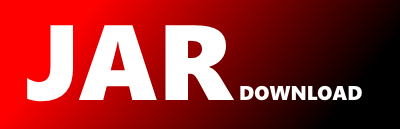
com.pulumi.aws.ecs.outputs.GetTaskExecutionResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ecs.outputs;
import com.pulumi.aws.ecs.outputs.GetTaskExecutionCapacityProviderStrategy;
import com.pulumi.aws.ecs.outputs.GetTaskExecutionNetworkConfiguration;
import com.pulumi.aws.ecs.outputs.GetTaskExecutionOverrides;
import com.pulumi.aws.ecs.outputs.GetTaskExecutionPlacementConstraint;
import com.pulumi.aws.ecs.outputs.GetTaskExecutionPlacementStrategy;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetTaskExecutionResult {
private @Nullable List capacityProviderStrategies;
private @Nullable String clientToken;
private String cluster;
private @Nullable Integer desiredCount;
private @Nullable Boolean enableEcsManagedTags;
private @Nullable Boolean enableExecuteCommand;
private @Nullable String group;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private @Nullable String launchType;
private @Nullable GetTaskExecutionNetworkConfiguration networkConfiguration;
private @Nullable GetTaskExecutionOverrides overrides;
private @Nullable List placementConstraints;
private @Nullable List placementStrategies;
private @Nullable String platformVersion;
private @Nullable String propagateTags;
private @Nullable String referenceId;
private @Nullable String startedBy;
private @Nullable Map tags;
/**
* @return A list of the provisioned task ARNs.
*
*/
private List taskArns;
private String taskDefinition;
private GetTaskExecutionResult() {}
public List capacityProviderStrategies() {
return this.capacityProviderStrategies == null ? List.of() : this.capacityProviderStrategies;
}
public Optional clientToken() {
return Optional.ofNullable(this.clientToken);
}
public String cluster() {
return this.cluster;
}
public Optional desiredCount() {
return Optional.ofNullable(this.desiredCount);
}
public Optional enableEcsManagedTags() {
return Optional.ofNullable(this.enableEcsManagedTags);
}
public Optional enableExecuteCommand() {
return Optional.ofNullable(this.enableExecuteCommand);
}
public Optional group() {
return Optional.ofNullable(this.group);
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public Optional launchType() {
return Optional.ofNullable(this.launchType);
}
public Optional networkConfiguration() {
return Optional.ofNullable(this.networkConfiguration);
}
public Optional overrides() {
return Optional.ofNullable(this.overrides);
}
public List placementConstraints() {
return this.placementConstraints == null ? List.of() : this.placementConstraints;
}
public List placementStrategies() {
return this.placementStrategies == null ? List.of() : this.placementStrategies;
}
public Optional platformVersion() {
return Optional.ofNullable(this.platformVersion);
}
public Optional propagateTags() {
return Optional.ofNullable(this.propagateTags);
}
public Optional referenceId() {
return Optional.ofNullable(this.referenceId);
}
public Optional startedBy() {
return Optional.ofNullable(this.startedBy);
}
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return A list of the provisioned task ARNs.
*
*/
public List taskArns() {
return this.taskArns;
}
public String taskDefinition() {
return this.taskDefinition;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetTaskExecutionResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List capacityProviderStrategies;
private @Nullable String clientToken;
private String cluster;
private @Nullable Integer desiredCount;
private @Nullable Boolean enableEcsManagedTags;
private @Nullable Boolean enableExecuteCommand;
private @Nullable String group;
private String id;
private @Nullable String launchType;
private @Nullable GetTaskExecutionNetworkConfiguration networkConfiguration;
private @Nullable GetTaskExecutionOverrides overrides;
private @Nullable List placementConstraints;
private @Nullable List placementStrategies;
private @Nullable String platformVersion;
private @Nullable String propagateTags;
private @Nullable String referenceId;
private @Nullable String startedBy;
private @Nullable Map tags;
private List taskArns;
private String taskDefinition;
public Builder() {}
public Builder(GetTaskExecutionResult defaults) {
Objects.requireNonNull(defaults);
this.capacityProviderStrategies = defaults.capacityProviderStrategies;
this.clientToken = defaults.clientToken;
this.cluster = defaults.cluster;
this.desiredCount = defaults.desiredCount;
this.enableEcsManagedTags = defaults.enableEcsManagedTags;
this.enableExecuteCommand = defaults.enableExecuteCommand;
this.group = defaults.group;
this.id = defaults.id;
this.launchType = defaults.launchType;
this.networkConfiguration = defaults.networkConfiguration;
this.overrides = defaults.overrides;
this.placementConstraints = defaults.placementConstraints;
this.placementStrategies = defaults.placementStrategies;
this.platformVersion = defaults.platformVersion;
this.propagateTags = defaults.propagateTags;
this.referenceId = defaults.referenceId;
this.startedBy = defaults.startedBy;
this.tags = defaults.tags;
this.taskArns = defaults.taskArns;
this.taskDefinition = defaults.taskDefinition;
}
@CustomType.Setter
public Builder capacityProviderStrategies(@Nullable List capacityProviderStrategies) {
this.capacityProviderStrategies = capacityProviderStrategies;
return this;
}
public Builder capacityProviderStrategies(GetTaskExecutionCapacityProviderStrategy... capacityProviderStrategies) {
return capacityProviderStrategies(List.of(capacityProviderStrategies));
}
@CustomType.Setter
public Builder clientToken(@Nullable String clientToken) {
this.clientToken = clientToken;
return this;
}
@CustomType.Setter
public Builder cluster(String cluster) {
if (cluster == null) {
throw new MissingRequiredPropertyException("GetTaskExecutionResult", "cluster");
}
this.cluster = cluster;
return this;
}
@CustomType.Setter
public Builder desiredCount(@Nullable Integer desiredCount) {
this.desiredCount = desiredCount;
return this;
}
@CustomType.Setter
public Builder enableEcsManagedTags(@Nullable Boolean enableEcsManagedTags) {
this.enableEcsManagedTags = enableEcsManagedTags;
return this;
}
@CustomType.Setter
public Builder enableExecuteCommand(@Nullable Boolean enableExecuteCommand) {
this.enableExecuteCommand = enableExecuteCommand;
return this;
}
@CustomType.Setter
public Builder group(@Nullable String group) {
this.group = group;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetTaskExecutionResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder launchType(@Nullable String launchType) {
this.launchType = launchType;
return this;
}
@CustomType.Setter
public Builder networkConfiguration(@Nullable GetTaskExecutionNetworkConfiguration networkConfiguration) {
this.networkConfiguration = networkConfiguration;
return this;
}
@CustomType.Setter
public Builder overrides(@Nullable GetTaskExecutionOverrides overrides) {
this.overrides = overrides;
return this;
}
@CustomType.Setter
public Builder placementConstraints(@Nullable List placementConstraints) {
this.placementConstraints = placementConstraints;
return this;
}
public Builder placementConstraints(GetTaskExecutionPlacementConstraint... placementConstraints) {
return placementConstraints(List.of(placementConstraints));
}
@CustomType.Setter
public Builder placementStrategies(@Nullable List placementStrategies) {
this.placementStrategies = placementStrategies;
return this;
}
public Builder placementStrategies(GetTaskExecutionPlacementStrategy... placementStrategies) {
return placementStrategies(List.of(placementStrategies));
}
@CustomType.Setter
public Builder platformVersion(@Nullable String platformVersion) {
this.platformVersion = platformVersion;
return this;
}
@CustomType.Setter
public Builder propagateTags(@Nullable String propagateTags) {
this.propagateTags = propagateTags;
return this;
}
@CustomType.Setter
public Builder referenceId(@Nullable String referenceId) {
this.referenceId = referenceId;
return this;
}
@CustomType.Setter
public Builder startedBy(@Nullable String startedBy) {
this.startedBy = startedBy;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder taskArns(List taskArns) {
if (taskArns == null) {
throw new MissingRequiredPropertyException("GetTaskExecutionResult", "taskArns");
}
this.taskArns = taskArns;
return this;
}
public Builder taskArns(String... taskArns) {
return taskArns(List.of(taskArns));
}
@CustomType.Setter
public Builder taskDefinition(String taskDefinition) {
if (taskDefinition == null) {
throw new MissingRequiredPropertyException("GetTaskExecutionResult", "taskDefinition");
}
this.taskDefinition = taskDefinition;
return this;
}
public GetTaskExecutionResult build() {
final var _resultValue = new GetTaskExecutionResult();
_resultValue.capacityProviderStrategies = capacityProviderStrategies;
_resultValue.clientToken = clientToken;
_resultValue.cluster = cluster;
_resultValue.desiredCount = desiredCount;
_resultValue.enableEcsManagedTags = enableEcsManagedTags;
_resultValue.enableExecuteCommand = enableExecuteCommand;
_resultValue.group = group;
_resultValue.id = id;
_resultValue.launchType = launchType;
_resultValue.networkConfiguration = networkConfiguration;
_resultValue.overrides = overrides;
_resultValue.placementConstraints = placementConstraints;
_resultValue.placementStrategies = placementStrategies;
_resultValue.platformVersion = platformVersion;
_resultValue.propagateTags = propagateTags;
_resultValue.referenceId = referenceId;
_resultValue.startedBy = startedBy;
_resultValue.tags = tags;
_resultValue.taskArns = taskArns;
_resultValue.taskDefinition = taskDefinition;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy