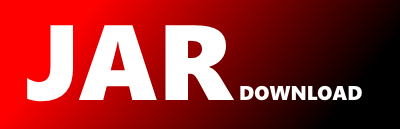
com.pulumi.aws.glue.WorkflowArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.glue;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class WorkflowArgs extends com.pulumi.resources.ResourceArgs {
public static final WorkflowArgs Empty = new WorkflowArgs();
/**
* A map of default run properties for this workflow. These properties are passed to all jobs associated to the workflow.
*
*/
@Import(name="defaultRunProperties")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy