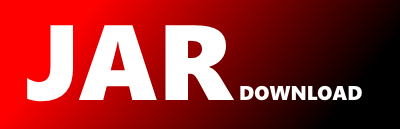
com.pulumi.aws.iot.outputs.TopicRuleErrorActionDynamodb Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.iot.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class TopicRuleErrorActionDynamodb {
/**
* @return The hash key name.
*
*/
private String hashKeyField;
/**
* @return The hash key type. Valid values are "STRING" or "NUMBER".
*
*/
private @Nullable String hashKeyType;
/**
* @return The hash key value.
*
*/
private String hashKeyValue;
/**
* @return The operation. Valid values are "INSERT", "UPDATE", or "DELETE".
*
*/
private @Nullable String operation;
/**
* @return The action payload.
*
*/
private @Nullable String payloadField;
/**
* @return The range key name.
*
*/
private @Nullable String rangeKeyField;
/**
* @return The range key type. Valid values are "STRING" or "NUMBER".
*
*/
private @Nullable String rangeKeyType;
/**
* @return The range key value.
*
*/
private @Nullable String rangeKeyValue;
/**
* @return The ARN of the IAM role that grants access to the DynamoDB table.
*
*/
private String roleArn;
/**
* @return The name of the DynamoDB table.
*
*/
private String tableName;
private TopicRuleErrorActionDynamodb() {}
/**
* @return The hash key name.
*
*/
public String hashKeyField() {
return this.hashKeyField;
}
/**
* @return The hash key type. Valid values are "STRING" or "NUMBER".
*
*/
public Optional hashKeyType() {
return Optional.ofNullable(this.hashKeyType);
}
/**
* @return The hash key value.
*
*/
public String hashKeyValue() {
return this.hashKeyValue;
}
/**
* @return The operation. Valid values are "INSERT", "UPDATE", or "DELETE".
*
*/
public Optional operation() {
return Optional.ofNullable(this.operation);
}
/**
* @return The action payload.
*
*/
public Optional payloadField() {
return Optional.ofNullable(this.payloadField);
}
/**
* @return The range key name.
*
*/
public Optional rangeKeyField() {
return Optional.ofNullable(this.rangeKeyField);
}
/**
* @return The range key type. Valid values are "STRING" or "NUMBER".
*
*/
public Optional rangeKeyType() {
return Optional.ofNullable(this.rangeKeyType);
}
/**
* @return The range key value.
*
*/
public Optional rangeKeyValue() {
return Optional.ofNullable(this.rangeKeyValue);
}
/**
* @return The ARN of the IAM role that grants access to the DynamoDB table.
*
*/
public String roleArn() {
return this.roleArn;
}
/**
* @return The name of the DynamoDB table.
*
*/
public String tableName() {
return this.tableName;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(TopicRuleErrorActionDynamodb defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String hashKeyField;
private @Nullable String hashKeyType;
private String hashKeyValue;
private @Nullable String operation;
private @Nullable String payloadField;
private @Nullable String rangeKeyField;
private @Nullable String rangeKeyType;
private @Nullable String rangeKeyValue;
private String roleArn;
private String tableName;
public Builder() {}
public Builder(TopicRuleErrorActionDynamodb defaults) {
Objects.requireNonNull(defaults);
this.hashKeyField = defaults.hashKeyField;
this.hashKeyType = defaults.hashKeyType;
this.hashKeyValue = defaults.hashKeyValue;
this.operation = defaults.operation;
this.payloadField = defaults.payloadField;
this.rangeKeyField = defaults.rangeKeyField;
this.rangeKeyType = defaults.rangeKeyType;
this.rangeKeyValue = defaults.rangeKeyValue;
this.roleArn = defaults.roleArn;
this.tableName = defaults.tableName;
}
@CustomType.Setter
public Builder hashKeyField(String hashKeyField) {
if (hashKeyField == null) {
throw new MissingRequiredPropertyException("TopicRuleErrorActionDynamodb", "hashKeyField");
}
this.hashKeyField = hashKeyField;
return this;
}
@CustomType.Setter
public Builder hashKeyType(@Nullable String hashKeyType) {
this.hashKeyType = hashKeyType;
return this;
}
@CustomType.Setter
public Builder hashKeyValue(String hashKeyValue) {
if (hashKeyValue == null) {
throw new MissingRequiredPropertyException("TopicRuleErrorActionDynamodb", "hashKeyValue");
}
this.hashKeyValue = hashKeyValue;
return this;
}
@CustomType.Setter
public Builder operation(@Nullable String operation) {
this.operation = operation;
return this;
}
@CustomType.Setter
public Builder payloadField(@Nullable String payloadField) {
this.payloadField = payloadField;
return this;
}
@CustomType.Setter
public Builder rangeKeyField(@Nullable String rangeKeyField) {
this.rangeKeyField = rangeKeyField;
return this;
}
@CustomType.Setter
public Builder rangeKeyType(@Nullable String rangeKeyType) {
this.rangeKeyType = rangeKeyType;
return this;
}
@CustomType.Setter
public Builder rangeKeyValue(@Nullable String rangeKeyValue) {
this.rangeKeyValue = rangeKeyValue;
return this;
}
@CustomType.Setter
public Builder roleArn(String roleArn) {
if (roleArn == null) {
throw new MissingRequiredPropertyException("TopicRuleErrorActionDynamodb", "roleArn");
}
this.roleArn = roleArn;
return this;
}
@CustomType.Setter
public Builder tableName(String tableName) {
if (tableName == null) {
throw new MissingRequiredPropertyException("TopicRuleErrorActionDynamodb", "tableName");
}
this.tableName = tableName;
return this;
}
public TopicRuleErrorActionDynamodb build() {
final var _resultValue = new TopicRuleErrorActionDynamodb();
_resultValue.hashKeyField = hashKeyField;
_resultValue.hashKeyType = hashKeyType;
_resultValue.hashKeyValue = hashKeyValue;
_resultValue.operation = operation;
_resultValue.payloadField = payloadField;
_resultValue.rangeKeyField = rangeKeyField;
_resultValue.rangeKeyType = rangeKeyType;
_resultValue.rangeKeyValue = rangeKeyValue;
_resultValue.roleArn = roleArn;
_resultValue.tableName = tableName;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy