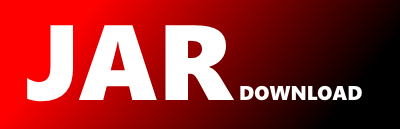
com.pulumi.aws.kms.ExternalKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.kms;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.kms.ExternalKeyArgs;
import com.pulumi.aws.kms.inputs.ExternalKeyState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages a single-Region or multi-Region primary KMS key that uses external key material.
* To instead manage a single-Region or multi-Region primary KMS key where AWS automatically generates and potentially rotates key material, see the `aws.kms.Key` resource.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.ExternalKey;
* import com.pulumi.aws.kms.ExternalKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new ExternalKey("example", ExternalKeyArgs.builder()
* .description("KMS EXTERNAL for AMI encryption")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import KMS External Keys using the `id`. For example:
*
* ```sh
* $ pulumi import aws:kms/externalKey:ExternalKey a arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
* ```
*
*/
@ResourceType(type="aws:kms/externalKey:ExternalKey")
public class ExternalKey extends com.pulumi.resources.CustomResource {
/**
* The Amazon Resource Name (ARN) of the key.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The Amazon Resource Name (ARN) of the key.
*
*/
public Output arn() {
return this.arn;
}
/**
* Specifies whether to disable the policy lockout check performed when creating or updating the key's policy. Setting this value to `true` increases the risk that the key becomes unmanageable. For more information, refer to the scenario in the [Default Key Policy](https://docs.aws.amazon.com/kms/latest/developerguide/key-policies.html#key-policy-default-allow-root-enable-iam) section in the AWS Key Management Service Developer Guide. Defaults to `false`.
*
*/
@Export(name="bypassPolicyLockoutSafetyCheck", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> bypassPolicyLockoutSafetyCheck;
/**
* @return Specifies whether to disable the policy lockout check performed when creating or updating the key's policy. Setting this value to `true` increases the risk that the key becomes unmanageable. For more information, refer to the scenario in the [Default Key Policy](https://docs.aws.amazon.com/kms/latest/developerguide/key-policies.html#key-policy-default-allow-root-enable-iam) section in the AWS Key Management Service Developer Guide. Defaults to `false`.
*
*/
public Output> bypassPolicyLockoutSafetyCheck() {
return Codegen.optional(this.bypassPolicyLockoutSafetyCheck);
}
/**
* Duration in days after which the key is deleted after destruction of the resource. Must be between `7` and `30` days. Defaults to `30`.
*
*/
@Export(name="deletionWindowInDays", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> deletionWindowInDays;
/**
* @return Duration in days after which the key is deleted after destruction of the resource. Must be between `7` and `30` days. Defaults to `30`.
*
*/
public Output> deletionWindowInDays() {
return Codegen.optional(this.deletionWindowInDays);
}
/**
* Description of the key.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description of the key.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* Specifies whether the key is enabled. Keys pending import can only be `false`. Imported keys default to `true` unless expired.
*
*/
@Export(name="enabled", refs={Boolean.class}, tree="[0]")
private Output enabled;
/**
* @return Specifies whether the key is enabled. Keys pending import can only be `false`. Imported keys default to `true` unless expired.
*
*/
public Output enabled() {
return this.enabled;
}
/**
* Whether the key material expires. Empty when pending key material import, otherwise `KEY_MATERIAL_EXPIRES` or `KEY_MATERIAL_DOES_NOT_EXPIRE`.
*
*/
@Export(name="expirationModel", refs={String.class}, tree="[0]")
private Output expirationModel;
/**
* @return Whether the key material expires. Empty when pending key material import, otherwise `KEY_MATERIAL_EXPIRES` or `KEY_MATERIAL_DOES_NOT_EXPIRE`.
*
*/
public Output expirationModel() {
return this.expirationModel;
}
/**
* Base64 encoded 256-bit symmetric encryption key material to import. The CMK is permanently associated with this key material. The same key material can be reimported, but you cannot import different key material.
*
*/
@Export(name="keyMaterialBase64", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> keyMaterialBase64;
/**
* @return Base64 encoded 256-bit symmetric encryption key material to import. The CMK is permanently associated with this key material. The same key material can be reimported, but you cannot import different key material.
*
*/
public Output> keyMaterialBase64() {
return Codegen.optional(this.keyMaterialBase64);
}
/**
* The state of the CMK.
*
*/
@Export(name="keyState", refs={String.class}, tree="[0]")
private Output keyState;
/**
* @return The state of the CMK.
*
*/
public Output keyState() {
return this.keyState;
}
/**
* The cryptographic operations for which you can use the CMK.
*
*/
@Export(name="keyUsage", refs={String.class}, tree="[0]")
private Output keyUsage;
/**
* @return The cryptographic operations for which you can use the CMK.
*
*/
public Output keyUsage() {
return this.keyUsage;
}
/**
* Indicates whether the KMS key is a multi-Region (`true`) or regional (`false`) key. Defaults to `false`.
*
*/
@Export(name="multiRegion", refs={Boolean.class}, tree="[0]")
private Output multiRegion;
/**
* @return Indicates whether the KMS key is a multi-Region (`true`) or regional (`false`) key. Defaults to `false`.
*
*/
public Output multiRegion() {
return this.multiRegion;
}
/**
* A key policy JSON document. If you do not provide a key policy, AWS KMS attaches a default key policy to the CMK.
*
*/
@Export(name="policy", refs={String.class}, tree="[0]")
private Output policy;
/**
* @return A key policy JSON document. If you do not provide a key policy, AWS KMS attaches a default key policy to the CMK.
*
*/
public Output policy() {
return this.policy;
}
/**
* A key-value map of tags to assign to the key. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A key-value map of tags to assign to the key. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy