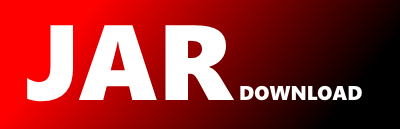
com.pulumi.aws.lex.outputs.GetBotResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lex.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetBotResult {
/**
* @return ARN of the bot.
*
*/
private String arn;
/**
* @return Checksum of the bot used to identify a specific revision of the bot's `$LATEST` version.
*
*/
private String checksum;
/**
* @return If this Amazon Lex Bot is related to a website, program, or other application that is directed or targeted, in whole or in part, to children under age 13 and subject to COPPA.
*
*/
private Boolean childDirected;
/**
* @return Date that the bot was created.
*
*/
private String createdDate;
/**
* @return Description of the bot.
*
*/
private String description;
/**
* @return When set to true user utterances are sent to Amazon Comprehend for sentiment analysis.
*
*/
private Boolean detectSentiment;
/**
* @return Set to true if natural language understanding improvements are enabled.
*
*/
private Boolean enableModelImprovements;
/**
* @return If the `status` is `FAILED`, the reason why the bot failed to build.
*
*/
private String failureReason;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
*/
private Integer idleSessionTtlInSeconds;
/**
* @return Date that the bot was updated.
*
*/
private String lastUpdatedDate;
/**
* @return Target locale for the bot. Any intent used in the bot must be compatible with the locale of the bot.
*
*/
private String locale;
/**
* @return Name of the bot, case sensitive.
*
*/
private String name;
/**
* @return The threshold where Amazon Lex will insert the AMAZON.FallbackIntent, AMAZON.KendraSearchIntent, or both when returning alternative intents in a PostContent or PostText response. AMAZON.FallbackIntent and AMAZON.KendraSearchIntent are only inserted if they are configured for the bot.
*
*/
private Double nluIntentConfidenceThreshold;
/**
* @return Status of the bot.
*
*/
private String status;
/**
* @return Version of the bot. For a new bot, the version is always `$LATEST`.
*
*/
private @Nullable String version;
/**
* @return Amazon Polly voice ID that the Amazon Lex Bot uses for voice interactions with the user.
*
*/
private String voiceId;
private GetBotResult() {}
/**
* @return ARN of the bot.
*
*/
public String arn() {
return this.arn;
}
/**
* @return Checksum of the bot used to identify a specific revision of the bot's `$LATEST` version.
*
*/
public String checksum() {
return this.checksum;
}
/**
* @return If this Amazon Lex Bot is related to a website, program, or other application that is directed or targeted, in whole or in part, to children under age 13 and subject to COPPA.
*
*/
public Boolean childDirected() {
return this.childDirected;
}
/**
* @return Date that the bot was created.
*
*/
public String createdDate() {
return this.createdDate;
}
/**
* @return Description of the bot.
*
*/
public String description() {
return this.description;
}
/**
* @return When set to true user utterances are sent to Amazon Comprehend for sentiment analysis.
*
*/
public Boolean detectSentiment() {
return this.detectSentiment;
}
/**
* @return Set to true if natural language understanding improvements are enabled.
*
*/
public Boolean enableModelImprovements() {
return this.enableModelImprovements;
}
/**
* @return If the `status` is `FAILED`, the reason why the bot failed to build.
*
*/
public String failureReason() {
return this.failureReason;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
*/
public Integer idleSessionTtlInSeconds() {
return this.idleSessionTtlInSeconds;
}
/**
* @return Date that the bot was updated.
*
*/
public String lastUpdatedDate() {
return this.lastUpdatedDate;
}
/**
* @return Target locale for the bot. Any intent used in the bot must be compatible with the locale of the bot.
*
*/
public String locale() {
return this.locale;
}
/**
* @return Name of the bot, case sensitive.
*
*/
public String name() {
return this.name;
}
/**
* @return The threshold where Amazon Lex will insert the AMAZON.FallbackIntent, AMAZON.KendraSearchIntent, or both when returning alternative intents in a PostContent or PostText response. AMAZON.FallbackIntent and AMAZON.KendraSearchIntent are only inserted if they are configured for the bot.
*
*/
public Double nluIntentConfidenceThreshold() {
return this.nluIntentConfidenceThreshold;
}
/**
* @return Status of the bot.
*
*/
public String status() {
return this.status;
}
/**
* @return Version of the bot. For a new bot, the version is always `$LATEST`.
*
*/
public Optional version() {
return Optional.ofNullable(this.version);
}
/**
* @return Amazon Polly voice ID that the Amazon Lex Bot uses for voice interactions with the user.
*
*/
public String voiceId() {
return this.voiceId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBotResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private String checksum;
private Boolean childDirected;
private String createdDate;
private String description;
private Boolean detectSentiment;
private Boolean enableModelImprovements;
private String failureReason;
private String id;
private Integer idleSessionTtlInSeconds;
private String lastUpdatedDate;
private String locale;
private String name;
private Double nluIntentConfidenceThreshold;
private String status;
private @Nullable String version;
private String voiceId;
public Builder() {}
public Builder(GetBotResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.checksum = defaults.checksum;
this.childDirected = defaults.childDirected;
this.createdDate = defaults.createdDate;
this.description = defaults.description;
this.detectSentiment = defaults.detectSentiment;
this.enableModelImprovements = defaults.enableModelImprovements;
this.failureReason = defaults.failureReason;
this.id = defaults.id;
this.idleSessionTtlInSeconds = defaults.idleSessionTtlInSeconds;
this.lastUpdatedDate = defaults.lastUpdatedDate;
this.locale = defaults.locale;
this.name = defaults.name;
this.nluIntentConfidenceThreshold = defaults.nluIntentConfidenceThreshold;
this.status = defaults.status;
this.version = defaults.version;
this.voiceId = defaults.voiceId;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetBotResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder checksum(String checksum) {
if (checksum == null) {
throw new MissingRequiredPropertyException("GetBotResult", "checksum");
}
this.checksum = checksum;
return this;
}
@CustomType.Setter
public Builder childDirected(Boolean childDirected) {
if (childDirected == null) {
throw new MissingRequiredPropertyException("GetBotResult", "childDirected");
}
this.childDirected = childDirected;
return this;
}
@CustomType.Setter
public Builder createdDate(String createdDate) {
if (createdDate == null) {
throw new MissingRequiredPropertyException("GetBotResult", "createdDate");
}
this.createdDate = createdDate;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetBotResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder detectSentiment(Boolean detectSentiment) {
if (detectSentiment == null) {
throw new MissingRequiredPropertyException("GetBotResult", "detectSentiment");
}
this.detectSentiment = detectSentiment;
return this;
}
@CustomType.Setter
public Builder enableModelImprovements(Boolean enableModelImprovements) {
if (enableModelImprovements == null) {
throw new MissingRequiredPropertyException("GetBotResult", "enableModelImprovements");
}
this.enableModelImprovements = enableModelImprovements;
return this;
}
@CustomType.Setter
public Builder failureReason(String failureReason) {
if (failureReason == null) {
throw new MissingRequiredPropertyException("GetBotResult", "failureReason");
}
this.failureReason = failureReason;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetBotResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder idleSessionTtlInSeconds(Integer idleSessionTtlInSeconds) {
if (idleSessionTtlInSeconds == null) {
throw new MissingRequiredPropertyException("GetBotResult", "idleSessionTtlInSeconds");
}
this.idleSessionTtlInSeconds = idleSessionTtlInSeconds;
return this;
}
@CustomType.Setter
public Builder lastUpdatedDate(String lastUpdatedDate) {
if (lastUpdatedDate == null) {
throw new MissingRequiredPropertyException("GetBotResult", "lastUpdatedDate");
}
this.lastUpdatedDate = lastUpdatedDate;
return this;
}
@CustomType.Setter
public Builder locale(String locale) {
if (locale == null) {
throw new MissingRequiredPropertyException("GetBotResult", "locale");
}
this.locale = locale;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetBotResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder nluIntentConfidenceThreshold(Double nluIntentConfidenceThreshold) {
if (nluIntentConfidenceThreshold == null) {
throw new MissingRequiredPropertyException("GetBotResult", "nluIntentConfidenceThreshold");
}
this.nluIntentConfidenceThreshold = nluIntentConfidenceThreshold;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetBotResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder version(@Nullable String version) {
this.version = version;
return this;
}
@CustomType.Setter
public Builder voiceId(String voiceId) {
if (voiceId == null) {
throw new MissingRequiredPropertyException("GetBotResult", "voiceId");
}
this.voiceId = voiceId;
return this;
}
public GetBotResult build() {
final var _resultValue = new GetBotResult();
_resultValue.arn = arn;
_resultValue.checksum = checksum;
_resultValue.childDirected = childDirected;
_resultValue.createdDate = createdDate;
_resultValue.description = description;
_resultValue.detectSentiment = detectSentiment;
_resultValue.enableModelImprovements = enableModelImprovements;
_resultValue.failureReason = failureReason;
_resultValue.id = id;
_resultValue.idleSessionTtlInSeconds = idleSessionTtlInSeconds;
_resultValue.lastUpdatedDate = lastUpdatedDate;
_resultValue.locale = locale;
_resultValue.name = name;
_resultValue.nluIntentConfidenceThreshold = nluIntentConfidenceThreshold;
_resultValue.status = status;
_resultValue.version = version;
_resultValue.voiceId = voiceId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy