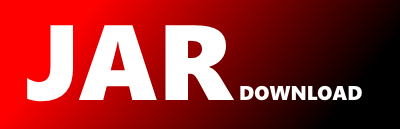
com.pulumi.aws.rds.inputs.GetEngineVersionPlainArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.rds.inputs;
import com.pulumi.aws.rds.inputs.GetEngineVersionFilter;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetEngineVersionPlainArgs extends com.pulumi.resources.InvokeArgs {
public static final GetEngineVersionPlainArgs Empty = new GetEngineVersionPlainArgs();
/**
* Whether the engine version must be an AWS-defined default version. Some engines have multiple default versions, such as for each major version. Using `default_only` may help avoid `multiple RDS engine versions` errors. See also `latest`.
*
*/
@Import(name="defaultOnly")
private @Nullable Boolean defaultOnly;
/**
* @return Whether the engine version must be an AWS-defined default version. Some engines have multiple default versions, such as for each major version. Using `default_only` may help avoid `multiple RDS engine versions` errors. See also `latest`.
*
*/
public Optional defaultOnly() {
return Optional.ofNullable(this.defaultOnly);
}
/**
* Database engine. Engine values include `aurora`, `aurora-mysql`, `aurora-postgresql`, `docdb`, `mariadb`, `mysql`, `neptune`, `oracle-ee`, `oracle-se`, `oracle-se1`, `oracle-se2`, `postgres`, `sqlserver-ee`, `sqlserver-ex`, `sqlserver-se`, and `sqlserver-web`.
*
* The following arguments are optional:
*
*/
@Import(name="engine", required=true)
private String engine;
/**
* @return Database engine. Engine values include `aurora`, `aurora-mysql`, `aurora-postgresql`, `docdb`, `mariadb`, `mysql`, `neptune`, `oracle-ee`, `oracle-se`, `oracle-se1`, `oracle-se2`, `postgres`, `sqlserver-ee`, `sqlserver-ex`, `sqlserver-se`, and `sqlserver-web`.
*
* The following arguments are optional:
*
*/
public String engine() {
return this.engine;
}
/**
* One or more name/value pairs to use in filtering versions. There are several valid keys; for a full reference, check out [describe-db-engine-versions in the AWS CLI reference](https://awscli.amazonaws.com/v2/documentation/api/latest/reference/rds/describe-db-engine-versions.html).
*
*/
@Import(name="filters")
private @Nullable List filters;
/**
* @return One or more name/value pairs to use in filtering versions. There are several valid keys; for a full reference, check out [describe-db-engine-versions in the AWS CLI reference](https://awscli.amazonaws.com/v2/documentation/api/latest/reference/rds/describe-db-engine-versions.html).
*
*/
public Optional> filters() {
return Optional.ofNullable(this.filters);
}
/**
* Whether the engine version must have one or more major upgrade targets. Not including `has_major_target` or setting it to `false` doesn't imply that there's no corresponding major upgrade target for the engine version.
*
*/
@Import(name="hasMajorTarget")
private @Nullable Boolean hasMajorTarget;
/**
* @return Whether the engine version must have one or more major upgrade targets. Not including `has_major_target` or setting it to `false` doesn't imply that there's no corresponding major upgrade target for the engine version.
*
*/
public Optional hasMajorTarget() {
return Optional.ofNullable(this.hasMajorTarget);
}
/**
* Whether the engine version must have one or more minor upgrade targets. Not including `has_minor_target` or setting it to `false` doesn't imply that there's no corresponding minor upgrade target for the engine version.
*
*/
@Import(name="hasMinorTarget")
private @Nullable Boolean hasMinorTarget;
/**
* @return Whether the engine version must have one or more minor upgrade targets. Not including `has_minor_target` or setting it to `false` doesn't imply that there's no corresponding minor upgrade target for the engine version.
*
*/
public Optional hasMinorTarget() {
return Optional.ofNullable(this.hasMinorTarget);
}
/**
* Whether the engine version `status` can either be `deprecated` or `available`. When not set or set to `false`, the engine version `status` will always be `available`.
*
*/
@Import(name="includeAll")
private @Nullable Boolean includeAll;
/**
* @return Whether the engine version `status` can either be `deprecated` or `available`. When not set or set to `false`, the engine version `status` will always be `available`.
*
*/
public Optional includeAll() {
return Optional.ofNullable(this.includeAll);
}
/**
* Whether the engine version is the most recent version matching the other criteria. This is different from `default_only` in important ways: "default" relies on AWS-defined defaults, the latest version isn't always the default, and AWS might have multiple default versions for an engine. As a result, `default_only` might not prevent errors from `multiple RDS engine versions`, while `latest` will. (`latest` can be used with `default_only`.) **Note:** The data source uses a best-effort approach at selecting the latest version. Due to the complexity of version identifiers across engines and incomplete version date information provided by AWS, using `latest` may not always result in the engine version being the actual latest version.
*
*/
@Import(name="latest")
private @Nullable Boolean latest;
/**
* @return Whether the engine version is the most recent version matching the other criteria. This is different from `default_only` in important ways: "default" relies on AWS-defined defaults, the latest version isn't always the default, and AWS might have multiple default versions for an engine. As a result, `default_only` might not prevent errors from `multiple RDS engine versions`, while `latest` will. (`latest` can be used with `default_only`.) **Note:** The data source uses a best-effort approach at selecting the latest version. Due to the complexity of version identifiers across engines and incomplete version date information provided by AWS, using `latest` may not always result in the engine version being the actual latest version.
*
*/
public Optional latest() {
return Optional.ofNullable(this.latest);
}
/**
* Name of a specific database parameter group family. Examples of parameter group families are `mysql8.0`, `mariadb10.4`, and `postgres12`.
*
*/
@Import(name="parameterGroupFamily")
private @Nullable String parameterGroupFamily;
/**
* @return Name of a specific database parameter group family. Examples of parameter group families are `mysql8.0`, `mariadb10.4`, and `postgres12`.
*
*/
public Optional parameterGroupFamily() {
return Optional.ofNullable(this.parameterGroupFamily);
}
/**
* Ordered list of preferred major version upgrade targets. The engine version will be the first match in the list unless the `latest` parameter is set to `true`. The engine version will be the default version if you don't include any criteria, such as `preferred_major_targets`.
*
*/
@Import(name="preferredMajorTargets")
private @Nullable List preferredMajorTargets;
/**
* @return Ordered list of preferred major version upgrade targets. The engine version will be the first match in the list unless the `latest` parameter is set to `true`. The engine version will be the default version if you don't include any criteria, such as `preferred_major_targets`.
*
*/
public Optional> preferredMajorTargets() {
return Optional.ofNullable(this.preferredMajorTargets);
}
/**
* Ordered list of preferred version upgrade targets. The engine version will be the first match in this list unless the `latest` parameter is set to `true`. The engine version will be the default version if you don't include any criteria, such as `preferred_upgrade_targets`.
*
*/
@Import(name="preferredUpgradeTargets")
private @Nullable List preferredUpgradeTargets;
/**
* @return Ordered list of preferred version upgrade targets. The engine version will be the first match in this list unless the `latest` parameter is set to `true`. The engine version will be the default version if you don't include any criteria, such as `preferred_upgrade_targets`.
*
*/
public Optional> preferredUpgradeTargets() {
return Optional.ofNullable(this.preferredUpgradeTargets);
}
/**
* Ordered list of preferred versions. The engine version will be the first match in this list unless the `latest` parameter is set to `true`. The engine version will be the default version if you don't include any criteria, such as `preferred_versions`.
*
*/
@Import(name="preferredVersions")
private @Nullable List preferredVersions;
/**
* @return Ordered list of preferred versions. The engine version will be the first match in this list unless the `latest` parameter is set to `true`. The engine version will be the default version if you don't include any criteria, such as `preferred_versions`.
*
*/
public Optional> preferredVersions() {
return Optional.ofNullable(this.preferredVersions);
}
@Import(name="version")
private @Nullable String version;
public Optional version() {
return Optional.ofNullable(this.version);
}
private GetEngineVersionPlainArgs() {}
private GetEngineVersionPlainArgs(GetEngineVersionPlainArgs $) {
this.defaultOnly = $.defaultOnly;
this.engine = $.engine;
this.filters = $.filters;
this.hasMajorTarget = $.hasMajorTarget;
this.hasMinorTarget = $.hasMinorTarget;
this.includeAll = $.includeAll;
this.latest = $.latest;
this.parameterGroupFamily = $.parameterGroupFamily;
this.preferredMajorTargets = $.preferredMajorTargets;
this.preferredUpgradeTargets = $.preferredUpgradeTargets;
this.preferredVersions = $.preferredVersions;
this.version = $.version;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetEngineVersionPlainArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetEngineVersionPlainArgs $;
public Builder() {
$ = new GetEngineVersionPlainArgs();
}
public Builder(GetEngineVersionPlainArgs defaults) {
$ = new GetEngineVersionPlainArgs(Objects.requireNonNull(defaults));
}
/**
* @param defaultOnly Whether the engine version must be an AWS-defined default version. Some engines have multiple default versions, such as for each major version. Using `default_only` may help avoid `multiple RDS engine versions` errors. See also `latest`.
*
* @return builder
*
*/
public Builder defaultOnly(@Nullable Boolean defaultOnly) {
$.defaultOnly = defaultOnly;
return this;
}
/**
* @param engine Database engine. Engine values include `aurora`, `aurora-mysql`, `aurora-postgresql`, `docdb`, `mariadb`, `mysql`, `neptune`, `oracle-ee`, `oracle-se`, `oracle-se1`, `oracle-se2`, `postgres`, `sqlserver-ee`, `sqlserver-ex`, `sqlserver-se`, and `sqlserver-web`.
*
* The following arguments are optional:
*
* @return builder
*
*/
public Builder engine(String engine) {
$.engine = engine;
return this;
}
/**
* @param filters One or more name/value pairs to use in filtering versions. There are several valid keys; for a full reference, check out [describe-db-engine-versions in the AWS CLI reference](https://awscli.amazonaws.com/v2/documentation/api/latest/reference/rds/describe-db-engine-versions.html).
*
* @return builder
*
*/
public Builder filters(@Nullable List filters) {
$.filters = filters;
return this;
}
/**
* @param filters One or more name/value pairs to use in filtering versions. There are several valid keys; for a full reference, check out [describe-db-engine-versions in the AWS CLI reference](https://awscli.amazonaws.com/v2/documentation/api/latest/reference/rds/describe-db-engine-versions.html).
*
* @return builder
*
*/
public Builder filters(GetEngineVersionFilter... filters) {
return filters(List.of(filters));
}
/**
* @param hasMajorTarget Whether the engine version must have one or more major upgrade targets. Not including `has_major_target` or setting it to `false` doesn't imply that there's no corresponding major upgrade target for the engine version.
*
* @return builder
*
*/
public Builder hasMajorTarget(@Nullable Boolean hasMajorTarget) {
$.hasMajorTarget = hasMajorTarget;
return this;
}
/**
* @param hasMinorTarget Whether the engine version must have one or more minor upgrade targets. Not including `has_minor_target` or setting it to `false` doesn't imply that there's no corresponding minor upgrade target for the engine version.
*
* @return builder
*
*/
public Builder hasMinorTarget(@Nullable Boolean hasMinorTarget) {
$.hasMinorTarget = hasMinorTarget;
return this;
}
/**
* @param includeAll Whether the engine version `status` can either be `deprecated` or `available`. When not set or set to `false`, the engine version `status` will always be `available`.
*
* @return builder
*
*/
public Builder includeAll(@Nullable Boolean includeAll) {
$.includeAll = includeAll;
return this;
}
/**
* @param latest Whether the engine version is the most recent version matching the other criteria. This is different from `default_only` in important ways: "default" relies on AWS-defined defaults, the latest version isn't always the default, and AWS might have multiple default versions for an engine. As a result, `default_only` might not prevent errors from `multiple RDS engine versions`, while `latest` will. (`latest` can be used with `default_only`.) **Note:** The data source uses a best-effort approach at selecting the latest version. Due to the complexity of version identifiers across engines and incomplete version date information provided by AWS, using `latest` may not always result in the engine version being the actual latest version.
*
* @return builder
*
*/
public Builder latest(@Nullable Boolean latest) {
$.latest = latest;
return this;
}
/**
* @param parameterGroupFamily Name of a specific database parameter group family. Examples of parameter group families are `mysql8.0`, `mariadb10.4`, and `postgres12`.
*
* @return builder
*
*/
public Builder parameterGroupFamily(@Nullable String parameterGroupFamily) {
$.parameterGroupFamily = parameterGroupFamily;
return this;
}
/**
* @param preferredMajorTargets Ordered list of preferred major version upgrade targets. The engine version will be the first match in the list unless the `latest` parameter is set to `true`. The engine version will be the default version if you don't include any criteria, such as `preferred_major_targets`.
*
* @return builder
*
*/
public Builder preferredMajorTargets(@Nullable List preferredMajorTargets) {
$.preferredMajorTargets = preferredMajorTargets;
return this;
}
/**
* @param preferredMajorTargets Ordered list of preferred major version upgrade targets. The engine version will be the first match in the list unless the `latest` parameter is set to `true`. The engine version will be the default version if you don't include any criteria, such as `preferred_major_targets`.
*
* @return builder
*
*/
public Builder preferredMajorTargets(String... preferredMajorTargets) {
return preferredMajorTargets(List.of(preferredMajorTargets));
}
/**
* @param preferredUpgradeTargets Ordered list of preferred version upgrade targets. The engine version will be the first match in this list unless the `latest` parameter is set to `true`. The engine version will be the default version if you don't include any criteria, such as `preferred_upgrade_targets`.
*
* @return builder
*
*/
public Builder preferredUpgradeTargets(@Nullable List preferredUpgradeTargets) {
$.preferredUpgradeTargets = preferredUpgradeTargets;
return this;
}
/**
* @param preferredUpgradeTargets Ordered list of preferred version upgrade targets. The engine version will be the first match in this list unless the `latest` parameter is set to `true`. The engine version will be the default version if you don't include any criteria, such as `preferred_upgrade_targets`.
*
* @return builder
*
*/
public Builder preferredUpgradeTargets(String... preferredUpgradeTargets) {
return preferredUpgradeTargets(List.of(preferredUpgradeTargets));
}
/**
* @param preferredVersions Ordered list of preferred versions. The engine version will be the first match in this list unless the `latest` parameter is set to `true`. The engine version will be the default version if you don't include any criteria, such as `preferred_versions`.
*
* @return builder
*
*/
public Builder preferredVersions(@Nullable List preferredVersions) {
$.preferredVersions = preferredVersions;
return this;
}
/**
* @param preferredVersions Ordered list of preferred versions. The engine version will be the first match in this list unless the `latest` parameter is set to `true`. The engine version will be the default version if you don't include any criteria, such as `preferred_versions`.
*
* @return builder
*
*/
public Builder preferredVersions(String... preferredVersions) {
return preferredVersions(List.of(preferredVersions));
}
public Builder version(@Nullable String version) {
$.version = version;
return this;
}
public GetEngineVersionPlainArgs build() {
if ($.engine == null) {
throw new MissingRequiredPropertyException("GetEngineVersionPlainArgs", "engine");
}
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy