxPricePercentageOverLowestPrice) {
this.onDemandMaxPricePercentageOverLowestPrice = onDemandMaxPricePercentageOverLowestPrice;
return this;
}
@CustomType.Setter
public Builder requireHibernateSupport(@Nullable Boolean requireHibernateSupport) {
this.requireHibernateSupport = requireHibernateSupport;
return this;
}
@CustomType.Setter
public Builder spotMaxPricePercentageOverLowestPrice(@Nullable Integer spotMaxPricePercentageOverLowestPrice) {
this.spotMaxPricePercentageOverLowestPrice = spotMaxPricePercentageOverLowestPrice;
return this;
}
@CustomType.Setter
public Builder totalLocalStorageGb(@Nullable LaunchTemplateInstanceRequirementsTotalLocalStorageGb totalLocalStorageGb) {
this.totalLocalStorageGb = totalLocalStorageGb;
return this;
}
@CustomType.Setter
public Builder vcpuCount(LaunchTemplateInstanceRequirementsVcpuCount vcpuCount) {
if (vcpuCount == null) {
throw new MissingRequiredPropertyException("LaunchTemplateInstanceRequirements", "vcpuCount");
}
this.vcpuCount = vcpuCount;
return this;
}
public LaunchTemplateInstanceRequirements build() {
final var _resultValue = new LaunchTemplateInstanceRequirements();
_resultValue.acceleratorCount = acceleratorCount;
_resultValue.acceleratorManufacturers = acceleratorManufacturers;
_resultValue.acceleratorNames = acceleratorNames;
_resultValue.acceleratorTotalMemoryMib = acceleratorTotalMemoryMib;
_resultValue.acceleratorTypes = acceleratorTypes;
_resultValue.allowedInstanceTypes = allowedInstanceTypes;
_resultValue.bareMetal = bareMetal;
_resultValue.baselineEbsBandwidthMbps = baselineEbsBandwidthMbps;
_resultValue.burstablePerformance = burstablePerformance;
_resultValue.cpuManufacturers = cpuManufacturers;
_resultValue.excludedInstanceTypes = excludedInstanceTypes;
_resultValue.instanceGenerations = instanceGenerations;
_resultValue.localStorage = localStorage;
_resultValue.localStorageTypes = localStorageTypes;
_resultValue.maxSpotPriceAsPercentageOfOptimalOnDemandPrice = maxSpotPriceAsPercentageOfOptimalOnDemandPrice;
_resultValue.memoryGibPerVcpu = memoryGibPerVcpu;
_resultValue.memoryMib = memoryMib;
_resultValue.networkBandwidthGbps = networkBandwidthGbps;
_resultValue.networkInterfaceCount = networkInterfaceCount;
_resultValue.onDemandMaxPricePercentageOverLowestPrice = onDemandMaxPricePercentageOverLowestPrice;
_resultValue.requireHibernateSupport = requireHibernateSupport;
_resultValue.spotMaxPricePercentageOverLowestPrice = spotMaxPricePercentageOverLowestPrice;
_resultValue.totalLocalStorageGb = totalLocalStorageGb;
_resultValue.vcpuCount = vcpuCount;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy