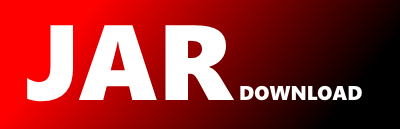
com.pulumi.aws.ec2transitgateway.outputs.GetMulticastDomainResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2transitgateway.outputs;
import com.pulumi.aws.ec2transitgateway.outputs.GetMulticastDomainAssociation;
import com.pulumi.aws.ec2transitgateway.outputs.GetMulticastDomainFilter;
import com.pulumi.aws.ec2transitgateway.outputs.GetMulticastDomainMember;
import com.pulumi.aws.ec2transitgateway.outputs.GetMulticastDomainSource;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class GetMulticastDomainResult {
/**
* @return EC2 Transit Gateway Multicast Domain ARN.
*
*/
private String arn;
/**
* @return EC2 Transit Gateway Multicast Domain Associations
*
*/
private List associations;
/**
* @return Whether to automatically accept cross-account subnet associations that are associated with the EC2 Transit Gateway Multicast Domain.
*
*/
private String autoAcceptSharedAssociations;
private @Nullable List filters;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Whether to enable Internet Group Management Protocol (IGMP) version 2 for the EC2 Transit Gateway Multicast Domain.
*
*/
private String igmpv2Support;
/**
* @return EC2 Multicast Domain Group Members
*
*/
private List members;
/**
* @return Identifier of the AWS account that owns the EC2 Transit Gateway Multicast Domain.
*
*/
private String ownerId;
/**
* @return EC2 Multicast Domain Group Sources
*
*/
private List sources;
private String state;
/**
* @return Whether to enable support for statically configuring multicast group sources for the EC2 Transit Gateway Multicast Domain.
*
*/
private String staticSourcesSupport;
/**
* @return Key-value tags for the EC2 Transit Gateway Multicast Domain.
*
*/
private Map tags;
/**
* @return The ID of the transit gateway attachment.
*
*/
private String transitGatewayAttachmentId;
/**
* @return EC2 Transit Gateway identifier.
*
*/
private String transitGatewayId;
private String transitGatewayMulticastDomainId;
private GetMulticastDomainResult() {}
/**
* @return EC2 Transit Gateway Multicast Domain ARN.
*
*/
public String arn() {
return this.arn;
}
/**
* @return EC2 Transit Gateway Multicast Domain Associations
*
*/
public List associations() {
return this.associations;
}
/**
* @return Whether to automatically accept cross-account subnet associations that are associated with the EC2 Transit Gateway Multicast Domain.
*
*/
public String autoAcceptSharedAssociations() {
return this.autoAcceptSharedAssociations;
}
public List filters() {
return this.filters == null ? List.of() : this.filters;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Whether to enable Internet Group Management Protocol (IGMP) version 2 for the EC2 Transit Gateway Multicast Domain.
*
*/
public String igmpv2Support() {
return this.igmpv2Support;
}
/**
* @return EC2 Multicast Domain Group Members
*
*/
public List members() {
return this.members;
}
/**
* @return Identifier of the AWS account that owns the EC2 Transit Gateway Multicast Domain.
*
*/
public String ownerId() {
return this.ownerId;
}
/**
* @return EC2 Multicast Domain Group Sources
*
*/
public List sources() {
return this.sources;
}
public String state() {
return this.state;
}
/**
* @return Whether to enable support for statically configuring multicast group sources for the EC2 Transit Gateway Multicast Domain.
*
*/
public String staticSourcesSupport() {
return this.staticSourcesSupport;
}
/**
* @return Key-value tags for the EC2 Transit Gateway Multicast Domain.
*
*/
public Map tags() {
return this.tags;
}
/**
* @return The ID of the transit gateway attachment.
*
*/
public String transitGatewayAttachmentId() {
return this.transitGatewayAttachmentId;
}
/**
* @return EC2 Transit Gateway identifier.
*
*/
public String transitGatewayId() {
return this.transitGatewayId;
}
public String transitGatewayMulticastDomainId() {
return this.transitGatewayMulticastDomainId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetMulticastDomainResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private List associations;
private String autoAcceptSharedAssociations;
private @Nullable List filters;
private String id;
private String igmpv2Support;
private List members;
private String ownerId;
private List sources;
private String state;
private String staticSourcesSupport;
private Map tags;
private String transitGatewayAttachmentId;
private String transitGatewayId;
private String transitGatewayMulticastDomainId;
public Builder() {}
public Builder(GetMulticastDomainResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.associations = defaults.associations;
this.autoAcceptSharedAssociations = defaults.autoAcceptSharedAssociations;
this.filters = defaults.filters;
this.id = defaults.id;
this.igmpv2Support = defaults.igmpv2Support;
this.members = defaults.members;
this.ownerId = defaults.ownerId;
this.sources = defaults.sources;
this.state = defaults.state;
this.staticSourcesSupport = defaults.staticSourcesSupport;
this.tags = defaults.tags;
this.transitGatewayAttachmentId = defaults.transitGatewayAttachmentId;
this.transitGatewayId = defaults.transitGatewayId;
this.transitGatewayMulticastDomainId = defaults.transitGatewayMulticastDomainId;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder associations(List associations) {
if (associations == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "associations");
}
this.associations = associations;
return this;
}
public Builder associations(GetMulticastDomainAssociation... associations) {
return associations(List.of(associations));
}
@CustomType.Setter
public Builder autoAcceptSharedAssociations(String autoAcceptSharedAssociations) {
if (autoAcceptSharedAssociations == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "autoAcceptSharedAssociations");
}
this.autoAcceptSharedAssociations = autoAcceptSharedAssociations;
return this;
}
@CustomType.Setter
public Builder filters(@Nullable List filters) {
this.filters = filters;
return this;
}
public Builder filters(GetMulticastDomainFilter... filters) {
return filters(List.of(filters));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder igmpv2Support(String igmpv2Support) {
if (igmpv2Support == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "igmpv2Support");
}
this.igmpv2Support = igmpv2Support;
return this;
}
@CustomType.Setter
public Builder members(List members) {
if (members == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "members");
}
this.members = members;
return this;
}
public Builder members(GetMulticastDomainMember... members) {
return members(List.of(members));
}
@CustomType.Setter
public Builder ownerId(String ownerId) {
if (ownerId == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "ownerId");
}
this.ownerId = ownerId;
return this;
}
@CustomType.Setter
public Builder sources(List sources) {
if (sources == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "sources");
}
this.sources = sources;
return this;
}
public Builder sources(GetMulticastDomainSource... sources) {
return sources(List.of(sources));
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder staticSourcesSupport(String staticSourcesSupport) {
if (staticSourcesSupport == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "staticSourcesSupport");
}
this.staticSourcesSupport = staticSourcesSupport;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder transitGatewayAttachmentId(String transitGatewayAttachmentId) {
if (transitGatewayAttachmentId == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "transitGatewayAttachmentId");
}
this.transitGatewayAttachmentId = transitGatewayAttachmentId;
return this;
}
@CustomType.Setter
public Builder transitGatewayId(String transitGatewayId) {
if (transitGatewayId == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "transitGatewayId");
}
this.transitGatewayId = transitGatewayId;
return this;
}
@CustomType.Setter
public Builder transitGatewayMulticastDomainId(String transitGatewayMulticastDomainId) {
if (transitGatewayMulticastDomainId == null) {
throw new MissingRequiredPropertyException("GetMulticastDomainResult", "transitGatewayMulticastDomainId");
}
this.transitGatewayMulticastDomainId = transitGatewayMulticastDomainId;
return this;
}
public GetMulticastDomainResult build() {
final var _resultValue = new GetMulticastDomainResult();
_resultValue.arn = arn;
_resultValue.associations = associations;
_resultValue.autoAcceptSharedAssociations = autoAcceptSharedAssociations;
_resultValue.filters = filters;
_resultValue.id = id;
_resultValue.igmpv2Support = igmpv2Support;
_resultValue.members = members;
_resultValue.ownerId = ownerId;
_resultValue.sources = sources;
_resultValue.state = state;
_resultValue.staticSourcesSupport = staticSourcesSupport;
_resultValue.tags = tags;
_resultValue.transitGatewayAttachmentId = transitGatewayAttachmentId;
_resultValue.transitGatewayId = transitGatewayId;
_resultValue.transitGatewayMulticastDomainId = transitGatewayMulticastDomainId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy