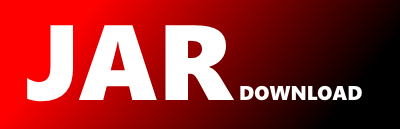
com.pulumi.aws.lex.outputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecification Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lex.outputs;
import com.pulumi.aws.lex.outputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureConditional;
import com.pulumi.aws.lex.outputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureNextStep;
import com.pulumi.aws.lex.outputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureResponse;
import com.pulumi.aws.lex.outputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessConditional;
import com.pulumi.aws.lex.outputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessNextStep;
import com.pulumi.aws.lex.outputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessResponse;
import com.pulumi.aws.lex.outputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutConditional;
import com.pulumi.aws.lex.outputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutNextStep;
import com.pulumi.aws.lex.outputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutResponse;
import com.pulumi.core.annotations.CustomType;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecification {
/**
* @return Configuration block for conditional branches to evaluate after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed. See `failure_conditional`.
*
*/
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureConditional failureConditional;
/**
* @return Configuration block for the next step the bot runs after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed. See `failure_next_step`.
*
*/
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureNextStep failureNextStep;
/**
* @return Configuration block for message groups that Amazon Lex uses to respond the user input. See `failure_response`.
*
*/
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureResponse failureResponse;
/**
* @return Configuration block for conditional branches to evaluate after the dialog code hook finishes successfully. See `success_conditional`.
*
*/
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessConditional successConditional;
/**
* @return Configuration block for the next step the bot runs after the dialog code hook finishes successfully. See `success_next_step`.
*
*/
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessNextStep successNextStep;
/**
* @return Configuration block for message groups that Amazon Lex uses to respond the user input. See `success_response`.
*
*/
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessResponse successResponse;
/**
* @return Configuration block for conditional branches to evaluate if the code hook times out. See `timeout_conditional`.
*
*/
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutConditional timeoutConditional;
/**
* @return Configuration block for the next step that the bot runs when the code hook times out. See `timeout_next_step`.
*
*/
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutNextStep timeoutNextStep;
/**
* @return Configuration block for a list of message groups that Amazon Lex uses to respond the user input. See `timeout_response`.
*
*/
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutResponse timeoutResponse;
private V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecification() {}
/**
* @return Configuration block for conditional branches to evaluate after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed. See `failure_conditional`.
*
*/
public Optional failureConditional() {
return Optional.ofNullable(this.failureConditional);
}
/**
* @return Configuration block for the next step the bot runs after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed. See `failure_next_step`.
*
*/
public Optional failureNextStep() {
return Optional.ofNullable(this.failureNextStep);
}
/**
* @return Configuration block for message groups that Amazon Lex uses to respond the user input. See `failure_response`.
*
*/
public Optional failureResponse() {
return Optional.ofNullable(this.failureResponse);
}
/**
* @return Configuration block for conditional branches to evaluate after the dialog code hook finishes successfully. See `success_conditional`.
*
*/
public Optional successConditional() {
return Optional.ofNullable(this.successConditional);
}
/**
* @return Configuration block for the next step the bot runs after the dialog code hook finishes successfully. See `success_next_step`.
*
*/
public Optional successNextStep() {
return Optional.ofNullable(this.successNextStep);
}
/**
* @return Configuration block for message groups that Amazon Lex uses to respond the user input. See `success_response`.
*
*/
public Optional successResponse() {
return Optional.ofNullable(this.successResponse);
}
/**
* @return Configuration block for conditional branches to evaluate if the code hook times out. See `timeout_conditional`.
*
*/
public Optional timeoutConditional() {
return Optional.ofNullable(this.timeoutConditional);
}
/**
* @return Configuration block for the next step that the bot runs when the code hook times out. See `timeout_next_step`.
*
*/
public Optional timeoutNextStep() {
return Optional.ofNullable(this.timeoutNextStep);
}
/**
* @return Configuration block for a list of message groups that Amazon Lex uses to respond the user input. See `timeout_response`.
*
*/
public Optional timeoutResponse() {
return Optional.ofNullable(this.timeoutResponse);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecification defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureConditional failureConditional;
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureNextStep failureNextStep;
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureResponse failureResponse;
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessConditional successConditional;
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessNextStep successNextStep;
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessResponse successResponse;
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutConditional timeoutConditional;
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutNextStep timeoutNextStep;
private @Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutResponse timeoutResponse;
public Builder() {}
public Builder(V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecification defaults) {
Objects.requireNonNull(defaults);
this.failureConditional = defaults.failureConditional;
this.failureNextStep = defaults.failureNextStep;
this.failureResponse = defaults.failureResponse;
this.successConditional = defaults.successConditional;
this.successNextStep = defaults.successNextStep;
this.successResponse = defaults.successResponse;
this.timeoutConditional = defaults.timeoutConditional;
this.timeoutNextStep = defaults.timeoutNextStep;
this.timeoutResponse = defaults.timeoutResponse;
}
@CustomType.Setter
public Builder failureConditional(@Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureConditional failureConditional) {
this.failureConditional = failureConditional;
return this;
}
@CustomType.Setter
public Builder failureNextStep(@Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureNextStep failureNextStep) {
this.failureNextStep = failureNextStep;
return this;
}
@CustomType.Setter
public Builder failureResponse(@Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureResponse failureResponse) {
this.failureResponse = failureResponse;
return this;
}
@CustomType.Setter
public Builder successConditional(@Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessConditional successConditional) {
this.successConditional = successConditional;
return this;
}
@CustomType.Setter
public Builder successNextStep(@Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessNextStep successNextStep) {
this.successNextStep = successNextStep;
return this;
}
@CustomType.Setter
public Builder successResponse(@Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessResponse successResponse) {
this.successResponse = successResponse;
return this;
}
@CustomType.Setter
public Builder timeoutConditional(@Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutConditional timeoutConditional) {
this.timeoutConditional = timeoutConditional;
return this;
}
@CustomType.Setter
public Builder timeoutNextStep(@Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutNextStep timeoutNextStep) {
this.timeoutNextStep = timeoutNextStep;
return this;
}
@CustomType.Setter
public Builder timeoutResponse(@Nullable V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutResponse timeoutResponse) {
this.timeoutResponse = timeoutResponse;
return this;
}
public V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecification build() {
final var _resultValue = new V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecification();
_resultValue.failureConditional = failureConditional;
_resultValue.failureNextStep = failureNextStep;
_resultValue.failureResponse = failureResponse;
_resultValue.successConditional = successConditional;
_resultValue.successNextStep = successNextStep;
_resultValue.successResponse = successResponse;
_resultValue.timeoutConditional = timeoutConditional;
_resultValue.timeoutNextStep = timeoutNextStep;
_resultValue.timeoutResponse = timeoutResponse;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy