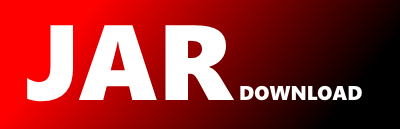
com.pulumi.aws.medialive.outputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettings Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.medialive.outputs;
import com.pulumi.aws.medialive.outputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsCaptionLanguageMapping;
import com.pulumi.aws.medialive.outputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsDestination;
import com.pulumi.aws.medialive.outputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsHlsCdnSetting;
import com.pulumi.aws.medialive.outputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsKeyProviderSettings;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettings {
/**
* @return The ad marker type for this output group.
*
*/
private @Nullable List adMarkers;
private @Nullable String baseUrlContent;
private @Nullable String baseUrlContent1;
private @Nullable String baseUrlManifest;
private @Nullable String baseUrlManifest1;
private @Nullable List captionLanguageMappings;
private @Nullable String captionLanguageSetting;
private @Nullable String clientCache;
private @Nullable String codecSpecification;
private @Nullable String constantIv;
private ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsDestination destination;
private @Nullable String directoryStructure;
private @Nullable String discontinuityTags;
private @Nullable String encryptionType;
private @Nullable List hlsCdnSettings;
private @Nullable String hlsId3SegmentTagging;
private @Nullable String iframeOnlyPlaylists;
private @Nullable String incompleteSegmentBehavior;
private @Nullable Integer indexNSegments;
private @Nullable String inputLossAction;
private @Nullable String ivInManifest;
private @Nullable String ivSource;
private @Nullable Integer keepSegments;
private @Nullable String keyFormat;
private @Nullable String keyFormatVersions;
private @Nullable ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsKeyProviderSettings keyProviderSettings;
private @Nullable String manifestCompression;
private @Nullable String manifestDurationFormat;
private @Nullable Integer minSegmentLength;
private @Nullable String mode;
private @Nullable String outputSelection;
private @Nullable String programDateTime;
private @Nullable String programDateTimeClock;
private @Nullable Integer programDateTimePeriod;
private @Nullable String redundantManifest;
private @Nullable Integer segmentLength;
private @Nullable Integer segmentsPerSubdirectory;
private @Nullable String streamInfResolution;
/**
* @return Indicates ID3 frame that has the timecode.
*
*/
private @Nullable String timedMetadataId3Frame;
private @Nullable Integer timedMetadataId3Period;
private @Nullable Integer timestampDeltaMilliseconds;
private @Nullable String tsFileMode;
private ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettings() {}
/**
* @return The ad marker type for this output group.
*
*/
public List adMarkers() {
return this.adMarkers == null ? List.of() : this.adMarkers;
}
public Optional baseUrlContent() {
return Optional.ofNullable(this.baseUrlContent);
}
public Optional baseUrlContent1() {
return Optional.ofNullable(this.baseUrlContent1);
}
public Optional baseUrlManifest() {
return Optional.ofNullable(this.baseUrlManifest);
}
public Optional baseUrlManifest1() {
return Optional.ofNullable(this.baseUrlManifest1);
}
public List captionLanguageMappings() {
return this.captionLanguageMappings == null ? List.of() : this.captionLanguageMappings;
}
public Optional captionLanguageSetting() {
return Optional.ofNullable(this.captionLanguageSetting);
}
public Optional clientCache() {
return Optional.ofNullable(this.clientCache);
}
public Optional codecSpecification() {
return Optional.ofNullable(this.codecSpecification);
}
public Optional constantIv() {
return Optional.ofNullable(this.constantIv);
}
public ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsDestination destination() {
return this.destination;
}
public Optional directoryStructure() {
return Optional.ofNullable(this.directoryStructure);
}
public Optional discontinuityTags() {
return Optional.ofNullable(this.discontinuityTags);
}
public Optional encryptionType() {
return Optional.ofNullable(this.encryptionType);
}
public List hlsCdnSettings() {
return this.hlsCdnSettings == null ? List.of() : this.hlsCdnSettings;
}
public Optional hlsId3SegmentTagging() {
return Optional.ofNullable(this.hlsId3SegmentTagging);
}
public Optional iframeOnlyPlaylists() {
return Optional.ofNullable(this.iframeOnlyPlaylists);
}
public Optional incompleteSegmentBehavior() {
return Optional.ofNullable(this.incompleteSegmentBehavior);
}
public Optional indexNSegments() {
return Optional.ofNullable(this.indexNSegments);
}
public Optional inputLossAction() {
return Optional.ofNullable(this.inputLossAction);
}
public Optional ivInManifest() {
return Optional.ofNullable(this.ivInManifest);
}
public Optional ivSource() {
return Optional.ofNullable(this.ivSource);
}
public Optional keepSegments() {
return Optional.ofNullable(this.keepSegments);
}
public Optional keyFormat() {
return Optional.ofNullable(this.keyFormat);
}
public Optional keyFormatVersions() {
return Optional.ofNullable(this.keyFormatVersions);
}
public Optional keyProviderSettings() {
return Optional.ofNullable(this.keyProviderSettings);
}
public Optional manifestCompression() {
return Optional.ofNullable(this.manifestCompression);
}
public Optional manifestDurationFormat() {
return Optional.ofNullable(this.manifestDurationFormat);
}
public Optional minSegmentLength() {
return Optional.ofNullable(this.minSegmentLength);
}
public Optional mode() {
return Optional.ofNullable(this.mode);
}
public Optional outputSelection() {
return Optional.ofNullable(this.outputSelection);
}
public Optional programDateTime() {
return Optional.ofNullable(this.programDateTime);
}
public Optional programDateTimeClock() {
return Optional.ofNullable(this.programDateTimeClock);
}
public Optional programDateTimePeriod() {
return Optional.ofNullable(this.programDateTimePeriod);
}
public Optional redundantManifest() {
return Optional.ofNullable(this.redundantManifest);
}
public Optional segmentLength() {
return Optional.ofNullable(this.segmentLength);
}
public Optional segmentsPerSubdirectory() {
return Optional.ofNullable(this.segmentsPerSubdirectory);
}
public Optional streamInfResolution() {
return Optional.ofNullable(this.streamInfResolution);
}
/**
* @return Indicates ID3 frame that has the timecode.
*
*/
public Optional timedMetadataId3Frame() {
return Optional.ofNullable(this.timedMetadataId3Frame);
}
public Optional timedMetadataId3Period() {
return Optional.ofNullable(this.timedMetadataId3Period);
}
public Optional timestampDeltaMilliseconds() {
return Optional.ofNullable(this.timestampDeltaMilliseconds);
}
public Optional tsFileMode() {
return Optional.ofNullable(this.tsFileMode);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettings defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List adMarkers;
private @Nullable String baseUrlContent;
private @Nullable String baseUrlContent1;
private @Nullable String baseUrlManifest;
private @Nullable String baseUrlManifest1;
private @Nullable List captionLanguageMappings;
private @Nullable String captionLanguageSetting;
private @Nullable String clientCache;
private @Nullable String codecSpecification;
private @Nullable String constantIv;
private ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsDestination destination;
private @Nullable String directoryStructure;
private @Nullable String discontinuityTags;
private @Nullable String encryptionType;
private @Nullable List hlsCdnSettings;
private @Nullable String hlsId3SegmentTagging;
private @Nullable String iframeOnlyPlaylists;
private @Nullable String incompleteSegmentBehavior;
private @Nullable Integer indexNSegments;
private @Nullable String inputLossAction;
private @Nullable String ivInManifest;
private @Nullable String ivSource;
private @Nullable Integer keepSegments;
private @Nullable String keyFormat;
private @Nullable String keyFormatVersions;
private @Nullable ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsKeyProviderSettings keyProviderSettings;
private @Nullable String manifestCompression;
private @Nullable String manifestDurationFormat;
private @Nullable Integer minSegmentLength;
private @Nullable String mode;
private @Nullable String outputSelection;
private @Nullable String programDateTime;
private @Nullable String programDateTimeClock;
private @Nullable Integer programDateTimePeriod;
private @Nullable String redundantManifest;
private @Nullable Integer segmentLength;
private @Nullable Integer segmentsPerSubdirectory;
private @Nullable String streamInfResolution;
private @Nullable String timedMetadataId3Frame;
private @Nullable Integer timedMetadataId3Period;
private @Nullable Integer timestampDeltaMilliseconds;
private @Nullable String tsFileMode;
public Builder() {}
public Builder(ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettings defaults) {
Objects.requireNonNull(defaults);
this.adMarkers = defaults.adMarkers;
this.baseUrlContent = defaults.baseUrlContent;
this.baseUrlContent1 = defaults.baseUrlContent1;
this.baseUrlManifest = defaults.baseUrlManifest;
this.baseUrlManifest1 = defaults.baseUrlManifest1;
this.captionLanguageMappings = defaults.captionLanguageMappings;
this.captionLanguageSetting = defaults.captionLanguageSetting;
this.clientCache = defaults.clientCache;
this.codecSpecification = defaults.codecSpecification;
this.constantIv = defaults.constantIv;
this.destination = defaults.destination;
this.directoryStructure = defaults.directoryStructure;
this.discontinuityTags = defaults.discontinuityTags;
this.encryptionType = defaults.encryptionType;
this.hlsCdnSettings = defaults.hlsCdnSettings;
this.hlsId3SegmentTagging = defaults.hlsId3SegmentTagging;
this.iframeOnlyPlaylists = defaults.iframeOnlyPlaylists;
this.incompleteSegmentBehavior = defaults.incompleteSegmentBehavior;
this.indexNSegments = defaults.indexNSegments;
this.inputLossAction = defaults.inputLossAction;
this.ivInManifest = defaults.ivInManifest;
this.ivSource = defaults.ivSource;
this.keepSegments = defaults.keepSegments;
this.keyFormat = defaults.keyFormat;
this.keyFormatVersions = defaults.keyFormatVersions;
this.keyProviderSettings = defaults.keyProviderSettings;
this.manifestCompression = defaults.manifestCompression;
this.manifestDurationFormat = defaults.manifestDurationFormat;
this.minSegmentLength = defaults.minSegmentLength;
this.mode = defaults.mode;
this.outputSelection = defaults.outputSelection;
this.programDateTime = defaults.programDateTime;
this.programDateTimeClock = defaults.programDateTimeClock;
this.programDateTimePeriod = defaults.programDateTimePeriod;
this.redundantManifest = defaults.redundantManifest;
this.segmentLength = defaults.segmentLength;
this.segmentsPerSubdirectory = defaults.segmentsPerSubdirectory;
this.streamInfResolution = defaults.streamInfResolution;
this.timedMetadataId3Frame = defaults.timedMetadataId3Frame;
this.timedMetadataId3Period = defaults.timedMetadataId3Period;
this.timestampDeltaMilliseconds = defaults.timestampDeltaMilliseconds;
this.tsFileMode = defaults.tsFileMode;
}
@CustomType.Setter
public Builder adMarkers(@Nullable List adMarkers) {
this.adMarkers = adMarkers;
return this;
}
public Builder adMarkers(String... adMarkers) {
return adMarkers(List.of(adMarkers));
}
@CustomType.Setter
public Builder baseUrlContent(@Nullable String baseUrlContent) {
this.baseUrlContent = baseUrlContent;
return this;
}
@CustomType.Setter
public Builder baseUrlContent1(@Nullable String baseUrlContent1) {
this.baseUrlContent1 = baseUrlContent1;
return this;
}
@CustomType.Setter
public Builder baseUrlManifest(@Nullable String baseUrlManifest) {
this.baseUrlManifest = baseUrlManifest;
return this;
}
@CustomType.Setter
public Builder baseUrlManifest1(@Nullable String baseUrlManifest1) {
this.baseUrlManifest1 = baseUrlManifest1;
return this;
}
@CustomType.Setter
public Builder captionLanguageMappings(@Nullable List captionLanguageMappings) {
this.captionLanguageMappings = captionLanguageMappings;
return this;
}
public Builder captionLanguageMappings(ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsCaptionLanguageMapping... captionLanguageMappings) {
return captionLanguageMappings(List.of(captionLanguageMappings));
}
@CustomType.Setter
public Builder captionLanguageSetting(@Nullable String captionLanguageSetting) {
this.captionLanguageSetting = captionLanguageSetting;
return this;
}
@CustomType.Setter
public Builder clientCache(@Nullable String clientCache) {
this.clientCache = clientCache;
return this;
}
@CustomType.Setter
public Builder codecSpecification(@Nullable String codecSpecification) {
this.codecSpecification = codecSpecification;
return this;
}
@CustomType.Setter
public Builder constantIv(@Nullable String constantIv) {
this.constantIv = constantIv;
return this;
}
@CustomType.Setter
public Builder destination(ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsDestination destination) {
if (destination == null) {
throw new MissingRequiredPropertyException("ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettings", "destination");
}
this.destination = destination;
return this;
}
@CustomType.Setter
public Builder directoryStructure(@Nullable String directoryStructure) {
this.directoryStructure = directoryStructure;
return this;
}
@CustomType.Setter
public Builder discontinuityTags(@Nullable String discontinuityTags) {
this.discontinuityTags = discontinuityTags;
return this;
}
@CustomType.Setter
public Builder encryptionType(@Nullable String encryptionType) {
this.encryptionType = encryptionType;
return this;
}
@CustomType.Setter
public Builder hlsCdnSettings(@Nullable List hlsCdnSettings) {
this.hlsCdnSettings = hlsCdnSettings;
return this;
}
public Builder hlsCdnSettings(ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsHlsCdnSetting... hlsCdnSettings) {
return hlsCdnSettings(List.of(hlsCdnSettings));
}
@CustomType.Setter
public Builder hlsId3SegmentTagging(@Nullable String hlsId3SegmentTagging) {
this.hlsId3SegmentTagging = hlsId3SegmentTagging;
return this;
}
@CustomType.Setter
public Builder iframeOnlyPlaylists(@Nullable String iframeOnlyPlaylists) {
this.iframeOnlyPlaylists = iframeOnlyPlaylists;
return this;
}
@CustomType.Setter
public Builder incompleteSegmentBehavior(@Nullable String incompleteSegmentBehavior) {
this.incompleteSegmentBehavior = incompleteSegmentBehavior;
return this;
}
@CustomType.Setter
public Builder indexNSegments(@Nullable Integer indexNSegments) {
this.indexNSegments = indexNSegments;
return this;
}
@CustomType.Setter
public Builder inputLossAction(@Nullable String inputLossAction) {
this.inputLossAction = inputLossAction;
return this;
}
@CustomType.Setter
public Builder ivInManifest(@Nullable String ivInManifest) {
this.ivInManifest = ivInManifest;
return this;
}
@CustomType.Setter
public Builder ivSource(@Nullable String ivSource) {
this.ivSource = ivSource;
return this;
}
@CustomType.Setter
public Builder keepSegments(@Nullable Integer keepSegments) {
this.keepSegments = keepSegments;
return this;
}
@CustomType.Setter
public Builder keyFormat(@Nullable String keyFormat) {
this.keyFormat = keyFormat;
return this;
}
@CustomType.Setter
public Builder keyFormatVersions(@Nullable String keyFormatVersions) {
this.keyFormatVersions = keyFormatVersions;
return this;
}
@CustomType.Setter
public Builder keyProviderSettings(@Nullable ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsKeyProviderSettings keyProviderSettings) {
this.keyProviderSettings = keyProviderSettings;
return this;
}
@CustomType.Setter
public Builder manifestCompression(@Nullable String manifestCompression) {
this.manifestCompression = manifestCompression;
return this;
}
@CustomType.Setter
public Builder manifestDurationFormat(@Nullable String manifestDurationFormat) {
this.manifestDurationFormat = manifestDurationFormat;
return this;
}
@CustomType.Setter
public Builder minSegmentLength(@Nullable Integer minSegmentLength) {
this.minSegmentLength = minSegmentLength;
return this;
}
@CustomType.Setter
public Builder mode(@Nullable String mode) {
this.mode = mode;
return this;
}
@CustomType.Setter
public Builder outputSelection(@Nullable String outputSelection) {
this.outputSelection = outputSelection;
return this;
}
@CustomType.Setter
public Builder programDateTime(@Nullable String programDateTime) {
this.programDateTime = programDateTime;
return this;
}
@CustomType.Setter
public Builder programDateTimeClock(@Nullable String programDateTimeClock) {
this.programDateTimeClock = programDateTimeClock;
return this;
}
@CustomType.Setter
public Builder programDateTimePeriod(@Nullable Integer programDateTimePeriod) {
this.programDateTimePeriod = programDateTimePeriod;
return this;
}
@CustomType.Setter
public Builder redundantManifest(@Nullable String redundantManifest) {
this.redundantManifest = redundantManifest;
return this;
}
@CustomType.Setter
public Builder segmentLength(@Nullable Integer segmentLength) {
this.segmentLength = segmentLength;
return this;
}
@CustomType.Setter
public Builder segmentsPerSubdirectory(@Nullable Integer segmentsPerSubdirectory) {
this.segmentsPerSubdirectory = segmentsPerSubdirectory;
return this;
}
@CustomType.Setter
public Builder streamInfResolution(@Nullable String streamInfResolution) {
this.streamInfResolution = streamInfResolution;
return this;
}
@CustomType.Setter
public Builder timedMetadataId3Frame(@Nullable String timedMetadataId3Frame) {
this.timedMetadataId3Frame = timedMetadataId3Frame;
return this;
}
@CustomType.Setter
public Builder timedMetadataId3Period(@Nullable Integer timedMetadataId3Period) {
this.timedMetadataId3Period = timedMetadataId3Period;
return this;
}
@CustomType.Setter
public Builder timestampDeltaMilliseconds(@Nullable Integer timestampDeltaMilliseconds) {
this.timestampDeltaMilliseconds = timestampDeltaMilliseconds;
return this;
}
@CustomType.Setter
public Builder tsFileMode(@Nullable String tsFileMode) {
this.tsFileMode = tsFileMode;
return this;
}
public ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettings build() {
final var _resultValue = new ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettings();
_resultValue.adMarkers = adMarkers;
_resultValue.baseUrlContent = baseUrlContent;
_resultValue.baseUrlContent1 = baseUrlContent1;
_resultValue.baseUrlManifest = baseUrlManifest;
_resultValue.baseUrlManifest1 = baseUrlManifest1;
_resultValue.captionLanguageMappings = captionLanguageMappings;
_resultValue.captionLanguageSetting = captionLanguageSetting;
_resultValue.clientCache = clientCache;
_resultValue.codecSpecification = codecSpecification;
_resultValue.constantIv = constantIv;
_resultValue.destination = destination;
_resultValue.directoryStructure = directoryStructure;
_resultValue.discontinuityTags = discontinuityTags;
_resultValue.encryptionType = encryptionType;
_resultValue.hlsCdnSettings = hlsCdnSettings;
_resultValue.hlsId3SegmentTagging = hlsId3SegmentTagging;
_resultValue.iframeOnlyPlaylists = iframeOnlyPlaylists;
_resultValue.incompleteSegmentBehavior = incompleteSegmentBehavior;
_resultValue.indexNSegments = indexNSegments;
_resultValue.inputLossAction = inputLossAction;
_resultValue.ivInManifest = ivInManifest;
_resultValue.ivSource = ivSource;
_resultValue.keepSegments = keepSegments;
_resultValue.keyFormat = keyFormat;
_resultValue.keyFormatVersions = keyFormatVersions;
_resultValue.keyProviderSettings = keyProviderSettings;
_resultValue.manifestCompression = manifestCompression;
_resultValue.manifestDurationFormat = manifestDurationFormat;
_resultValue.minSegmentLength = minSegmentLength;
_resultValue.mode = mode;
_resultValue.outputSelection = outputSelection;
_resultValue.programDateTime = programDateTime;
_resultValue.programDateTimeClock = programDateTimeClock;
_resultValue.programDateTimePeriod = programDateTimePeriod;
_resultValue.redundantManifest = redundantManifest;
_resultValue.segmentLength = segmentLength;
_resultValue.segmentsPerSubdirectory = segmentsPerSubdirectory;
_resultValue.streamInfResolution = streamInfResolution;
_resultValue.timedMetadataId3Frame = timedMetadataId3Frame;
_resultValue.timedMetadataId3Period = timedMetadataId3Period;
_resultValue.timestampDeltaMilliseconds = timestampDeltaMilliseconds;
_resultValue.tsFileMode = tsFileMode;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy