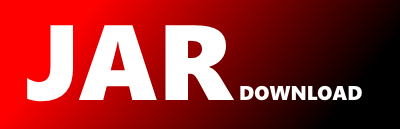
com.pulumi.aws.ecs.outputs.GetContainerDefinitionResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ecs.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetContainerDefinitionResult {
private String containerName;
/**
* @return CPU limit for this container definition
*
*/
private Integer cpu;
/**
* @return Indicator if networking is disabled
*
*/
private Boolean disableNetworking;
/**
* @return Set docker labels
*
*/
private Map dockerLabels;
/**
* @return Environment in use
*
*/
private Map environment;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Docker image in use, including the digest
*
*/
private String image;
/**
* @return Digest of the docker image in use
*
*/
private String imageDigest;
/**
* @return Memory limit for this container definition
*
*/
private Integer memory;
/**
* @return Soft limit (in MiB) of memory to reserve for the container. When system memory is under contention, Docker attempts to keep the container memory to this soft limit
*
*/
private Integer memoryReservation;
private String taskDefinition;
private GetContainerDefinitionResult() {}
public String containerName() {
return this.containerName;
}
/**
* @return CPU limit for this container definition
*
*/
public Integer cpu() {
return this.cpu;
}
/**
* @return Indicator if networking is disabled
*
*/
public Boolean disableNetworking() {
return this.disableNetworking;
}
/**
* @return Set docker labels
*
*/
public Map dockerLabels() {
return this.dockerLabels;
}
/**
* @return Environment in use
*
*/
public Map environment() {
return this.environment;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Docker image in use, including the digest
*
*/
public String image() {
return this.image;
}
/**
* @return Digest of the docker image in use
*
*/
public String imageDigest() {
return this.imageDigest;
}
/**
* @return Memory limit for this container definition
*
*/
public Integer memory() {
return this.memory;
}
/**
* @return Soft limit (in MiB) of memory to reserve for the container. When system memory is under contention, Docker attempts to keep the container memory to this soft limit
*
*/
public Integer memoryReservation() {
return this.memoryReservation;
}
public String taskDefinition() {
return this.taskDefinition;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetContainerDefinitionResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String containerName;
private Integer cpu;
private Boolean disableNetworking;
private Map dockerLabels;
private Map environment;
private String id;
private String image;
private String imageDigest;
private Integer memory;
private Integer memoryReservation;
private String taskDefinition;
public Builder() {}
public Builder(GetContainerDefinitionResult defaults) {
Objects.requireNonNull(defaults);
this.containerName = defaults.containerName;
this.cpu = defaults.cpu;
this.disableNetworking = defaults.disableNetworking;
this.dockerLabels = defaults.dockerLabels;
this.environment = defaults.environment;
this.id = defaults.id;
this.image = defaults.image;
this.imageDigest = defaults.imageDigest;
this.memory = defaults.memory;
this.memoryReservation = defaults.memoryReservation;
this.taskDefinition = defaults.taskDefinition;
}
@CustomType.Setter
public Builder containerName(String containerName) {
if (containerName == null) {
throw new MissingRequiredPropertyException("GetContainerDefinitionResult", "containerName");
}
this.containerName = containerName;
return this;
}
@CustomType.Setter
public Builder cpu(Integer cpu) {
if (cpu == null) {
throw new MissingRequiredPropertyException("GetContainerDefinitionResult", "cpu");
}
this.cpu = cpu;
return this;
}
@CustomType.Setter
public Builder disableNetworking(Boolean disableNetworking) {
if (disableNetworking == null) {
throw new MissingRequiredPropertyException("GetContainerDefinitionResult", "disableNetworking");
}
this.disableNetworking = disableNetworking;
return this;
}
@CustomType.Setter
public Builder dockerLabels(Map dockerLabels) {
if (dockerLabels == null) {
throw new MissingRequiredPropertyException("GetContainerDefinitionResult", "dockerLabels");
}
this.dockerLabels = dockerLabels;
return this;
}
@CustomType.Setter
public Builder environment(Map environment) {
if (environment == null) {
throw new MissingRequiredPropertyException("GetContainerDefinitionResult", "environment");
}
this.environment = environment;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetContainerDefinitionResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder image(String image) {
if (image == null) {
throw new MissingRequiredPropertyException("GetContainerDefinitionResult", "image");
}
this.image = image;
return this;
}
@CustomType.Setter
public Builder imageDigest(String imageDigest) {
if (imageDigest == null) {
throw new MissingRequiredPropertyException("GetContainerDefinitionResult", "imageDigest");
}
this.imageDigest = imageDigest;
return this;
}
@CustomType.Setter
public Builder memory(Integer memory) {
if (memory == null) {
throw new MissingRequiredPropertyException("GetContainerDefinitionResult", "memory");
}
this.memory = memory;
return this;
}
@CustomType.Setter
public Builder memoryReservation(Integer memoryReservation) {
if (memoryReservation == null) {
throw new MissingRequiredPropertyException("GetContainerDefinitionResult", "memoryReservation");
}
this.memoryReservation = memoryReservation;
return this;
}
@CustomType.Setter
public Builder taskDefinition(String taskDefinition) {
if (taskDefinition == null) {
throw new MissingRequiredPropertyException("GetContainerDefinitionResult", "taskDefinition");
}
this.taskDefinition = taskDefinition;
return this;
}
public GetContainerDefinitionResult build() {
final var _resultValue = new GetContainerDefinitionResult();
_resultValue.containerName = containerName;
_resultValue.cpu = cpu;
_resultValue.disableNetworking = disableNetworking;
_resultValue.dockerLabels = dockerLabels;
_resultValue.environment = environment;
_resultValue.id = id;
_resultValue.image = image;
_resultValue.imageDigest = imageDigest;
_resultValue.memory = memory;
_resultValue.memoryReservation = memoryReservation;
_resultValue.taskDefinition = taskDefinition;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy