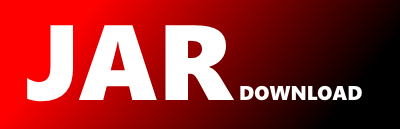
com.pulumi.aws.finspace.KxScalingGroup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.finspace;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.finspace.KxScalingGroupArgs;
import com.pulumi.aws.finspace.inputs.KxScalingGroupState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Resource for managing an AWS FinSpace Kx Scaling Group.
*
* ## Example Usage
*
* ### Basic Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.finspace.KxScalingGroup;
* import com.pulumi.aws.finspace.KxScalingGroupArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new KxScalingGroup("example", KxScalingGroupArgs.builder()
* .name("my-tf-kx-scalinggroup")
* .environmentId(exampleAwsFinspaceKxEnvironment.id())
* .availabilityZoneId("use1-az2")
* .hostType("kx.sg.4xlarge")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import an AWS FinSpace Kx Scaling Group using the `id` (environment ID and scaling group name, comma-delimited). For example:
*
* ```sh
* $ pulumi import aws:finspace/kxScalingGroup:KxScalingGroup example n3ceo7wqxoxcti5tujqwzs,my-tf-kx-scalinggroup
* ```
*
*/
@ResourceType(type="aws:finspace/kxScalingGroup:KxScalingGroup")
public class KxScalingGroup extends com.pulumi.resources.CustomResource {
/**
* Amazon Resource Name (ARN) identifier of the KX Scaling Group.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return Amazon Resource Name (ARN) identifier of the KX Scaling Group.
*
*/
public Output arn() {
return this.arn;
}
/**
* The availability zone identifiers for the requested regions.
*
*/
@Export(name="availabilityZoneId", refs={String.class}, tree="[0]")
private Output availabilityZoneId;
/**
* @return The availability zone identifiers for the requested regions.
*
*/
public Output availabilityZoneId() {
return this.availabilityZoneId;
}
/**
* The list of Managed kdb clusters that are currently active in the given scaling group.
*
*/
@Export(name="clusters", refs={List.class,String.class}, tree="[0,1]")
private Output> clusters;
/**
* @return The list of Managed kdb clusters that are currently active in the given scaling group.
*
*/
public Output> clusters() {
return this.clusters;
}
/**
* The timestamp at which the scaling group was created in FinSpace. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
@Export(name="createdTimestamp", refs={String.class}, tree="[0]")
private Output createdTimestamp;
/**
* @return The timestamp at which the scaling group was created in FinSpace. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
public Output createdTimestamp() {
return this.createdTimestamp;
}
/**
* A unique identifier for the kdb environment, where you want to create the scaling group.
*
*/
@Export(name="environmentId", refs={String.class}, tree="[0]")
private Output environmentId;
/**
* @return A unique identifier for the kdb environment, where you want to create the scaling group.
*
*/
public Output environmentId() {
return this.environmentId;
}
/**
* The memory and CPU capabilities of the scaling group host on which FinSpace Managed kdb clusters will be placed.
*
* The following arguments are optional:
*
*/
@Export(name="hostType", refs={String.class}, tree="[0]")
private Output hostType;
/**
* @return The memory and CPU capabilities of the scaling group host on which FinSpace Managed kdb clusters will be placed.
*
* The following arguments are optional:
*
*/
public Output hostType() {
return this.hostType;
}
/**
* Last timestamp at which the scaling group was updated in FinSpace. Value determined as epoch time in seconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000.
*
*/
@Export(name="lastModifiedTimestamp", refs={String.class}, tree="[0]")
private Output lastModifiedTimestamp;
/**
* @return Last timestamp at which the scaling group was updated in FinSpace. Value determined as epoch time in seconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000.
*
*/
public Output lastModifiedTimestamp() {
return this.lastModifiedTimestamp;
}
/**
* Unique name for the scaling group that you want to create.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Unique name for the scaling group that you want to create.
*
*/
public Output name() {
return this.name;
}
/**
* The status of scaling group.
* * `CREATING` – The scaling group creation is in progress.
* * `CREATE_FAILED` – The scaling group creation has failed.
* * `ACTIVE` – The scaling group is active.
* * `UPDATING` – The scaling group is in the process of being updated.
* * `UPDATE_FAILED` – The update action failed.
* * `DELETING` – The scaling group is in the process of being deleted.
* * `DELETE_FAILED` – The system failed to delete the scaling group.
* * `DELETED` – The scaling group is successfully deleted.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return The status of scaling group.
* * `CREATING` – The scaling group creation is in progress.
* * `CREATE_FAILED` – The scaling group creation has failed.
* * `ACTIVE` – The scaling group is active.
* * `UPDATING` – The scaling group is in the process of being updated.
* * `UPDATE_FAILED` – The update action failed.
* * `DELETING` – The scaling group is in the process of being deleted.
* * `DELETE_FAILED` – The system failed to delete the scaling group.
* * `DELETED` – The scaling group is successfully deleted.
*
*/
public Output status() {
return this.status;
}
/**
* The error message when a failed state occurs.
*
*/
@Export(name="statusReason", refs={String.class}, tree="[0]")
private Output statusReason;
/**
* @return The error message when a failed state occurs.
*
*/
public Output statusReason() {
return this.statusReason;
}
/**
* Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level. You can add up to 50 tags to a scaling group.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level. You can add up to 50 tags to a scaling group.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy