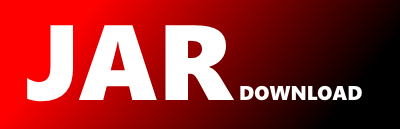
com.pulumi.aws.elasticache.inputs.GetReservedCacheNodeOfferingArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.elasticache.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
public final class GetReservedCacheNodeOfferingArgs extends com.pulumi.resources.InvokeArgs {
public static final GetReservedCacheNodeOfferingArgs Empty = new GetReservedCacheNodeOfferingArgs();
/**
* Node type for the reserved cache node.
* See AWS documentation for information on [supported node types for Redis](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/CacheNodes.SupportedTypes.html) and [guidance on selecting node types for Redis](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/nodes-select-size.html).
* See AWS documentation for information on [supported node types for Memcached](https://docs.aws.amazon.com/AmazonElastiCache/latest/mem-ug/CacheNodes.SupportedTypes.html) and [guidance on selecting node types for Memcached](https://docs.aws.amazon.com/AmazonElastiCache/latest/mem-ug/nodes-select-size.html).
*
*/
@Import(name="cacheNodeType", required=true)
private Output cacheNodeType;
/**
* @return Node type for the reserved cache node.
* See AWS documentation for information on [supported node types for Redis](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/CacheNodes.SupportedTypes.html) and [guidance on selecting node types for Redis](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/nodes-select-size.html).
* See AWS documentation for information on [supported node types for Memcached](https://docs.aws.amazon.com/AmazonElastiCache/latest/mem-ug/CacheNodes.SupportedTypes.html) and [guidance on selecting node types for Memcached](https://docs.aws.amazon.com/AmazonElastiCache/latest/mem-ug/nodes-select-size.html).
*
*/
public Output cacheNodeType() {
return this.cacheNodeType;
}
/**
* Duration of the reservation in RFC3339 duration format.
* Valid values are `P1Y` (one year) and `P3Y` (three years).
*
*/
@Import(name="duration", required=true)
private Output duration;
/**
* @return Duration of the reservation in RFC3339 duration format.
* Valid values are `P1Y` (one year) and `P3Y` (three years).
*
*/
public Output duration() {
return this.duration;
}
/**
* Offering type of this reserved cache node.
* For the latest generation of nodes (e.g. M5, R5, T4 and newer) valid values are `No Upfront`, `Partial Upfront`, and `All Upfront`.
* For other current generation nodes (i.e. T2, M3, M4, R3, or R4) the only valid value is `Heavy Utilization`.
* For previous generation modes (i.e. T1, M1, M2, or C1) valid values are `Heavy Utilization`, `Medium Utilization`, and `Light Utilization`.
*
*/
@Import(name="offeringType", required=true)
private Output offeringType;
/**
* @return Offering type of this reserved cache node.
* For the latest generation of nodes (e.g. M5, R5, T4 and newer) valid values are `No Upfront`, `Partial Upfront`, and `All Upfront`.
* For other current generation nodes (i.e. T2, M3, M4, R3, or R4) the only valid value is `Heavy Utilization`.
* For previous generation modes (i.e. T1, M1, M2, or C1) valid values are `Heavy Utilization`, `Medium Utilization`, and `Light Utilization`.
*
*/
public Output offeringType() {
return this.offeringType;
}
/**
* Engine type for the reserved cache node.
* Valid values are `redis` and `memcached`.
*
*/
@Import(name="productDescription", required=true)
private Output productDescription;
/**
* @return Engine type for the reserved cache node.
* Valid values are `redis` and `memcached`.
*
*/
public Output productDescription() {
return this.productDescription;
}
private GetReservedCacheNodeOfferingArgs() {}
private GetReservedCacheNodeOfferingArgs(GetReservedCacheNodeOfferingArgs $) {
this.cacheNodeType = $.cacheNodeType;
this.duration = $.duration;
this.offeringType = $.offeringType;
this.productDescription = $.productDescription;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetReservedCacheNodeOfferingArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetReservedCacheNodeOfferingArgs $;
public Builder() {
$ = new GetReservedCacheNodeOfferingArgs();
}
public Builder(GetReservedCacheNodeOfferingArgs defaults) {
$ = new GetReservedCacheNodeOfferingArgs(Objects.requireNonNull(defaults));
}
/**
* @param cacheNodeType Node type for the reserved cache node.
* See AWS documentation for information on [supported node types for Redis](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/CacheNodes.SupportedTypes.html) and [guidance on selecting node types for Redis](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/nodes-select-size.html).
* See AWS documentation for information on [supported node types for Memcached](https://docs.aws.amazon.com/AmazonElastiCache/latest/mem-ug/CacheNodes.SupportedTypes.html) and [guidance on selecting node types for Memcached](https://docs.aws.amazon.com/AmazonElastiCache/latest/mem-ug/nodes-select-size.html).
*
* @return builder
*
*/
public Builder cacheNodeType(Output cacheNodeType) {
$.cacheNodeType = cacheNodeType;
return this;
}
/**
* @param cacheNodeType Node type for the reserved cache node.
* See AWS documentation for information on [supported node types for Redis](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/CacheNodes.SupportedTypes.html) and [guidance on selecting node types for Redis](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/nodes-select-size.html).
* See AWS documentation for information on [supported node types for Memcached](https://docs.aws.amazon.com/AmazonElastiCache/latest/mem-ug/CacheNodes.SupportedTypes.html) and [guidance on selecting node types for Memcached](https://docs.aws.amazon.com/AmazonElastiCache/latest/mem-ug/nodes-select-size.html).
*
* @return builder
*
*/
public Builder cacheNodeType(String cacheNodeType) {
return cacheNodeType(Output.of(cacheNodeType));
}
/**
* @param duration Duration of the reservation in RFC3339 duration format.
* Valid values are `P1Y` (one year) and `P3Y` (three years).
*
* @return builder
*
*/
public Builder duration(Output duration) {
$.duration = duration;
return this;
}
/**
* @param duration Duration of the reservation in RFC3339 duration format.
* Valid values are `P1Y` (one year) and `P3Y` (three years).
*
* @return builder
*
*/
public Builder duration(String duration) {
return duration(Output.of(duration));
}
/**
* @param offeringType Offering type of this reserved cache node.
* For the latest generation of nodes (e.g. M5, R5, T4 and newer) valid values are `No Upfront`, `Partial Upfront`, and `All Upfront`.
* For other current generation nodes (i.e. T2, M3, M4, R3, or R4) the only valid value is `Heavy Utilization`.
* For previous generation modes (i.e. T1, M1, M2, or C1) valid values are `Heavy Utilization`, `Medium Utilization`, and `Light Utilization`.
*
* @return builder
*
*/
public Builder offeringType(Output offeringType) {
$.offeringType = offeringType;
return this;
}
/**
* @param offeringType Offering type of this reserved cache node.
* For the latest generation of nodes (e.g. M5, R5, T4 and newer) valid values are `No Upfront`, `Partial Upfront`, and `All Upfront`.
* For other current generation nodes (i.e. T2, M3, M4, R3, or R4) the only valid value is `Heavy Utilization`.
* For previous generation modes (i.e. T1, M1, M2, or C1) valid values are `Heavy Utilization`, `Medium Utilization`, and `Light Utilization`.
*
* @return builder
*
*/
public Builder offeringType(String offeringType) {
return offeringType(Output.of(offeringType));
}
/**
* @param productDescription Engine type for the reserved cache node.
* Valid values are `redis` and `memcached`.
*
* @return builder
*
*/
public Builder productDescription(Output productDescription) {
$.productDescription = productDescription;
return this;
}
/**
* @param productDescription Engine type for the reserved cache node.
* Valid values are `redis` and `memcached`.
*
* @return builder
*
*/
public Builder productDescription(String productDescription) {
return productDescription(Output.of(productDescription));
}
public GetReservedCacheNodeOfferingArgs build() {
if ($.cacheNodeType == null) {
throw new MissingRequiredPropertyException("GetReservedCacheNodeOfferingArgs", "cacheNodeType");
}
if ($.duration == null) {
throw new MissingRequiredPropertyException("GetReservedCacheNodeOfferingArgs", "duration");
}
if ($.offeringType == null) {
throw new MissingRequiredPropertyException("GetReservedCacheNodeOfferingArgs", "offeringType");
}
if ($.productDescription == null) {
throw new MissingRequiredPropertyException("GetReservedCacheNodeOfferingArgs", "productDescription");
}
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy