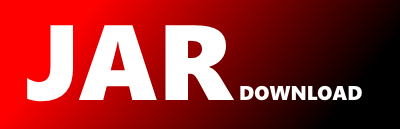
com.pulumi.aws.ec2.outputs.SecurityGroupEgress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SecurityGroupEgress {
/**
* @return List of CIDR blocks.
*
*/
private @Nullable List cidrBlocks;
/**
* @return Description of this egress rule.
*
*/
private @Nullable String description;
/**
* @return Start port (or ICMP type number if protocol is `icmp`)
*
*/
private Integer fromPort;
/**
* @return List of IPv6 CIDR blocks.
*
*/
private @Nullable List ipv6CidrBlocks;
/**
* @return List of Prefix List IDs.
*
*/
private @Nullable List prefixListIds;
/**
* @return Protocol. If you select a protocol of `-1` (semantically equivalent to `all`, which is not a valid value here), you must specify a `from_port` and `to_port` equal to 0. The supported values are defined in the `IpProtocol` argument in the [IpPermission](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_IpPermission.html) API reference.
*
*/
private String protocol;
/**
* @return List of security groups. A group name can be used relative to the default VPC. Otherwise, group ID.
*
*/
private @Nullable List securityGroups;
/**
* @return Whether the security group itself will be added as a source to this egress rule.
*
*/
private @Nullable Boolean self;
/**
* @return End range port (or ICMP code if protocol is `icmp`).
*
* The following arguments are optional:
*
* > **Note** Although `cidr_blocks`, `ipv6_cidr_blocks`, `prefix_list_ids`, and `security_groups` are all marked as optional, you _must_ provide one of them in order to configure the destination of the traffic.
*
*/
private Integer toPort;
private SecurityGroupEgress() {}
/**
* @return List of CIDR blocks.
*
*/
public List cidrBlocks() {
return this.cidrBlocks == null ? List.of() : this.cidrBlocks;
}
/**
* @return Description of this egress rule.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Start port (or ICMP type number if protocol is `icmp`)
*
*/
public Integer fromPort() {
return this.fromPort;
}
/**
* @return List of IPv6 CIDR blocks.
*
*/
public List ipv6CidrBlocks() {
return this.ipv6CidrBlocks == null ? List.of() : this.ipv6CidrBlocks;
}
/**
* @return List of Prefix List IDs.
*
*/
public List prefixListIds() {
return this.prefixListIds == null ? List.of() : this.prefixListIds;
}
/**
* @return Protocol. If you select a protocol of `-1` (semantically equivalent to `all`, which is not a valid value here), you must specify a `from_port` and `to_port` equal to 0. The supported values are defined in the `IpProtocol` argument in the [IpPermission](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_IpPermission.html) API reference.
*
*/
public String protocol() {
return this.protocol;
}
/**
* @return List of security groups. A group name can be used relative to the default VPC. Otherwise, group ID.
*
*/
public List securityGroups() {
return this.securityGroups == null ? List.of() : this.securityGroups;
}
/**
* @return Whether the security group itself will be added as a source to this egress rule.
*
*/
public Optional self() {
return Optional.ofNullable(this.self);
}
/**
* @return End range port (or ICMP code if protocol is `icmp`).
*
* The following arguments are optional:
*
* > **Note** Although `cidr_blocks`, `ipv6_cidr_blocks`, `prefix_list_ids`, and `security_groups` are all marked as optional, you _must_ provide one of them in order to configure the destination of the traffic.
*
*/
public Integer toPort() {
return this.toPort;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SecurityGroupEgress defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List cidrBlocks;
private @Nullable String description;
private Integer fromPort;
private @Nullable List ipv6CidrBlocks;
private @Nullable List prefixListIds;
private String protocol;
private @Nullable List securityGroups;
private @Nullable Boolean self;
private Integer toPort;
public Builder() {}
public Builder(SecurityGroupEgress defaults) {
Objects.requireNonNull(defaults);
this.cidrBlocks = defaults.cidrBlocks;
this.description = defaults.description;
this.fromPort = defaults.fromPort;
this.ipv6CidrBlocks = defaults.ipv6CidrBlocks;
this.prefixListIds = defaults.prefixListIds;
this.protocol = defaults.protocol;
this.securityGroups = defaults.securityGroups;
this.self = defaults.self;
this.toPort = defaults.toPort;
}
@CustomType.Setter
public Builder cidrBlocks(@Nullable List cidrBlocks) {
this.cidrBlocks = cidrBlocks;
return this;
}
public Builder cidrBlocks(String... cidrBlocks) {
return cidrBlocks(List.of(cidrBlocks));
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder fromPort(Integer fromPort) {
if (fromPort == null) {
throw new MissingRequiredPropertyException("SecurityGroupEgress", "fromPort");
}
this.fromPort = fromPort;
return this;
}
@CustomType.Setter
public Builder ipv6CidrBlocks(@Nullable List ipv6CidrBlocks) {
this.ipv6CidrBlocks = ipv6CidrBlocks;
return this;
}
public Builder ipv6CidrBlocks(String... ipv6CidrBlocks) {
return ipv6CidrBlocks(List.of(ipv6CidrBlocks));
}
@CustomType.Setter
public Builder prefixListIds(@Nullable List prefixListIds) {
this.prefixListIds = prefixListIds;
return this;
}
public Builder prefixListIds(String... prefixListIds) {
return prefixListIds(List.of(prefixListIds));
}
@CustomType.Setter
public Builder protocol(String protocol) {
if (protocol == null) {
throw new MissingRequiredPropertyException("SecurityGroupEgress", "protocol");
}
this.protocol = protocol;
return this;
}
@CustomType.Setter
public Builder securityGroups(@Nullable List securityGroups) {
this.securityGroups = securityGroups;
return this;
}
public Builder securityGroups(String... securityGroups) {
return securityGroups(List.of(securityGroups));
}
@CustomType.Setter
public Builder self(@Nullable Boolean self) {
this.self = self;
return this;
}
@CustomType.Setter
public Builder toPort(Integer toPort) {
if (toPort == null) {
throw new MissingRequiredPropertyException("SecurityGroupEgress", "toPort");
}
this.toPort = toPort;
return this;
}
public SecurityGroupEgress build() {
final var _resultValue = new SecurityGroupEgress();
_resultValue.cidrBlocks = cidrBlocks;
_resultValue.description = description;
_resultValue.fromPort = fromPort;
_resultValue.ipv6CidrBlocks = ipv6CidrBlocks;
_resultValue.prefixListIds = prefixListIds;
_resultValue.protocol = protocol;
_resultValue.securityGroups = securityGroups;
_resultValue.self = self;
_resultValue.toPort = toPort;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy