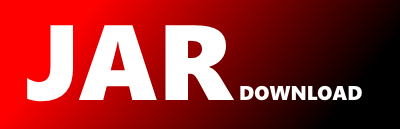
com.pulumi.aws.fsx.FileCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.fsx;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.fsx.FileCacheArgs;
import com.pulumi.aws.fsx.inputs.FileCacheState;
import com.pulumi.aws.fsx.outputs.FileCacheDataRepositoryAssociation;
import com.pulumi.aws.fsx.outputs.FileCacheLustreConfiguration;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Resource for managing an Amazon File Cache cache.
* See the [Create File Cache](https://docs.aws.amazon.com/fsx/latest/APIReference/API_CreateFileCache.html) for more information.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.fsx.FileCache;
* import com.pulumi.aws.fsx.FileCacheArgs;
* import com.pulumi.aws.fsx.inputs.FileCacheDataRepositoryAssociationArgs;
* import com.pulumi.aws.fsx.inputs.FileCacheLustreConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new FileCache("example", FileCacheArgs.builder()
* .dataRepositoryAssociations(FileCacheDataRepositoryAssociationArgs.builder()
* .dataRepositoryPath("nfs://filer.domain.com")
* .dataRepositorySubdirectories(
* "test",
* "test2")
* .fileCachePath("/ns1")
* .nfs(FileCacheDataRepositoryAssociationNfArgs.builder()
* .dnsIps(
* "192.168.0.1",
* "192.168.0.2")
* .version("NFS3")
* .build())
* .build())
* .fileCacheType("LUSTRE")
* .fileCacheTypeVersion("2.12")
* .lustreConfigurations(FileCacheLustreConfigurationArgs.builder()
* .deploymentType("CACHE_1")
* .metadataConfigurations(FileCacheLustreConfigurationMetadataConfigurationArgs.builder()
* .storageCapacity(2400)
* .build())
* .perUnitStorageThroughput(1000)
* .weeklyMaintenanceStartTime("2:05:00")
* .build())
* .subnetIds(test1.id())
* .storageCapacity(1200)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Amazon File Cache cache using the resource `id`. For example:
*
* ```sh
* $ pulumi import aws:fsx/fileCache:FileCache example fc-8012925589
* ```
*
*/
@ResourceType(type="aws:fsx/fileCache:FileCache")
public class FileCache extends com.pulumi.resources.CustomResource {
/**
* The Amazon Resource Name (ARN) for the resource.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The Amazon Resource Name (ARN) for the resource.
*
*/
public Output arn() {
return this.arn;
}
/**
* A boolean flag indicating whether tags for the cache should be copied to data repository associations. This value defaults to false.
*
*/
@Export(name="copyTagsToDataRepositoryAssociations", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> copyTagsToDataRepositoryAssociations;
/**
* @return A boolean flag indicating whether tags for the cache should be copied to data repository associations. This value defaults to false.
*
*/
public Output> copyTagsToDataRepositoryAssociations() {
return Codegen.optional(this.copyTagsToDataRepositoryAssociations);
}
/**
* A list of IDs of data repository associations that are associated with this cache.
*
*/
@Export(name="dataRepositoryAssociationIds", refs={List.class,String.class}, tree="[0,1]")
private Output> dataRepositoryAssociationIds;
/**
* @return A list of IDs of data repository associations that are associated with this cache.
*
*/
public Output> dataRepositoryAssociationIds() {
return this.dataRepositoryAssociationIds;
}
/**
* See the `data_repository_association` configuration block. Max of 8.
* A list of up to 8 configurations for data repository associations (DRAs) to be created during the cache creation. The DRAs link the cache to either an Amazon S3 data repository or a Network File System (NFS) data repository that supports the NFSv3 protocol. The DRA configurations must meet the following requirements: 1) All configurations on the list must be of the same data repository type, either all S3 or all NFS. A cache can't link to different data repository types at the same time. 2) An NFS DRA must link to an NFS file system that supports the NFSv3 protocol. DRA automatic import and automatic export is not supported.
*
*/
@Export(name="dataRepositoryAssociations", refs={List.class,FileCacheDataRepositoryAssociation.class}, tree="[0,1]")
private Output* @Nullable */ List> dataRepositoryAssociations;
/**
* @return See the `data_repository_association` configuration block. Max of 8.
* A list of up to 8 configurations for data repository associations (DRAs) to be created during the cache creation. The DRAs link the cache to either an Amazon S3 data repository or a Network File System (NFS) data repository that supports the NFSv3 protocol. The DRA configurations must meet the following requirements: 1) All configurations on the list must be of the same data repository type, either all S3 or all NFS. A cache can't link to different data repository types at the same time. 2) An NFS DRA must link to an NFS file system that supports the NFSv3 protocol. DRA automatic import and automatic export is not supported.
*
*/
public Output>> dataRepositoryAssociations() {
return Codegen.optional(this.dataRepositoryAssociations);
}
/**
* The Domain Name System (DNS) name for the cache.
*
*/
@Export(name="dnsName", refs={String.class}, tree="[0]")
private Output dnsName;
/**
* @return The Domain Name System (DNS) name for the cache.
*
*/
public Output dnsName() {
return this.dnsName;
}
/**
* The system-generated, unique ID of the cache.
*
*/
@Export(name="fileCacheId", refs={String.class}, tree="[0]")
private Output fileCacheId;
/**
* @return The system-generated, unique ID of the cache.
*
*/
public Output fileCacheId() {
return this.fileCacheId;
}
/**
* The type of cache that you're creating. The only supported value is `LUSTRE`.
*
*/
@Export(name="fileCacheType", refs={String.class}, tree="[0]")
private Output fileCacheType;
/**
* @return The type of cache that you're creating. The only supported value is `LUSTRE`.
*
*/
public Output fileCacheType() {
return this.fileCacheType;
}
/**
* The version for the type of cache that you're creating. The only supported value is `2.12`.
*
*/
@Export(name="fileCacheTypeVersion", refs={String.class}, tree="[0]")
private Output fileCacheTypeVersion;
/**
* @return The version for the type of cache that you're creating. The only supported value is `2.12`.
*
*/
public Output fileCacheTypeVersion() {
return this.fileCacheTypeVersion;
}
/**
* Specifies the ID of the AWS Key Management Service (AWS KMS) key to use for encrypting data on an Amazon File Cache. If a KmsKeyId isn't specified, the Amazon FSx-managed AWS KMS key for your account is used.
*
*/
@Export(name="kmsKeyId", refs={String.class}, tree="[0]")
private Output kmsKeyId;
/**
* @return Specifies the ID of the AWS Key Management Service (AWS KMS) key to use for encrypting data on an Amazon File Cache. If a KmsKeyId isn't specified, the Amazon FSx-managed AWS KMS key for your account is used.
*
*/
public Output kmsKeyId() {
return this.kmsKeyId;
}
/**
* See the `lustre_configuration` block. Required when `file_cache_type` is `LUSTRE`.
*
*/
@Export(name="lustreConfigurations", refs={List.class,FileCacheLustreConfiguration.class}, tree="[0,1]")
private Output* @Nullable */ List> lustreConfigurations;
/**
* @return See the `lustre_configuration` block. Required when `file_cache_type` is `LUSTRE`.
*
*/
public Output>> lustreConfigurations() {
return Codegen.optional(this.lustreConfigurations);
}
/**
* A list of network interface IDs.
*
*/
@Export(name="networkInterfaceIds", refs={List.class,String.class}, tree="[0,1]")
private Output> networkInterfaceIds;
/**
* @return A list of network interface IDs.
*
*/
public Output> networkInterfaceIds() {
return this.networkInterfaceIds;
}
@Export(name="ownerId", refs={String.class}, tree="[0]")
private Output ownerId;
public Output ownerId() {
return this.ownerId;
}
/**
* A list of IDs specifying the security groups to apply to all network interfaces created for Amazon File Cache access.
*
*/
@Export(name="securityGroupIds", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> securityGroupIds;
/**
* @return A list of IDs specifying the security groups to apply to all network interfaces created for Amazon File Cache access.
*
*/
public Output>> securityGroupIds() {
return Codegen.optional(this.securityGroupIds);
}
/**
* The storage capacity of the cache in gibibytes (GiB). Valid values are `1200` GiB, `2400` GiB, and increments of `2400` GiB.
*
*/
@Export(name="storageCapacity", refs={Integer.class}, tree="[0]")
private Output storageCapacity;
/**
* @return The storage capacity of the cache in gibibytes (GiB). Valid values are `1200` GiB, `2400` GiB, and increments of `2400` GiB.
*
*/
public Output storageCapacity() {
return this.storageCapacity;
}
/**
* A list of subnet IDs that the cache will be accessible from. You can specify only one subnet ID.
*
* The following arguments are optional:
*
*/
@Export(name="subnetIds", refs={List.class,String.class}, tree="[0,1]")
private Output> subnetIds;
/**
* @return A list of subnet IDs that the cache will be accessible from. You can specify only one subnet ID.
*
* The following arguments are optional:
*
*/
public Output> subnetIds() {
return this.subnetIds;
}
/**
* A map of tags to assign to the file cache. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the file cache. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy