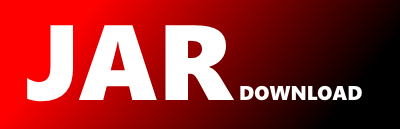
com.pulumi.aws.route53.outputs.GetResolverFirewallRuleGroupAssociationResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.route53.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetResolverFirewallRuleGroupAssociationResult {
private String arn;
private String creationTime;
private String creatorRequestId;
private String firewallRuleGroupAssociationId;
private String firewallRuleGroupId;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private String managedOwnerName;
private String modificationTime;
private String mutationProtection;
private String name;
private Integer priority;
private String status;
private String statusMessage;
private String vpcId;
private GetResolverFirewallRuleGroupAssociationResult() {}
public String arn() {
return this.arn;
}
public String creationTime() {
return this.creationTime;
}
public String creatorRequestId() {
return this.creatorRequestId;
}
public String firewallRuleGroupAssociationId() {
return this.firewallRuleGroupAssociationId;
}
public String firewallRuleGroupId() {
return this.firewallRuleGroupId;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public String managedOwnerName() {
return this.managedOwnerName;
}
public String modificationTime() {
return this.modificationTime;
}
public String mutationProtection() {
return this.mutationProtection;
}
public String name() {
return this.name;
}
public Integer priority() {
return this.priority;
}
public String status() {
return this.status;
}
public String statusMessage() {
return this.statusMessage;
}
public String vpcId() {
return this.vpcId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetResolverFirewallRuleGroupAssociationResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private String creationTime;
private String creatorRequestId;
private String firewallRuleGroupAssociationId;
private String firewallRuleGroupId;
private String id;
private String managedOwnerName;
private String modificationTime;
private String mutationProtection;
private String name;
private Integer priority;
private String status;
private String statusMessage;
private String vpcId;
public Builder() {}
public Builder(GetResolverFirewallRuleGroupAssociationResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.creationTime = defaults.creationTime;
this.creatorRequestId = defaults.creatorRequestId;
this.firewallRuleGroupAssociationId = defaults.firewallRuleGroupAssociationId;
this.firewallRuleGroupId = defaults.firewallRuleGroupId;
this.id = defaults.id;
this.managedOwnerName = defaults.managedOwnerName;
this.modificationTime = defaults.modificationTime;
this.mutationProtection = defaults.mutationProtection;
this.name = defaults.name;
this.priority = defaults.priority;
this.status = defaults.status;
this.statusMessage = defaults.statusMessage;
this.vpcId = defaults.vpcId;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder creationTime(String creationTime) {
if (creationTime == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "creationTime");
}
this.creationTime = creationTime;
return this;
}
@CustomType.Setter
public Builder creatorRequestId(String creatorRequestId) {
if (creatorRequestId == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "creatorRequestId");
}
this.creatorRequestId = creatorRequestId;
return this;
}
@CustomType.Setter
public Builder firewallRuleGroupAssociationId(String firewallRuleGroupAssociationId) {
if (firewallRuleGroupAssociationId == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "firewallRuleGroupAssociationId");
}
this.firewallRuleGroupAssociationId = firewallRuleGroupAssociationId;
return this;
}
@CustomType.Setter
public Builder firewallRuleGroupId(String firewallRuleGroupId) {
if (firewallRuleGroupId == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "firewallRuleGroupId");
}
this.firewallRuleGroupId = firewallRuleGroupId;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder managedOwnerName(String managedOwnerName) {
if (managedOwnerName == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "managedOwnerName");
}
this.managedOwnerName = managedOwnerName;
return this;
}
@CustomType.Setter
public Builder modificationTime(String modificationTime) {
if (modificationTime == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "modificationTime");
}
this.modificationTime = modificationTime;
return this;
}
@CustomType.Setter
public Builder mutationProtection(String mutationProtection) {
if (mutationProtection == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "mutationProtection");
}
this.mutationProtection = mutationProtection;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder priority(Integer priority) {
if (priority == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "priority");
}
this.priority = priority;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder statusMessage(String statusMessage) {
if (statusMessage == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "statusMessage");
}
this.statusMessage = statusMessage;
return this;
}
@CustomType.Setter
public Builder vpcId(String vpcId) {
if (vpcId == null) {
throw new MissingRequiredPropertyException("GetResolverFirewallRuleGroupAssociationResult", "vpcId");
}
this.vpcId = vpcId;
return this;
}
public GetResolverFirewallRuleGroupAssociationResult build() {
final var _resultValue = new GetResolverFirewallRuleGroupAssociationResult();
_resultValue.arn = arn;
_resultValue.creationTime = creationTime;
_resultValue.creatorRequestId = creatorRequestId;
_resultValue.firewallRuleGroupAssociationId = firewallRuleGroupAssociationId;
_resultValue.firewallRuleGroupId = firewallRuleGroupId;
_resultValue.id = id;
_resultValue.managedOwnerName = managedOwnerName;
_resultValue.modificationTime = modificationTime;
_resultValue.mutationProtection = mutationProtection;
_resultValue.name = name;
_resultValue.priority = priority;
_resultValue.status = status;
_resultValue.statusMessage = statusMessage;
_resultValue.vpcId = vpcId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy