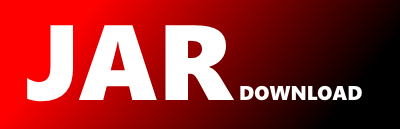
com.pulumi.aws.budgets.outputs.GetBudgetNotification Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.budgets.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetBudgetNotification {
/**
* @return (Required) Comparison operator to use to evaluate the condition. Can be `LESS_THAN`, `EQUAL_TO` or `GREATER_THAN`.
*
*/
private String comparisonOperator;
/**
* @return (Required) What kind of budget value to notify on. Can be `ACTUAL` or `FORECASTED`.
*
*/
private String notificationType;
/**
* @return (Optional) E-Mail addresses to notify. Either this or `subscriber_sns_topic_arns` is required.
*
*/
private List subscriberEmailAddresses;
/**
* @return (Optional) SNS topics to notify. Either this or `subscriber_email_addresses` is required.
*
*/
private List subscriberSnsTopicArns;
/**
* @return (Required) Threshold when the notification should be sent.
*
*/
private Double threshold;
/**
* @return (Required) What kind of threshold is defined. Can be `PERCENTAGE` OR `ABSOLUTE_VALUE`.
*
*/
private String thresholdType;
private GetBudgetNotification() {}
/**
* @return (Required) Comparison operator to use to evaluate the condition. Can be `LESS_THAN`, `EQUAL_TO` or `GREATER_THAN`.
*
*/
public String comparisonOperator() {
return this.comparisonOperator;
}
/**
* @return (Required) What kind of budget value to notify on. Can be `ACTUAL` or `FORECASTED`.
*
*/
public String notificationType() {
return this.notificationType;
}
/**
* @return (Optional) E-Mail addresses to notify. Either this or `subscriber_sns_topic_arns` is required.
*
*/
public List subscriberEmailAddresses() {
return this.subscriberEmailAddresses;
}
/**
* @return (Optional) SNS topics to notify. Either this or `subscriber_email_addresses` is required.
*
*/
public List subscriberSnsTopicArns() {
return this.subscriberSnsTopicArns;
}
/**
* @return (Required) Threshold when the notification should be sent.
*
*/
public Double threshold() {
return this.threshold;
}
/**
* @return (Required) What kind of threshold is defined. Can be `PERCENTAGE` OR `ABSOLUTE_VALUE`.
*
*/
public String thresholdType() {
return this.thresholdType;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBudgetNotification defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String comparisonOperator;
private String notificationType;
private List subscriberEmailAddresses;
private List subscriberSnsTopicArns;
private Double threshold;
private String thresholdType;
public Builder() {}
public Builder(GetBudgetNotification defaults) {
Objects.requireNonNull(defaults);
this.comparisonOperator = defaults.comparisonOperator;
this.notificationType = defaults.notificationType;
this.subscriberEmailAddresses = defaults.subscriberEmailAddresses;
this.subscriberSnsTopicArns = defaults.subscriberSnsTopicArns;
this.threshold = defaults.threshold;
this.thresholdType = defaults.thresholdType;
}
@CustomType.Setter
public Builder comparisonOperator(String comparisonOperator) {
if (comparisonOperator == null) {
throw new MissingRequiredPropertyException("GetBudgetNotification", "comparisonOperator");
}
this.comparisonOperator = comparisonOperator;
return this;
}
@CustomType.Setter
public Builder notificationType(String notificationType) {
if (notificationType == null) {
throw new MissingRequiredPropertyException("GetBudgetNotification", "notificationType");
}
this.notificationType = notificationType;
return this;
}
@CustomType.Setter
public Builder subscriberEmailAddresses(List subscriberEmailAddresses) {
if (subscriberEmailAddresses == null) {
throw new MissingRequiredPropertyException("GetBudgetNotification", "subscriberEmailAddresses");
}
this.subscriberEmailAddresses = subscriberEmailAddresses;
return this;
}
public Builder subscriberEmailAddresses(String... subscriberEmailAddresses) {
return subscriberEmailAddresses(List.of(subscriberEmailAddresses));
}
@CustomType.Setter
public Builder subscriberSnsTopicArns(List subscriberSnsTopicArns) {
if (subscriberSnsTopicArns == null) {
throw new MissingRequiredPropertyException("GetBudgetNotification", "subscriberSnsTopicArns");
}
this.subscriberSnsTopicArns = subscriberSnsTopicArns;
return this;
}
public Builder subscriberSnsTopicArns(String... subscriberSnsTopicArns) {
return subscriberSnsTopicArns(List.of(subscriberSnsTopicArns));
}
@CustomType.Setter
public Builder threshold(Double threshold) {
if (threshold == null) {
throw new MissingRequiredPropertyException("GetBudgetNotification", "threshold");
}
this.threshold = threshold;
return this;
}
@CustomType.Setter
public Builder thresholdType(String thresholdType) {
if (thresholdType == null) {
throw new MissingRequiredPropertyException("GetBudgetNotification", "thresholdType");
}
this.thresholdType = thresholdType;
return this;
}
public GetBudgetNotification build() {
final var _resultValue = new GetBudgetNotification();
_resultValue.comparisonOperator = comparisonOperator;
_resultValue.notificationType = notificationType;
_resultValue.subscriberEmailAddresses = subscriberEmailAddresses;
_resultValue.subscriberSnsTopicArns = subscriberSnsTopicArns;
_resultValue.threshold = threshold;
_resultValue.thresholdType = thresholdType;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy