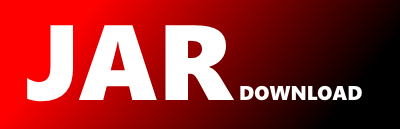
com.pulumi.aws.servicequotas.inputs.ServiceQuotaState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.servicequotas.inputs;
import com.pulumi.aws.servicequotas.inputs.ServiceQuotaUsageMetricArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ServiceQuotaState extends com.pulumi.resources.ResourceArgs {
public static final ServiceQuotaState Empty = new ServiceQuotaState();
/**
* Whether the service quota can be increased.
*
*/
@Import(name="adjustable")
private @Nullable Output adjustable;
/**
* @return Whether the service quota can be increased.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy