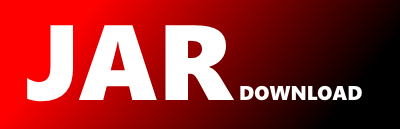
com.pulumi.aws.wafv2.inputs.WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.wafv2.inputs;
import com.pulumi.aws.wafv2.inputs.WebAclRuleStatementXssMatchStatementFieldToMatchCookiesMatchPatternArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
public final class WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs extends com.pulumi.resources.ResourceArgs {
public static final WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs Empty = new WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs();
/**
* The filter to use to identify the subset of cookies to inspect in a web request. You must specify exactly one setting: either `all`, `included_cookies` or `excluded_cookies`. More details: [CookieMatchPattern](https://docs.aws.amazon.com/waf/latest/APIReference/API_CookieMatchPattern.html)
*
*/
@Import(name="matchPatterns", required=true)
private Output> matchPatterns;
/**
* @return The filter to use to identify the subset of cookies to inspect in a web request. You must specify exactly one setting: either `all`, `included_cookies` or `excluded_cookies`. More details: [CookieMatchPattern](https://docs.aws.amazon.com/waf/latest/APIReference/API_CookieMatchPattern.html)
*
*/
public Output> matchPatterns() {
return this.matchPatterns;
}
/**
* The parts of the cookies to inspect with the rule inspection criteria. If you specify All, AWS WAF inspects both keys and values. Valid values: `ALL`, `KEY`, `VALUE`
*
*/
@Import(name="matchScope", required=true)
private Output matchScope;
/**
* @return The parts of the cookies to inspect with the rule inspection criteria. If you specify All, AWS WAF inspects both keys and values. Valid values: `ALL`, `KEY`, `VALUE`
*
*/
public Output matchScope() {
return this.matchScope;
}
/**
* What AWS WAF should do if the cookies of the request are larger than AWS WAF can inspect. AWS WAF does not support inspecting the entire contents of request cookies when they exceed 8 KB (8192 bytes) or 200 total cookies. The underlying host service forwards a maximum of 200 cookies and at most 8 KB of cookie contents to AWS WAF. Valid values: `CONTINUE`, `MATCH`, `NO_MATCH`.
*
*/
@Import(name="oversizeHandling", required=true)
private Output oversizeHandling;
/**
* @return What AWS WAF should do if the cookies of the request are larger than AWS WAF can inspect. AWS WAF does not support inspecting the entire contents of request cookies when they exceed 8 KB (8192 bytes) or 200 total cookies. The underlying host service forwards a maximum of 200 cookies and at most 8 KB of cookie contents to AWS WAF. Valid values: `CONTINUE`, `MATCH`, `NO_MATCH`.
*
*/
public Output oversizeHandling() {
return this.oversizeHandling;
}
private WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs() {}
private WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs(WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs $) {
this.matchPatterns = $.matchPatterns;
this.matchScope = $.matchScope;
this.oversizeHandling = $.oversizeHandling;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs $;
public Builder() {
$ = new WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs();
}
public Builder(WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs defaults) {
$ = new WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs(Objects.requireNonNull(defaults));
}
/**
* @param matchPatterns The filter to use to identify the subset of cookies to inspect in a web request. You must specify exactly one setting: either `all`, `included_cookies` or `excluded_cookies`. More details: [CookieMatchPattern](https://docs.aws.amazon.com/waf/latest/APIReference/API_CookieMatchPattern.html)
*
* @return builder
*
*/
public Builder matchPatterns(Output> matchPatterns) {
$.matchPatterns = matchPatterns;
return this;
}
/**
* @param matchPatterns The filter to use to identify the subset of cookies to inspect in a web request. You must specify exactly one setting: either `all`, `included_cookies` or `excluded_cookies`. More details: [CookieMatchPattern](https://docs.aws.amazon.com/waf/latest/APIReference/API_CookieMatchPattern.html)
*
* @return builder
*
*/
public Builder matchPatterns(List matchPatterns) {
return matchPatterns(Output.of(matchPatterns));
}
/**
* @param matchPatterns The filter to use to identify the subset of cookies to inspect in a web request. You must specify exactly one setting: either `all`, `included_cookies` or `excluded_cookies`. More details: [CookieMatchPattern](https://docs.aws.amazon.com/waf/latest/APIReference/API_CookieMatchPattern.html)
*
* @return builder
*
*/
public Builder matchPatterns(WebAclRuleStatementXssMatchStatementFieldToMatchCookiesMatchPatternArgs... matchPatterns) {
return matchPatterns(List.of(matchPatterns));
}
/**
* @param matchScope The parts of the cookies to inspect with the rule inspection criteria. If you specify All, AWS WAF inspects both keys and values. Valid values: `ALL`, `KEY`, `VALUE`
*
* @return builder
*
*/
public Builder matchScope(Output matchScope) {
$.matchScope = matchScope;
return this;
}
/**
* @param matchScope The parts of the cookies to inspect with the rule inspection criteria. If you specify All, AWS WAF inspects both keys and values. Valid values: `ALL`, `KEY`, `VALUE`
*
* @return builder
*
*/
public Builder matchScope(String matchScope) {
return matchScope(Output.of(matchScope));
}
/**
* @param oversizeHandling What AWS WAF should do if the cookies of the request are larger than AWS WAF can inspect. AWS WAF does not support inspecting the entire contents of request cookies when they exceed 8 KB (8192 bytes) or 200 total cookies. The underlying host service forwards a maximum of 200 cookies and at most 8 KB of cookie contents to AWS WAF. Valid values: `CONTINUE`, `MATCH`, `NO_MATCH`.
*
* @return builder
*
*/
public Builder oversizeHandling(Output oversizeHandling) {
$.oversizeHandling = oversizeHandling;
return this;
}
/**
* @param oversizeHandling What AWS WAF should do if the cookies of the request are larger than AWS WAF can inspect. AWS WAF does not support inspecting the entire contents of request cookies when they exceed 8 KB (8192 bytes) or 200 total cookies. The underlying host service forwards a maximum of 200 cookies and at most 8 KB of cookie contents to AWS WAF. Valid values: `CONTINUE`, `MATCH`, `NO_MATCH`.
*
* @return builder
*
*/
public Builder oversizeHandling(String oversizeHandling) {
return oversizeHandling(Output.of(oversizeHandling));
}
public WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs build() {
if ($.matchPatterns == null) {
throw new MissingRequiredPropertyException("WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs", "matchPatterns");
}
if ($.matchScope == null) {
throw new MissingRequiredPropertyException("WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs", "matchScope");
}
if ($.oversizeHandling == null) {
throw new MissingRequiredPropertyException("WebAclRuleStatementXssMatchStatementFieldToMatchCookiesArgs", "oversizeHandling");
}
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy