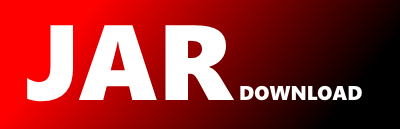
com.pulumi.aws.ec2.outputs.SpotInstanceRequestNetworkInterface Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SpotInstanceRequestNetworkInterface {
/**
* @return Whether or not to delete the network interface on instance termination. Defaults to `false`. Currently, the only valid value is `false`, as this is only supported when creating new network interfaces when launching an instance.
*
*/
private @Nullable Boolean deleteOnTermination;
/**
* @return Integer index of the network interface attachment. Limited by instance type.
*
*/
private Integer deviceIndex;
/**
* @return Integer index of the network card. Limited by instance type. The default index is `0`.
*
*/
private @Nullable Integer networkCardIndex;
/**
* @return ID of the network interface to attach.
*
*/
private String networkInterfaceId;
private SpotInstanceRequestNetworkInterface() {}
/**
* @return Whether or not to delete the network interface on instance termination. Defaults to `false`. Currently, the only valid value is `false`, as this is only supported when creating new network interfaces when launching an instance.
*
*/
public Optional deleteOnTermination() {
return Optional.ofNullable(this.deleteOnTermination);
}
/**
* @return Integer index of the network interface attachment. Limited by instance type.
*
*/
public Integer deviceIndex() {
return this.deviceIndex;
}
/**
* @return Integer index of the network card. Limited by instance type. The default index is `0`.
*
*/
public Optional networkCardIndex() {
return Optional.ofNullable(this.networkCardIndex);
}
/**
* @return ID of the network interface to attach.
*
*/
public String networkInterfaceId() {
return this.networkInterfaceId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SpotInstanceRequestNetworkInterface defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean deleteOnTermination;
private Integer deviceIndex;
private @Nullable Integer networkCardIndex;
private String networkInterfaceId;
public Builder() {}
public Builder(SpotInstanceRequestNetworkInterface defaults) {
Objects.requireNonNull(defaults);
this.deleteOnTermination = defaults.deleteOnTermination;
this.deviceIndex = defaults.deviceIndex;
this.networkCardIndex = defaults.networkCardIndex;
this.networkInterfaceId = defaults.networkInterfaceId;
}
@CustomType.Setter
public Builder deleteOnTermination(@Nullable Boolean deleteOnTermination) {
this.deleteOnTermination = deleteOnTermination;
return this;
}
@CustomType.Setter
public Builder deviceIndex(Integer deviceIndex) {
if (deviceIndex == null) {
throw new MissingRequiredPropertyException("SpotInstanceRequestNetworkInterface", "deviceIndex");
}
this.deviceIndex = deviceIndex;
return this;
}
@CustomType.Setter
public Builder networkCardIndex(@Nullable Integer networkCardIndex) {
this.networkCardIndex = networkCardIndex;
return this;
}
@CustomType.Setter
public Builder networkInterfaceId(String networkInterfaceId) {
if (networkInterfaceId == null) {
throw new MissingRequiredPropertyException("SpotInstanceRequestNetworkInterface", "networkInterfaceId");
}
this.networkInterfaceId = networkInterfaceId;
return this;
}
public SpotInstanceRequestNetworkInterface build() {
final var _resultValue = new SpotInstanceRequestNetworkInterface();
_resultValue.deleteOnTermination = deleteOnTermination;
_resultValue.deviceIndex = deviceIndex;
_resultValue.networkCardIndex = networkCardIndex;
_resultValue.networkInterfaceId = networkInterfaceId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy