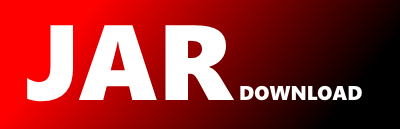
com.pulumi.aws.medialive.outputs.ChannelEncoderSettingsOutputGroupOutputOutputSettingsHlsOutputSettingsHlsSettingsStandardHlsSettingsM3u8Settings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.medialive.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ChannelEncoderSettingsOutputGroupOutputOutputSettingsHlsOutputSettingsHlsSettingsStandardHlsSettingsM3u8Settings {
private @Nullable Integer audioFramesPerPes;
private @Nullable String audioPids;
private @Nullable String ecmPid;
private @Nullable String nielsenId3Behavior;
private @Nullable Integer patInterval;
private @Nullable String pcrControl;
private @Nullable Integer pcrPeriod;
private @Nullable String pcrPid;
private @Nullable Integer pmtInterval;
private @Nullable String pmtPid;
private @Nullable Integer programNum;
private @Nullable String scte35Behavior;
/**
* @return PID from which to read SCTE-35 messages.
*
*/
private @Nullable String scte35Pid;
private @Nullable String timedMetadataBehavior;
private @Nullable String timedMetadataPid;
private @Nullable Integer transportStreamId;
private @Nullable String videoPid;
private ChannelEncoderSettingsOutputGroupOutputOutputSettingsHlsOutputSettingsHlsSettingsStandardHlsSettingsM3u8Settings() {}
public Optional audioFramesPerPes() {
return Optional.ofNullable(this.audioFramesPerPes);
}
public Optional audioPids() {
return Optional.ofNullable(this.audioPids);
}
public Optional ecmPid() {
return Optional.ofNullable(this.ecmPid);
}
public Optional nielsenId3Behavior() {
return Optional.ofNullable(this.nielsenId3Behavior);
}
public Optional patInterval() {
return Optional.ofNullable(this.patInterval);
}
public Optional pcrControl() {
return Optional.ofNullable(this.pcrControl);
}
public Optional pcrPeriod() {
return Optional.ofNullable(this.pcrPeriod);
}
public Optional pcrPid() {
return Optional.ofNullable(this.pcrPid);
}
public Optional pmtInterval() {
return Optional.ofNullable(this.pmtInterval);
}
public Optional pmtPid() {
return Optional.ofNullable(this.pmtPid);
}
public Optional programNum() {
return Optional.ofNullable(this.programNum);
}
public Optional scte35Behavior() {
return Optional.ofNullable(this.scte35Behavior);
}
/**
* @return PID from which to read SCTE-35 messages.
*
*/
public Optional scte35Pid() {
return Optional.ofNullable(this.scte35Pid);
}
public Optional timedMetadataBehavior() {
return Optional.ofNullable(this.timedMetadataBehavior);
}
public Optional timedMetadataPid() {
return Optional.ofNullable(this.timedMetadataPid);
}
public Optional transportStreamId() {
return Optional.ofNullable(this.transportStreamId);
}
public Optional videoPid() {
return Optional.ofNullable(this.videoPid);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ChannelEncoderSettingsOutputGroupOutputOutputSettingsHlsOutputSettingsHlsSettingsStandardHlsSettingsM3u8Settings defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer audioFramesPerPes;
private @Nullable String audioPids;
private @Nullable String ecmPid;
private @Nullable String nielsenId3Behavior;
private @Nullable Integer patInterval;
private @Nullable String pcrControl;
private @Nullable Integer pcrPeriod;
private @Nullable String pcrPid;
private @Nullable Integer pmtInterval;
private @Nullable String pmtPid;
private @Nullable Integer programNum;
private @Nullable String scte35Behavior;
private @Nullable String scte35Pid;
private @Nullable String timedMetadataBehavior;
private @Nullable String timedMetadataPid;
private @Nullable Integer transportStreamId;
private @Nullable String videoPid;
public Builder() {}
public Builder(ChannelEncoderSettingsOutputGroupOutputOutputSettingsHlsOutputSettingsHlsSettingsStandardHlsSettingsM3u8Settings defaults) {
Objects.requireNonNull(defaults);
this.audioFramesPerPes = defaults.audioFramesPerPes;
this.audioPids = defaults.audioPids;
this.ecmPid = defaults.ecmPid;
this.nielsenId3Behavior = defaults.nielsenId3Behavior;
this.patInterval = defaults.patInterval;
this.pcrControl = defaults.pcrControl;
this.pcrPeriod = defaults.pcrPeriod;
this.pcrPid = defaults.pcrPid;
this.pmtInterval = defaults.pmtInterval;
this.pmtPid = defaults.pmtPid;
this.programNum = defaults.programNum;
this.scte35Behavior = defaults.scte35Behavior;
this.scte35Pid = defaults.scte35Pid;
this.timedMetadataBehavior = defaults.timedMetadataBehavior;
this.timedMetadataPid = defaults.timedMetadataPid;
this.transportStreamId = defaults.transportStreamId;
this.videoPid = defaults.videoPid;
}
@CustomType.Setter
public Builder audioFramesPerPes(@Nullable Integer audioFramesPerPes) {
this.audioFramesPerPes = audioFramesPerPes;
return this;
}
@CustomType.Setter
public Builder audioPids(@Nullable String audioPids) {
this.audioPids = audioPids;
return this;
}
@CustomType.Setter
public Builder ecmPid(@Nullable String ecmPid) {
this.ecmPid = ecmPid;
return this;
}
@CustomType.Setter
public Builder nielsenId3Behavior(@Nullable String nielsenId3Behavior) {
this.nielsenId3Behavior = nielsenId3Behavior;
return this;
}
@CustomType.Setter
public Builder patInterval(@Nullable Integer patInterval) {
this.patInterval = patInterval;
return this;
}
@CustomType.Setter
public Builder pcrControl(@Nullable String pcrControl) {
this.pcrControl = pcrControl;
return this;
}
@CustomType.Setter
public Builder pcrPeriod(@Nullable Integer pcrPeriod) {
this.pcrPeriod = pcrPeriod;
return this;
}
@CustomType.Setter
public Builder pcrPid(@Nullable String pcrPid) {
this.pcrPid = pcrPid;
return this;
}
@CustomType.Setter
public Builder pmtInterval(@Nullable Integer pmtInterval) {
this.pmtInterval = pmtInterval;
return this;
}
@CustomType.Setter
public Builder pmtPid(@Nullable String pmtPid) {
this.pmtPid = pmtPid;
return this;
}
@CustomType.Setter
public Builder programNum(@Nullable Integer programNum) {
this.programNum = programNum;
return this;
}
@CustomType.Setter
public Builder scte35Behavior(@Nullable String scte35Behavior) {
this.scte35Behavior = scte35Behavior;
return this;
}
@CustomType.Setter
public Builder scte35Pid(@Nullable String scte35Pid) {
this.scte35Pid = scte35Pid;
return this;
}
@CustomType.Setter
public Builder timedMetadataBehavior(@Nullable String timedMetadataBehavior) {
this.timedMetadataBehavior = timedMetadataBehavior;
return this;
}
@CustomType.Setter
public Builder timedMetadataPid(@Nullable String timedMetadataPid) {
this.timedMetadataPid = timedMetadataPid;
return this;
}
@CustomType.Setter
public Builder transportStreamId(@Nullable Integer transportStreamId) {
this.transportStreamId = transportStreamId;
return this;
}
@CustomType.Setter
public Builder videoPid(@Nullable String videoPid) {
this.videoPid = videoPid;
return this;
}
public ChannelEncoderSettingsOutputGroupOutputOutputSettingsHlsOutputSettingsHlsSettingsStandardHlsSettingsM3u8Settings build() {
final var _resultValue = new ChannelEncoderSettingsOutputGroupOutputOutputSettingsHlsOutputSettingsHlsSettingsStandardHlsSettingsM3u8Settings();
_resultValue.audioFramesPerPes = audioFramesPerPes;
_resultValue.audioPids = audioPids;
_resultValue.ecmPid = ecmPid;
_resultValue.nielsenId3Behavior = nielsenId3Behavior;
_resultValue.patInterval = patInterval;
_resultValue.pcrControl = pcrControl;
_resultValue.pcrPeriod = pcrPeriod;
_resultValue.pcrPid = pcrPid;
_resultValue.pmtInterval = pmtInterval;
_resultValue.pmtPid = pmtPid;
_resultValue.programNum = programNum;
_resultValue.scte35Behavior = scte35Behavior;
_resultValue.scte35Pid = scte35Pid;
_resultValue.timedMetadataBehavior = timedMetadataBehavior;
_resultValue.timedMetadataPid = timedMetadataPid;
_resultValue.transportStreamId = transportStreamId;
_resultValue.videoPid = videoPid;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy