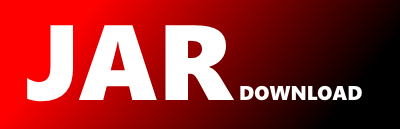
com.pulumi.aws.iot.inputs.TopicRuleErrorActionDynamodbArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.iot.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TopicRuleErrorActionDynamodbArgs extends com.pulumi.resources.ResourceArgs {
public static final TopicRuleErrorActionDynamodbArgs Empty = new TopicRuleErrorActionDynamodbArgs();
/**
* The hash key name.
*
*/
@Import(name="hashKeyField", required=true)
private Output hashKeyField;
/**
* @return The hash key name.
*
*/
public Output hashKeyField() {
return this.hashKeyField;
}
/**
* The hash key type. Valid values are "STRING" or "NUMBER".
*
*/
@Import(name="hashKeyType")
private @Nullable Output hashKeyType;
/**
* @return The hash key type. Valid values are "STRING" or "NUMBER".
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy