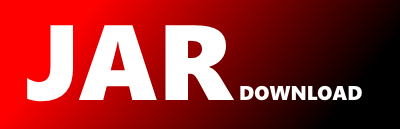
com.pulumi.aws.redshiftserverless.inputs.UsageLimitState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.redshiftserverless.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class UsageLimitState extends com.pulumi.resources.ResourceArgs {
public static final UsageLimitState Empty = new UsageLimitState();
/**
* The limit amount. If time-based, this amount is in Redshift Processing Units (RPU) consumed per hour. If data-based, this amount is in terabytes (TB) of data transferred between Regions in cross-account sharing. The value must be a positive number.
*
*/
@Import(name="amount")
private @Nullable Output amount;
/**
* @return The limit amount. If time-based, this amount is in Redshift Processing Units (RPU) consumed per hour. If data-based, this amount is in terabytes (TB) of data transferred between Regions in cross-account sharing. The value must be a positive number.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy