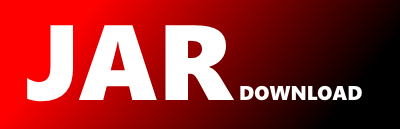
com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsAudioDescriptionCodecSettingsEac3AtmosSettingsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.medialive.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ChannelEncoderSettingsAudioDescriptionCodecSettingsEac3AtmosSettingsArgs extends com.pulumi.resources.ResourceArgs {
public static final ChannelEncoderSettingsAudioDescriptionCodecSettingsEac3AtmosSettingsArgs Empty = new ChannelEncoderSettingsAudioDescriptionCodecSettingsEac3AtmosSettingsArgs();
/**
* Average bitrate in bits/second.
*
*/
@Import(name="bitrate")
private @Nullable Output bitrate;
/**
* @return Average bitrate in bits/second.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy