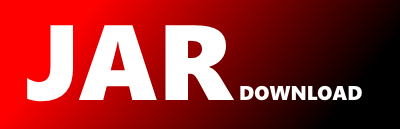
com.pulumi.aws.datasync.inputs.EfsLocationState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.datasync.inputs;
import com.pulumi.aws.datasync.inputs.EfsLocationEc2ConfigArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EfsLocationState extends com.pulumi.resources.ResourceArgs {
public static final EfsLocationState Empty = new EfsLocationState();
/**
* Specifies the Amazon Resource Name (ARN) of the access point that DataSync uses to access the Amazon EFS file system.
*
*/
@Import(name="accessPointArn")
private @Nullable Output accessPointArn;
/**
* @return Specifies the Amazon Resource Name (ARN) of the access point that DataSync uses to access the Amazon EFS file system.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy