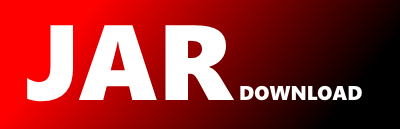
com.pulumi.aws.ec2.inputs.DefaultSecurityGroupState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.inputs;
import com.pulumi.aws.ec2.inputs.DefaultSecurityGroupEgressArgs;
import com.pulumi.aws.ec2.inputs.DefaultSecurityGroupIngressArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DefaultSecurityGroupState extends com.pulumi.resources.ResourceArgs {
public static final DefaultSecurityGroupState Empty = new DefaultSecurityGroupState();
/**
* ARN of the security group.
*
*/
@Import(name="arn")
private @Nullable Output arn;
/**
* @return ARN of the security group.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy