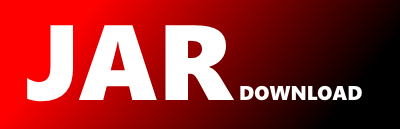
com.pulumi.aws.rds.ClusterInstance Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.rds;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.rds.ClusterInstanceArgs;
import com.pulumi.aws.rds.inputs.ClusterInstanceState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an RDS Cluster Instance Resource. A Cluster Instance Resource defines
* attributes that are specific to a single instance in a RDS Cluster,
* specifically running Amazon Aurora.
*
* Unlike other RDS resources that support replication, with Amazon Aurora you do
* not designate a primary and subsequent replicas. Instead, you simply add RDS
* Instances and Aurora manages the replication. You can use the [count][5]
* meta-parameter to make multiple instances and join them all to the same RDS
* Cluster, or you may specify different Cluster Instance resources with various
* `instance_class` sizes.
*
* For more information on Amazon Aurora, see [Aurora on Amazon RDS](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/CHAP_Aurora.html) in the Amazon RDS User Guide.
*
* > **NOTE:** Deletion Protection from the RDS service can only be enabled at the cluster level, not for individual cluster instances. You can still add the [`protect` CustomResourceOption](https://www.pulumi.com/docs/intro/concepts/programming-model/#protect) to this resource configuration if you desire protection from accidental deletion.
*
* > **NOTE:** `aurora` is no longer a valid `engine` because of [Amazon Aurora's MySQL-Compatible Edition version 1 end of life](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/Aurora.MySQL56.EOL.html).
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Cluster;
* import com.pulumi.aws.rds.ClusterArgs;
* import com.pulumi.aws.rds.ClusterInstance;
* import com.pulumi.aws.rds.ClusterInstanceArgs;
* import com.pulumi.codegen.internal.KeyedValue;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var default_ = new Cluster("default", ClusterArgs.builder()
* .clusterIdentifier("aurora-cluster-demo")
* .availabilityZones(
* "us-west-2a",
* "us-west-2b",
* "us-west-2c")
* .databaseName("mydb")
* .masterUsername("foo")
* .masterPassword("barbut8chars")
* .build());
*
* for (var i = 0; i < 2; i++) {
* new ClusterInstance("clusterInstances-" + i, ClusterInstanceArgs.builder()
* .identifier(String.format("aurora-cluster-demo-%s", range.value()))
* .clusterIdentifier(default_.id())
* .instanceClass("db.r4.large")
* .engine(default_.engine())
* .engineVersion(default_.engineVersion())
* .build());
*
*
* }
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import RDS Cluster Instances using the `identifier`. For example:
*
* ```sh
* $ pulumi import aws:rds/clusterInstance:ClusterInstance prod_instance_1 aurora-cluster-instance-1
* ```
*
*/
@ResourceType(type="aws:rds/clusterInstance:ClusterInstance")
public class ClusterInstance extends com.pulumi.resources.CustomResource {
/**
* Specifies whether any database modifications are applied immediately, or during the next maintenance window. Default is`false`.
*
*/
@Export(name="applyImmediately", refs={Boolean.class}, tree="[0]")
private Output applyImmediately;
/**
* @return Specifies whether any database modifications are applied immediately, or during the next maintenance window. Default is`false`.
*
*/
public Output applyImmediately() {
return this.applyImmediately;
}
/**
* Amazon Resource Name (ARN) of cluster instance
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return Amazon Resource Name (ARN) of cluster instance
*
*/
public Output arn() {
return this.arn;
}
/**
* Indicates that minor engine upgrades will be applied automatically to the DB instance during the maintenance window. Default `true`.
*
*/
@Export(name="autoMinorVersionUpgrade", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> autoMinorVersionUpgrade;
/**
* @return Indicates that minor engine upgrades will be applied automatically to the DB instance during the maintenance window. Default `true`.
*
*/
public Output> autoMinorVersionUpgrade() {
return Codegen.optional(this.autoMinorVersionUpgrade);
}
/**
* EC2 Availability Zone that the DB instance is created in. See [docs](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_CreateDBInstance.html) about the details.
*
*/
@Export(name="availabilityZone", refs={String.class}, tree="[0]")
private Output availabilityZone;
/**
* @return EC2 Availability Zone that the DB instance is created in. See [docs](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_CreateDBInstance.html) about the details.
*
*/
public Output availabilityZone() {
return this.availabilityZone;
}
/**
* Identifier of the CA certificate for the DB instance.
*
*/
@Export(name="caCertIdentifier", refs={String.class}, tree="[0]")
private Output caCertIdentifier;
/**
* @return Identifier of the CA certificate for the DB instance.
*
*/
public Output caCertIdentifier() {
return this.caCertIdentifier;
}
/**
* Identifier of the `aws.rds.Cluster` in which to launch this instance.
*
*/
@Export(name="clusterIdentifier", refs={String.class}, tree="[0]")
private Output clusterIdentifier;
/**
* @return Identifier of the `aws.rds.Cluster` in which to launch this instance.
*
*/
public Output clusterIdentifier() {
return this.clusterIdentifier;
}
/**
* Indicates whether to copy all of the user-defined tags from the DB instance to snapshots of the DB instance. Default `false`.
*
*/
@Export(name="copyTagsToSnapshot", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> copyTagsToSnapshot;
/**
* @return Indicates whether to copy all of the user-defined tags from the DB instance to snapshots of the DB instance. Default `false`.
*
*/
public Output> copyTagsToSnapshot() {
return Codegen.optional(this.copyTagsToSnapshot);
}
/**
* Instance profile associated with the underlying Amazon EC2 instance of an RDS Custom DB instance.
*
*/
@Export(name="customIamInstanceProfile", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> customIamInstanceProfile;
/**
* @return Instance profile associated with the underlying Amazon EC2 instance of an RDS Custom DB instance.
*
*/
public Output> customIamInstanceProfile() {
return Codegen.optional(this.customIamInstanceProfile);
}
/**
* Name of the DB parameter group to associate with this instance.
*
*/
@Export(name="dbParameterGroupName", refs={String.class}, tree="[0]")
private Output dbParameterGroupName;
/**
* @return Name of the DB parameter group to associate with this instance.
*
*/
public Output dbParameterGroupName() {
return this.dbParameterGroupName;
}
/**
* Specifies the DB subnet group to associate with this DB instance. The default behavior varies depending on whether `db_subnet_group_name` is specified. Please refer to official [AWS documentation](https://docs.aws.amazon.com/cli/latest/reference/rds/create-db-instance.html) to understand how `db_subnet_group_name` and `publicly_accessible` parameters affect DB instance behaviour. **NOTE:** This must match the `db_subnet_group_name` of the attached `aws.rds.Cluster`.
*
*/
@Export(name="dbSubnetGroupName", refs={String.class}, tree="[0]")
private Output dbSubnetGroupName;
/**
* @return Specifies the DB subnet group to associate with this DB instance. The default behavior varies depending on whether `db_subnet_group_name` is specified. Please refer to official [AWS documentation](https://docs.aws.amazon.com/cli/latest/reference/rds/create-db-instance.html) to understand how `db_subnet_group_name` and `publicly_accessible` parameters affect DB instance behaviour. **NOTE:** This must match the `db_subnet_group_name` of the attached `aws.rds.Cluster`.
*
*/
public Output dbSubnetGroupName() {
return this.dbSubnetGroupName;
}
/**
* Region-unique, immutable identifier for the DB instance.
*
*/
@Export(name="dbiResourceId", refs={String.class}, tree="[0]")
private Output dbiResourceId;
/**
* @return Region-unique, immutable identifier for the DB instance.
*
*/
public Output dbiResourceId() {
return this.dbiResourceId;
}
/**
* DNS address for this instance. May not be writable
*
*/
@Export(name="endpoint", refs={String.class}, tree="[0]")
private Output endpoint;
/**
* @return DNS address for this instance. May not be writable
*
*/
public Output endpoint() {
return this.endpoint;
}
/**
* Name of the database engine to be used for the RDS cluster instance.
* Valid Values: `aurora-mysql`, `aurora-postgresql`, `mysql`, `postgres`.(Note that `mysql` and `postgres` are Multi-AZ RDS clusters).
*
*/
@Export(name="engine", refs={String.class}, tree="[0]")
private Output engine;
/**
* @return Name of the database engine to be used for the RDS cluster instance.
* Valid Values: `aurora-mysql`, `aurora-postgresql`, `mysql`, `postgres`.(Note that `mysql` and `postgres` are Multi-AZ RDS clusters).
*
*/
public Output engine() {
return this.engine;
}
/**
* Database engine version. Please note that to upgrade the `engine_version` of the instance, it must be done on the `aws.rds.Cluster` `engine_version`. Trying to upgrade in `aws_cluster_instance` will not update the `engine_version`.
*
*/
@Export(name="engineVersion", refs={String.class}, tree="[0]")
private Output engineVersion;
/**
* @return Database engine version. Please note that to upgrade the `engine_version` of the instance, it must be done on the `aws.rds.Cluster` `engine_version`. Trying to upgrade in `aws_cluster_instance` will not update the `engine_version`.
*
*/
public Output engineVersion() {
return this.engineVersion;
}
/**
* Database engine version
*
*/
@Export(name="engineVersionActual", refs={String.class}, tree="[0]")
private Output engineVersionActual;
/**
* @return Database engine version
*
*/
public Output engineVersionActual() {
return this.engineVersionActual;
}
/**
* Identifier for the RDS instance, if omitted, Pulumi will assign a random, unique identifier.
*
*/
@Export(name="identifier", refs={String.class}, tree="[0]")
private Output identifier;
/**
* @return Identifier for the RDS instance, if omitted, Pulumi will assign a random, unique identifier.
*
*/
public Output identifier() {
return this.identifier;
}
/**
* Creates a unique identifier beginning with the specified prefix. Conflicts with `identifier`.
*
*/
@Export(name="identifierPrefix", refs={String.class}, tree="[0]")
private Output identifierPrefix;
/**
* @return Creates a unique identifier beginning with the specified prefix. Conflicts with `identifier`.
*
*/
public Output identifierPrefix() {
return this.identifierPrefix;
}
/**
* Instance class to use. For details on CPU and memory, see [Scaling Aurora DB Instances](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Aurora.Managing.html). Aurora uses `db.*` instance classes/types. Please see [AWS Documentation](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Concepts.DBInstanceClass.html) for currently available instance classes and complete details. For Aurora Serverless v2 use `db.serverless`.
*
*/
@Export(name="instanceClass", refs={String.class}, tree="[0]")
private Output instanceClass;
/**
* @return Instance class to use. For details on CPU and memory, see [Scaling Aurora DB Instances](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Aurora.Managing.html). Aurora uses `db.*` instance classes/types. Please see [AWS Documentation](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Concepts.DBInstanceClass.html) for currently available instance classes and complete details. For Aurora Serverless v2 use `db.serverless`.
*
*/
public Output instanceClass() {
return this.instanceClass;
}
/**
* ARN for the KMS encryption key if one is set to the cluster.
*
*/
@Export(name="kmsKeyId", refs={String.class}, tree="[0]")
private Output kmsKeyId;
/**
* @return ARN for the KMS encryption key if one is set to the cluster.
*
*/
public Output kmsKeyId() {
return this.kmsKeyId;
}
/**
* Interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB instance. To disable collecting Enhanced Monitoring metrics, specify 0. The default is 0. Valid Values: 0, 1, 5, 10, 15, 30, 60.
*
*/
@Export(name="monitoringInterval", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> monitoringInterval;
/**
* @return Interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB instance. To disable collecting Enhanced Monitoring metrics, specify 0. The default is 0. Valid Values: 0, 1, 5, 10, 15, 30, 60.
*
*/
public Output> monitoringInterval() {
return Codegen.optional(this.monitoringInterval);
}
/**
* ARN for the IAM role that permits RDS to send enhanced monitoring metrics to CloudWatch Logs. You can find more information on the [AWS Documentation](http://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_Monitoring.html) what IAM permissions are needed to allow Enhanced Monitoring for RDS Instances.
*
*/
@Export(name="monitoringRoleArn", refs={String.class}, tree="[0]")
private Output monitoringRoleArn;
/**
* @return ARN for the IAM role that permits RDS to send enhanced monitoring metrics to CloudWatch Logs. You can find more information on the [AWS Documentation](http://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_Monitoring.html) what IAM permissions are needed to allow Enhanced Monitoring for RDS Instances.
*
*/
public Output monitoringRoleArn() {
return this.monitoringRoleArn;
}
/**
* Network type of the DB instance.
*
*/
@Export(name="networkType", refs={String.class}, tree="[0]")
private Output networkType;
/**
* @return Network type of the DB instance.
*
*/
public Output networkType() {
return this.networkType;
}
/**
* Specifies whether Performance Insights is enabled or not.
*
*/
@Export(name="performanceInsightsEnabled", refs={Boolean.class}, tree="[0]")
private Output performanceInsightsEnabled;
/**
* @return Specifies whether Performance Insights is enabled or not.
*
*/
public Output performanceInsightsEnabled() {
return this.performanceInsightsEnabled;
}
/**
* ARN for the KMS key to encrypt Performance Insights data. When specifying `performance_insights_kms_key_id`, `performance_insights_enabled` needs to be set to true.
*
*/
@Export(name="performanceInsightsKmsKeyId", refs={String.class}, tree="[0]")
private Output performanceInsightsKmsKeyId;
/**
* @return ARN for the KMS key to encrypt Performance Insights data. When specifying `performance_insights_kms_key_id`, `performance_insights_enabled` needs to be set to true.
*
*/
public Output performanceInsightsKmsKeyId() {
return this.performanceInsightsKmsKeyId;
}
/**
* Amount of time in days to retain Performance Insights data. Valid values are `7`, `731` (2 years) or a multiple of `31`. When specifying `performance_insights_retention_period`, `performance_insights_enabled` needs to be set to true. Defaults to '7'.
*
*/
@Export(name="performanceInsightsRetentionPeriod", refs={Integer.class}, tree="[0]")
private Output performanceInsightsRetentionPeriod;
/**
* @return Amount of time in days to retain Performance Insights data. Valid values are `7`, `731` (2 years) or a multiple of `31`. When specifying `performance_insights_retention_period`, `performance_insights_enabled` needs to be set to true. Defaults to '7'.
*
*/
public Output performanceInsightsRetentionPeriod() {
return this.performanceInsightsRetentionPeriod;
}
/**
* Database port
*
*/
@Export(name="port", refs={Integer.class}, tree="[0]")
private Output port;
/**
* @return Database port
*
*/
public Output port() {
return this.port;
}
/**
* Daily time range during which automated backups are created if automated backups are enabled. Eg: "04:00-09:00". **NOTE:** If `preferred_backup_window` is set at the cluster level, this argument **must** be omitted.
*
*/
@Export(name="preferredBackupWindow", refs={String.class}, tree="[0]")
private Output preferredBackupWindow;
/**
* @return Daily time range during which automated backups are created if automated backups are enabled. Eg: "04:00-09:00". **NOTE:** If `preferred_backup_window` is set at the cluster level, this argument **must** be omitted.
*
*/
public Output preferredBackupWindow() {
return this.preferredBackupWindow;
}
/**
* Window to perform maintenance in. Syntax: "ddd:hh24:mi-ddd:hh24:mi". Eg: "Mon:00:00-Mon:03:00".
*
*/
@Export(name="preferredMaintenanceWindow", refs={String.class}, tree="[0]")
private Output preferredMaintenanceWindow;
/**
* @return Window to perform maintenance in. Syntax: "ddd:hh24:mi-ddd:hh24:mi". Eg: "Mon:00:00-Mon:03:00".
*
*/
public Output preferredMaintenanceWindow() {
return this.preferredMaintenanceWindow;
}
/**
* Default 0. Failover Priority setting on instance level. The reader who has lower tier has higher priority to get promoted to writer.
*
*/
@Export(name="promotionTier", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> promotionTier;
/**
* @return Default 0. Failover Priority setting on instance level. The reader who has lower tier has higher priority to get promoted to writer.
*
*/
public Output> promotionTier() {
return Codegen.optional(this.promotionTier);
}
/**
* Bool to control if instance is publicly accessible. Default `false`. See the documentation on [Creating DB Instances](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_CreateDBInstance.html) for more details on controlling this property.
*
*/
@Export(name="publiclyAccessible", refs={Boolean.class}, tree="[0]")
private Output publiclyAccessible;
/**
* @return Bool to control if instance is publicly accessible. Default `false`. See the documentation on [Creating DB Instances](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_CreateDBInstance.html) for more details on controlling this property.
*
*/
public Output publiclyAccessible() {
return this.publiclyAccessible;
}
/**
* Specifies whether the DB cluster is encrypted.
*
*/
@Export(name="storageEncrypted", refs={Boolean.class}, tree="[0]")
private Output storageEncrypted;
/**
* @return Specifies whether the DB cluster is encrypted.
*
*/
public Output storageEncrypted() {
return this.storageEncrypted;
}
/**
* Map of tags to assign to the instance. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Map of tags to assign to the instance. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy