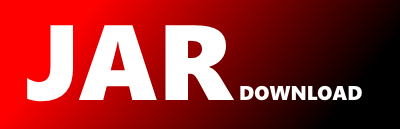
com.pulumi.aws.lex.inputs.V2modelsIntentConfirmationSettingDeclinationResponseMessageGroupMessageImageResponseCardArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lex.inputs;
import com.pulumi.aws.lex.inputs.V2modelsIntentConfirmationSettingDeclinationResponseMessageGroupMessageImageResponseCardButtonArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class V2modelsIntentConfirmationSettingDeclinationResponseMessageGroupMessageImageResponseCardArgs extends com.pulumi.resources.ResourceArgs {
public static final V2modelsIntentConfirmationSettingDeclinationResponseMessageGroupMessageImageResponseCardArgs Empty = new V2modelsIntentConfirmationSettingDeclinationResponseMessageGroupMessageImageResponseCardArgs();
/**
* Configuration blocks for buttons that should be displayed on the response card. The arrangement of the buttons is determined by the platform that displays the button. See `button`.
*
*/
@Import(name="buttons")
private @Nullable Output> buttons;
/**
* @return Configuration blocks for buttons that should be displayed on the response card. The arrangement of the buttons is determined by the platform that displays the button. See `button`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy