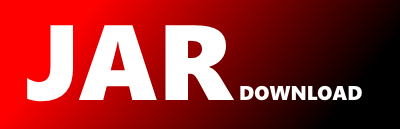
com.pulumi.aws.wafv2.inputs.WebAclLoggingConfigurationRedactedFieldArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.wafv2.inputs;
import com.pulumi.aws.wafv2.inputs.WebAclLoggingConfigurationRedactedFieldMethodArgs;
import com.pulumi.aws.wafv2.inputs.WebAclLoggingConfigurationRedactedFieldQueryStringArgs;
import com.pulumi.aws.wafv2.inputs.WebAclLoggingConfigurationRedactedFieldSingleHeaderArgs;
import com.pulumi.aws.wafv2.inputs.WebAclLoggingConfigurationRedactedFieldUriPathArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class WebAclLoggingConfigurationRedactedFieldArgs extends com.pulumi.resources.ResourceArgs {
public static final WebAclLoggingConfigurationRedactedFieldArgs Empty = new WebAclLoggingConfigurationRedactedFieldArgs();
/**
* HTTP method to be redacted. It must be specified as an empty configuration block `{}`. The method indicates the type of operation that the request is asking the origin to perform.
*
*/
@Import(name="method")
private @Nullable Output method;
/**
* @return HTTP method to be redacted. It must be specified as an empty configuration block `{}`. The method indicates the type of operation that the request is asking the origin to perform.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy