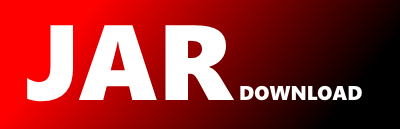
com.pulumi.aws.route53.outputs.GetResolverRulesResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.route53.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetResolverRulesResult {
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private @Nullable String nameRegex;
private @Nullable String ownerId;
private @Nullable String resolverEndpointId;
/**
* @return IDs of the matched resolver rules.
*
*/
private List resolverRuleIds;
private @Nullable String ruleType;
private @Nullable String shareStatus;
private GetResolverRulesResult() {}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public Optional nameRegex() {
return Optional.ofNullable(this.nameRegex);
}
public Optional ownerId() {
return Optional.ofNullable(this.ownerId);
}
public Optional resolverEndpointId() {
return Optional.ofNullable(this.resolverEndpointId);
}
/**
* @return IDs of the matched resolver rules.
*
*/
public List resolverRuleIds() {
return this.resolverRuleIds;
}
public Optional ruleType() {
return Optional.ofNullable(this.ruleType);
}
public Optional shareStatus() {
return Optional.ofNullable(this.shareStatus);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetResolverRulesResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String id;
private @Nullable String nameRegex;
private @Nullable String ownerId;
private @Nullable String resolverEndpointId;
private List resolverRuleIds;
private @Nullable String ruleType;
private @Nullable String shareStatus;
public Builder() {}
public Builder(GetResolverRulesResult defaults) {
Objects.requireNonNull(defaults);
this.id = defaults.id;
this.nameRegex = defaults.nameRegex;
this.ownerId = defaults.ownerId;
this.resolverEndpointId = defaults.resolverEndpointId;
this.resolverRuleIds = defaults.resolverRuleIds;
this.ruleType = defaults.ruleType;
this.shareStatus = defaults.shareStatus;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetResolverRulesResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder nameRegex(@Nullable String nameRegex) {
this.nameRegex = nameRegex;
return this;
}
@CustomType.Setter
public Builder ownerId(@Nullable String ownerId) {
this.ownerId = ownerId;
return this;
}
@CustomType.Setter
public Builder resolverEndpointId(@Nullable String resolverEndpointId) {
this.resolverEndpointId = resolverEndpointId;
return this;
}
@CustomType.Setter
public Builder resolverRuleIds(List resolverRuleIds) {
if (resolverRuleIds == null) {
throw new MissingRequiredPropertyException("GetResolverRulesResult", "resolverRuleIds");
}
this.resolverRuleIds = resolverRuleIds;
return this;
}
public Builder resolverRuleIds(String... resolverRuleIds) {
return resolverRuleIds(List.of(resolverRuleIds));
}
@CustomType.Setter
public Builder ruleType(@Nullable String ruleType) {
this.ruleType = ruleType;
return this;
}
@CustomType.Setter
public Builder shareStatus(@Nullable String shareStatus) {
this.shareStatus = shareStatus;
return this;
}
public GetResolverRulesResult build() {
final var _resultValue = new GetResolverRulesResult();
_resultValue.id = id;
_resultValue.nameRegex = nameRegex;
_resultValue.ownerId = ownerId;
_resultValue.resolverEndpointId = resolverEndpointId;
_resultValue.resolverRuleIds = resolverRuleIds;
_resultValue.ruleType = ruleType;
_resultValue.shareStatus = shareStatus;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy