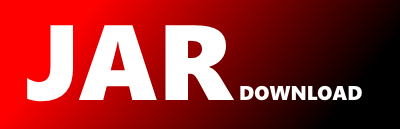
com.pulumi.aws.eks.outputs.GetAddonResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.eks.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetAddonResult {
private String addonName;
/**
* @return Version of EKS add-on.
*
*/
private String addonVersion;
/**
* @return ARN of the EKS add-on.
*
*/
private String arn;
private String clusterName;
/**
* @return Configuration values for the addon with a single JSON string.
*
*/
private String configurationValues;
/**
* @return Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the EKS add-on was created.
*
*/
private String createdAt;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the EKS add-on was updated.
*
*/
private String modifiedAt;
/**
* @return ARN of IAM role used for EKS add-on. If value is empty -
* then add-on uses the IAM role assigned to the EKS Cluster node.
*
*/
private String serviceAccountRoleArn;
private Map tags;
private GetAddonResult() {}
public String addonName() {
return this.addonName;
}
/**
* @return Version of EKS add-on.
*
*/
public String addonVersion() {
return this.addonVersion;
}
/**
* @return ARN of the EKS add-on.
*
*/
public String arn() {
return this.arn;
}
public String clusterName() {
return this.clusterName;
}
/**
* @return Configuration values for the addon with a single JSON string.
*
*/
public String configurationValues() {
return this.configurationValues;
}
/**
* @return Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the EKS add-on was created.
*
*/
public String createdAt() {
return this.createdAt;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the EKS add-on was updated.
*
*/
public String modifiedAt() {
return this.modifiedAt;
}
/**
* @return ARN of IAM role used for EKS add-on. If value is empty -
* then add-on uses the IAM role assigned to the EKS Cluster node.
*
*/
public String serviceAccountRoleArn() {
return this.serviceAccountRoleArn;
}
public Map tags() {
return this.tags;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAddonResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String addonName;
private String addonVersion;
private String arn;
private String clusterName;
private String configurationValues;
private String createdAt;
private String id;
private String modifiedAt;
private String serviceAccountRoleArn;
private Map tags;
public Builder() {}
public Builder(GetAddonResult defaults) {
Objects.requireNonNull(defaults);
this.addonName = defaults.addonName;
this.addonVersion = defaults.addonVersion;
this.arn = defaults.arn;
this.clusterName = defaults.clusterName;
this.configurationValues = defaults.configurationValues;
this.createdAt = defaults.createdAt;
this.id = defaults.id;
this.modifiedAt = defaults.modifiedAt;
this.serviceAccountRoleArn = defaults.serviceAccountRoleArn;
this.tags = defaults.tags;
}
@CustomType.Setter
public Builder addonName(String addonName) {
if (addonName == null) {
throw new MissingRequiredPropertyException("GetAddonResult", "addonName");
}
this.addonName = addonName;
return this;
}
@CustomType.Setter
public Builder addonVersion(String addonVersion) {
if (addonVersion == null) {
throw new MissingRequiredPropertyException("GetAddonResult", "addonVersion");
}
this.addonVersion = addonVersion;
return this;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetAddonResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder clusterName(String clusterName) {
if (clusterName == null) {
throw new MissingRequiredPropertyException("GetAddonResult", "clusterName");
}
this.clusterName = clusterName;
return this;
}
@CustomType.Setter
public Builder configurationValues(String configurationValues) {
if (configurationValues == null) {
throw new MissingRequiredPropertyException("GetAddonResult", "configurationValues");
}
this.configurationValues = configurationValues;
return this;
}
@CustomType.Setter
public Builder createdAt(String createdAt) {
if (createdAt == null) {
throw new MissingRequiredPropertyException("GetAddonResult", "createdAt");
}
this.createdAt = createdAt;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetAddonResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder modifiedAt(String modifiedAt) {
if (modifiedAt == null) {
throw new MissingRequiredPropertyException("GetAddonResult", "modifiedAt");
}
this.modifiedAt = modifiedAt;
return this;
}
@CustomType.Setter
public Builder serviceAccountRoleArn(String serviceAccountRoleArn) {
if (serviceAccountRoleArn == null) {
throw new MissingRequiredPropertyException("GetAddonResult", "serviceAccountRoleArn");
}
this.serviceAccountRoleArn = serviceAccountRoleArn;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetAddonResult", "tags");
}
this.tags = tags;
return this;
}
public GetAddonResult build() {
final var _resultValue = new GetAddonResult();
_resultValue.addonName = addonName;
_resultValue.addonVersion = addonVersion;
_resultValue.arn = arn;
_resultValue.clusterName = clusterName;
_resultValue.configurationValues = configurationValues;
_resultValue.createdAt = createdAt;
_resultValue.id = id;
_resultValue.modifiedAt = modifiedAt;
_resultValue.serviceAccountRoleArn = serviceAccountRoleArn;
_resultValue.tags = tags;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy